1 回答
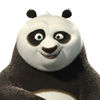
TA贡献1786条经验 获得超13个赞
我使用loop_start()andloop_stop()而不是loop_forever()然后使用 between start,stop我可以创建自己的循环来检查消息和打印文本。
我使用变量state来控制代码是在第一个hello( state = "start") 之前还是它得到hello,现在它必须检查时间并重复文本“好” ( state = "hello") 或者它有 20 秒之后hello并且它没有打印任何内容 (state = "other")
在内部on_message,我只hello在收到消息时才将状态更改为hello,而旧状态则不同hello。
import paho.mqtt.client as mqttClient
import time
# --- functions ---
def on_connect(client, userdata, flags, rc):
#global state
global connected
if rc == 0:
print("Connected to broker")
connected = True
else:
print("Connection failed")
def on_message(client, userdata, message):
global state
global last_processed
if message.payload.decode() == "hello":
if state != 'hello':
state = 'hello'
last_processed = time.time()
# OR
#if state != 'hello':
# if message.payload.decode() == "hello":
# state = 'hello'
# last_processed = time.time()
# --- main ---
broker_address = "192.168.1.111" # broker address
port = 1883 # broker port
user = "abcde" # connection username
password = "12345" # connection password
# ---
client = mqttClient.Client("Python") # create new instance
client.username_pw_set(user, password=password) # set username and password
client.on_connect= on_connect # attach function to callback
client.on_message= on_message # attach function to callback
client.connect(broker_address, port, 60) # connect
client.subscribe("home/OpenMQTTGateway/433toMQTT") # subscribe
# --- main loop ---
last_processed = None
connected = False # signal connection
state = 'start' # 'start', 'hello', 'other', 'not connected'
# ---
client.loop_start()
try:
while True:
if not connected:
print('not connected')
if state == 'start':
print('bad')
if state == 'hello':
if last_processed and time.time() - last_processed < 20:
print("good", time.time() - last_processed, end='\r') # '\r` to write it in the same line.
else:
print('bad')
state = 'other'
last_processed = None
if state == 'other':
pass
time.sleep(1.0) # to slow down example
except KeyboardInterrupt:
print('KeyboardInterrupt')
client.loop_stop()
编辑:
如果您只需要bad一次hello,那么您可以bad在循环之前先打印,然后hello在收到消息时打印,然后time.sleep(20)在打印bad和更改状态之前打印。
import paho.mqtt.client as mqttClient
import time
# --- functions ---
def on_connect(client, userdata, flags, rc):
#global state
global connected
if rc == 0:
print("Connected to broker")
connected = True
else:
print("Connection failed")
def on_message(client, userdata, message):
global state
message = message.payload.decode()
if state != 'hello':
if message == 'hello':
state = 'hello'
print('good') # once when start `hello`
else:
print('msg:', message)
# --- main ---
broker_address = "192.168.1.111" # broker address
port = 1883 # broker port
user = "abcde" # connection username
password = "12345" # connection password
# ---
client = mqttClient.Client("Python") # create new instance
client.username_pw_set(user, password=password) # set username and password
client.on_connect= on_connect # attach function to callback
client.on_message= on_message # attach function to callback
client.connect(broker_address, port, 60) # connect
client.subscribe("home/OpenMQTTGateway/433toMQTT") # subscribe
# --- main loop ---
connected = False # signal connection
state = 'other' # 'hello'
# ---
client.loop_start()
print('bad') # once at start
try:
while True:
if state == 'hello':
time.sleep(20)
print('bad') # once when end `hello`
state = 'other'
time.sleep(1.0) # to slow down example
except KeyboardInterrupt:
print('KeyboardInterrupt')
client.loop_stop()
使用threading.Timerinstead of time.sleep()because sleep()blocks loop 会很有用,如果你想做更多的事情,它就没那么有用了。
import paho.mqtt.client as mqttClient
import threading
# --- functions ---
def on_connect(client, userdata, flags, rc):
#global state
global connected
if rc == 0:
print("Connected to broker")
connected = True
else:
print("Connection failed")
def on_message(client, userdata, message):
global state
message = message.payload.decode()
if state != 'hello':
if message == 'hello':
state = 'hello'
print('good') # once when start `hello`
threading.Timer(20, end_hello).start()
else:
print('msg:', message)
def end_hello():
global state
print('bad') # once when end `hello`
state = 'other'
# --- main ---
broker_address = "192.168.1.111" # broker address
port = 1883 # broker port
user = "abcde" # connection username
password = "12345" # connection password
# ---
client = mqttClient.Client("Python") # create new instance
client.username_pw_set(user, password=password) # set username and password
client.on_connect= on_connect # attach function to callback
client.on_message= on_message # attach function to callback
client.connect(broker_address, port, 60) # connect
client.subscribe("home/OpenMQTTGateway/433toMQTT") # subscribe
# --- main loop ---
connected = False # signal connection
state = 'other' # 'hello'
# ---
print('bad') # once at start
client.loop_forever()
最终你仍然可以检查循环时间
import paho.mqtt.client as mqttClient
import time
# --- functions ---
def on_connect(client, userdata, flags, rc):
#global state
global connected
if rc == 0:
print("Connected to broker")
connected = True
else:
print("Connection failed")
def on_message(client, userdata, message):
global state
global last_processed
message = message.payload.decode()
if state != 'hello':
if message == 'hello':
state = 'hello'
last_processed = time.time()
print('good') # once when start `hello`
else:
print('msg:', message)
# --- main ---
broker_address = "192.168.1.111" # broker address
port = 1883 # broker port
user = "abcde" # connection username
password = "12345" # connection password
# ---
client = mqttClient.Client("Python") # create new instance
client.username_pw_set(user, password=password) # set username and password
client.on_connect= on_connect # attach function to callback
client.on_message= on_message # attach function to callback
client.connect(broker_address, port, 60) # connect
client.subscribe("home/OpenMQTTGateway/433toMQTT") # subscribe
# --- main loop ---
last_processed = None
connected = False # signal connection
state = 'other' # 'hello'
# ---
client.loop_start()
print('bad') # once at start
try:
while True:
if state == 'hello':
if time.time() >= last_processed + 20:
print('bad') # once when end `hello`
state = 'other'
time.sleep(1.0) # to slow down example
except KeyboardInterrupt:
print('KeyboardInterrupt')
client.loop_stop()
添加回答
举报