3 回答
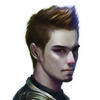
TA贡献1777条经验 获得超3个赞
有两种方法可以做到这一点,具体取决于您想要的行为。在这两种情况下,我们都想获取标题元素及其位置:
var headerDiv = document.querySelector("header");
var headerBottom = headerDiv.offsetTop + headerDiv.offsetHeight;
这意味着在滚动中,我们可以检查我们是否已经传递了标题:
if (window.pageYOffset >= headerBottom)
1:标题正常滚动到视图之外,向上滚动时固定在顶部
在此版本中,向下滚动时标题会滚出视图,但当您开始向上滚动时,它会固定在屏幕顶部。
完整的工作示例:
var prevScrollpos = window.pageYOffset;
/* Get the header element and it's position */
var headerDiv = document.querySelector("header");
var headerBottom = headerDiv.offsetTop + headerDiv.offsetHeight;
window.onscroll = function() {
var currentScrollPos = window.pageYOffset;
/* if scrolling down, let it scroll out of view as normal */
if (prevScrollpos <= currentScrollPos ){
headerDiv.classList.remove("fixedToTop");
headerDiv.style.top ="-7.2rem";
}
/* otherwise if we're scrolling up, fix the nav to the top */
else{
headerDiv.classList.add("fixedToTop");
headerDiv.style.top = "0";
}
prevScrollpos = currentScrollPos;
}
body{ margin: 0;}
header {
background: blue;
height: 7.2rem;
transition: all 0.2s ease-in-out;
z-index: 100;
}
.content {
height:1000px;
}
header.fixedToTop {
position: fixed;
top: 0;
left: 0;
right: 0;
}
/* add space for fixed header when it's fixed to top */
header.fixedToTop + .content{
margin-top:8rem;
}
<header></header>
<div class="content" id="contentdiv">
<h1>My Page</h1>
<p>Content 1</p><p>Content 2</p><p>Content 3</p><p>Content 4</p><p>Content 5</p>
<p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p>
<p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p>
</div>
这是如何运作的
我们需要从标题中删除固定定位并将其添加到fixedToTop我们将应用于滚动的类(例如):
header {
height: 7.2rem;
transition: all 0.2s ease-in-out;
z-index: 100;
}
header.fixedToTop {
position: fixed;
top: 0; left: 0; right: 0;
}
现在滚动函数的逻辑非常简单:
如果我们向下滚动,则让标题滚动到视图之外 - 删除类
fixedToTop
。如果我们向上滚动,添加我们的
fixedToTop
类,这将使它出现
请注意,我们需要显式设置值才能top
使过渡动画起作用,因此我们也在代码中这样做。
将它们放在一起,我们得到以下滚动函数:
window.onscroll = function() {
var currentScrollPos = window.pageYOffset;
/* if scrolling down, let it scroll out of view as normal */
if (prevScrollpos <= currentScrollPos ){
headerDiv.classList.remove("fixedToTop");
headerDiv.style.top ="-7.2rem";
}
/* otherwise if we're scrolling up, fix the nav to the top */
else{
headerDiv.classList.add("fixedToTop");
headerDiv.style.top = "0";
}
prevScrollpos = currentScrollPos;
}
2:标题是固定的,直到我们滚动到它通常会消失的地方;向上滚动时固定在顶部
在这种情况下,标题是固定的,直到我们滚动通过它会消失的“自然”位置。
工作示例:
var prevScrollpos = window.pageYOffset;
/* Get the header element and it's position */
var headerDiv = document.querySelector("header");
var headerBottom = headerDiv.offsetTop + headerDiv.offsetHeight;
window.onscroll = function() {
var currentScrollPos = window.pageYOffset;
/* if we're scrolling up, or we haven't passed the header,
show the header at the top */
if (prevScrollpos > currentScrollPos || currentScrollPos < headerBottom){
headerDiv.style.top = "0";
}
else{
/* otherwise we're scrolling down & have passed the header so hide it */
headerDiv.style.top = "-7.2rem";
}
prevScrollpos = currentScrollPos;
}
header {
background: blue;
height: 7.2rem;
position: fixed;
top: 0;
left: 0;
right: 0;
transition: top 0.2s ease-in-out;
z-index: 100;
}
.content {
height:1000px;
margin-top:8rem; /* add space for fixed header */
}
<header></header>
<div class="content" id="contentdiv">
<h1>My Page</h1>
<p>Content 1</p><p>Content 2</p><p>Content 3</p><p>Content 4</p><p>Content 5</p>
<p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p>
<p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p><p>Content</p>
</div>
这是如何运作的
滚动功能中的逻辑是这样的:
如果我们向上滚动,则显示标题:
如果我们向下滚动...
...如果我们滚动通过标题,将其隐藏
...否则显示它
将它们放在一起,我们得到以下滚动函数:
var prevScrollpos = window.pageYOffset; // save the current position
window.onscroll = function() {
var currentScrollPos = window.pageYOffset;
/* if we're scrolling up, or we haven't passed the header, show the header */
if (prevScrollpos > currentScrollPos || currentScrollPos < headerBottom){
headerDiv.style.top = "0";
}
else{
/* otherwise we're scrolling down & have passed the header so hide it */
headerDiv.style.top = "-7.2rem";
}
prevScrollpos = currentScrollPos;
}
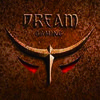
TA贡献1829条经验 获得超7个赞
我使用了 FluffyKitten 提供的第一个答案,效果很好。谢谢。我会在那里发表评论,但我缺乏这样做的声誉。
为了帮助其他人,在实现 FluffyKitten 的第一个答案时,我了解到标题和 .content 的垂直对齐方式在 (a) 加载或刷新(页面上还没有滚动,所以不是 fixedToTop)与(b)滚动后的 fixedToTop 之间略有不同,因为只有主体边距设置为 0。
我不仅将 body 元素边距设置为 0(就像 FluffyKitten 所做的那样),而且还必须将页眉和 .content 元素的顶部边距设置为 0,以防止在我的网页首次加载或刷新时出现轻微的垂直布局差异(当标题不固定到顶部时)与向上滚动后标题固定到顶部时的对比。
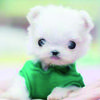
TA贡献1865条经验 获得超7个赞
也许您可以将滚动位置与元素的高度进行比较:
var prevScrollpos = window.pageYOffset;
window.onscroll = function() {
var currentScrollPos = window.pageYOffset;
if (prevScrollpos > currentScrollPos) {
document.querySelector("header").style.top = "0";
} else if (currentScrollPos > document.querySelector("header").offsetHeight) {
document.querySelector("header").style.top = "-7.2rem";
}
prevScrollpos = currentScrollPos;
}
header {
background-color: rgb(255, 255, 255);
height: 7.2rem;
position: fixed;
top: 0;
left: 0;
right: 0;
transition: top 0.2s ease-in-out;
z-index: 100;
}
添加回答
举报