2 回答
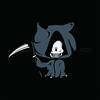
TA贡献1895条经验 获得超3个赞
这是后来者的最终解决方案。适用于 http/https:
package main
import (
"fmt"
"net"
"strings"
)
func main() {
fmt.Println("Start server...")
ln, _ := net.Listen("tcp", ":8000")
for {
conn, _ := ln.Accept()
handleSocket(conn)
}
}
func handleSocket(client_to_proxy net.Conn) {
buffer := make([]byte, 32*1024)
_, e := client_to_proxy.Read(buffer)
if e != nil {
fmt.Println("ERROR1 ", e)
return
}
message := string(buffer)
a := strings.Count(message, "\r\n")
fmt.Println(message)
fmt.Println(a)
if e != nil {
fmt.Println("ERROR1 ", e)
return
}
splited := strings.Split(message, " ")
//host := strings.Split(splited[1], ":")
if splited[0] == "CONNECT" {
//message = strings.Replace(message, "CONNECT", "GET", 1)
proxy_to_server, e := net.Dial("tcp", splited[1])
if e != nil {
fmt.Println("ERROR2 ", e)
return
}
//_, e = proxy_to_server.Write([]byte(message))
//if e != nil {
// fmt.Println("ERROR2 ", e)
// return
//}
lenn, e := client_to_proxy.Write([]byte("HTTP/1.1 200 OK\r\n\r\n"))
if e != nil {
fmt.Println("ERROR8 ", e)
return
}
fmt.Println(lenn)
read443(client_to_proxy, proxy_to_server)
} else if splited[0] == "GET" {
host1 := strings.Replace(splited[1], "http://", "", 1)
host2 := host1[:len(host1)-1]
var final_host string
if strings.LastIndexAny(host2, "/") > 0 {
final_host = host2[:strings.LastIndexAny(host2, "/")]
} else {
final_host = host2
}
proxy_to_server, e := net.Dial("tcp", final_host+":80")
if e != nil {
fmt.Println("ERROR7 ", e)
return
}
_, e = proxy_to_server.Write([]byte(message))
if e != nil {
fmt.Println("ERROR6 ", e)
return
}
write80(client_to_proxy, proxy_to_server)
}
}
func write80(client_to_proxy net.Conn, proxy_to_server net.Conn) {
buffer := make([]byte, 64*1024)
readLeng, err := proxy_to_server.Read(buffer)
if err != nil {
fmt.Println("ERROR9 ", err)
return
}
fmt.Println("WRIIIIIIIIIIIIIIIIIIIIIIT from server:")
fmt.Println(string(buffer[:readLeng]))
if readLeng > 0 {
_, err := client_to_proxy.Write(buffer[:readLeng])
if err != nil {
fmt.Println("ERR4 ", err)
return
}
}
go read80(client_to_proxy, proxy_to_server)
for {
readLeng, err := proxy_to_server.Read(buffer)
if err != nil {
fmt.Println("ERROR10 ", err)
return
}
fmt.Println("WRIIIIIIIIIIIIIIIIIIIIIIT from server:")
fmt.Println(string(buffer[:readLeng]))
if readLeng > 0 {
_, err := client_to_proxy.Write(buffer[:readLeng])
if err != nil {
fmt.Println("ERR4 ", err)
return
}
}
}
}
func read80(client_to_proxy net.Conn, proxy_to_server net.Conn) {
buffer := make([]byte, 32*1024)
for {
readLeng, err := client_to_proxy.Read(buffer)
if err != nil {
return
}
fmt.Println("REEEEEEEEEEEEEEEEEEEEEEED from client:")
fmt.Println(string(buffer[:readLeng]))
if readLeng > 0 {
_, err := proxy_to_server.Write(buffer[:readLeng])
if err != nil {
fmt.Println("ERR5 ", err)
return
}
}
}
}
func write443(client_to_proxy net.Conn, proxy_to_server net.Conn) {
buffer := make([]byte, 32*1024)
for {
readLeng, err := proxy_to_server.Read(buffer)
if err != nil {
fmt.Println("ERROR10 ", err)
return
}
fmt.Println("WRIIIIIIIIIIIIIIIIIIIIIIT from server:")
fmt.Println(string(buffer[:readLeng]))
if readLeng > 0 {
_, err := client_to_proxy.Write(buffer[:readLeng])
if err != nil {
fmt.Println("ERR4 ", err)
return
}
}
}
}
func read443(client_to_proxy net.Conn, proxy_to_server net.Conn) {
buffer := make([]byte, 32*1024)
readLeng, err := client_to_proxy.Read(buffer)
if err != nil {
return
}
fmt.Println("REEEEEEEEEEEEEEEEEEEEEEED from client:")
fmt.Println(string(buffer[:readLeng]))
if readLeng > 0 {
_, err := proxy_to_server.Write(buffer[:readLeng])
if err != nil {
fmt.Println("ERR5 ", err)
return
}
}
go write443(client_to_proxy, proxy_to_server)
for {
readLeng, err := client_to_proxy.Read(buffer)
if err != nil {
return
}
fmt.Println("REEEEEEEEEEEEEEEEEEEEEEED from client:")
fmt.Println(string(buffer[:readLeng]))
if readLeng > 0 {
_, err := proxy_to_server.Write(buffer[:readLeng])
if err != nil {
fmt.Println("ERR5 ", err)
return
}
}
}
}
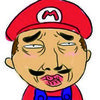
TA贡献1851条经验 获得超5个赞
与所有 HTTP 请求一样,CONNECT 请求以多行请求标头开头,该标头以仅包含 \r\n 的行结尾。但你只读了它的第一行:
message, e := bufio.NewReader(client_to_proxy).ReadString('\n')
请求的其余部分被发送到服务器,这可能会返回一些错误,因为这不是 TLS 握手的预期开始。TLS 握手的开始仅出现在请求标头之后。服务器返回的错误然后被转发到客户端并被解释为 TLS 消息——这被视为损坏的消息,因此是“坏 mac”。
readAll(client_to_proxy, proxy_to_server)
无需将所有剩余数据(即除了请求的第一行之外的所有内容)转发到服务器,您需要首先读取完整的请求标头,然后才在客户端和服务器之间转发所有内容。
- 2 回答
- 0 关注
- 288 浏览
添加回答
举报