4 回答
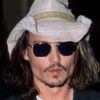
TA贡献1784条经验 获得超9个赞
您可以使用Array.reduce来获得所需的结果,使用数组作为累加器。
我们枚举过期数组中的每个对象,如果它不存在于输出数组中,我们将其添加,否则我们将对象目标添加到输出中的相关元素。
const overdue = [ { "user.employeeId": "10001440", "objectives": [ "Understand the financial indexes." ] }, { "user.employeeId": "10000303", "objectives": [ "Document preparation & writing skills" ] }, { "user.employeeId": "10002168", "objectives": [ "Chia ratio setting for Fuze Tea products L11" ] }, { "user.employeeId": "10002168", "objectives": [ "Brix parameter differences between Processing and Production of Fuze Tea Lemon-Lemongrass standardization" ] }, { "user.employeeId": "10002168", "objectives": [ "Paramix Line 9 setting parameter standardization" ] }, ];
let result = overdue.reduce((res, row) => {
let el = res.find(el => el["user.employeeId"] === row["user.employeeId"]);
// If we find the object in the output array simply update the objectives
if (el) {
el.objectives = [...el.objectives, ...row.objectives];
} else {
// If we _don't_ find the object, add to the output array.
res.push({ ...row});
}
return res;
}, [])
console.log("Result:",result);
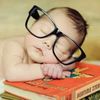
TA贡献1850条经验 获得超11个赞
使用array.reduce对象并将其传递给累加器。在回调函数中检查累加器是否具有与 的值相同的键user.employeeId。如果不是,则创建键并将迭代中的当前对象添加为它的值。如果已经有一个键,那么只需更新目标数组。在检索值时Object.valuewhich 将给出一个数组
const overdue = [{
"user.employeeId": "10001440",
"objectives": [
"Understand the financial indexes."
]
},
{
"user.employeeId": "10000303",
"objectives": [
"Document preparation & writing skills"
]
},
{
"user.employeeId": "10002168",
"objectives": [
"Chia ratio setting for Fuze Tea products L11"
]
},
{
"user.employeeId": "10002168",
"objectives": [
"Brix parameter differences between Processing and Production of Fuze Tea Lemon-Lemongrass standardization"
]
},
{
"user.employeeId": "10002168",
"objectives": [
"Paramix Line 9 setting parameter standardization"
]
},
];
let newData = overdue.reduce((acc, curr) => {
if (!acc[curr['user.employeeId']]) {
acc[curr['user.employeeId']] = curr;
} else {
curr.objectives.forEach((item) => {
acc[curr['user.employeeId']]['objectives'].push(item)
})
}
return acc;
}, {});
console.log(Object.values(newData))
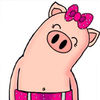
TA贡献2039条经验 获得超7个赞
我不确定如何在 lodash 中执行此操作,但这是我仅使用 vanilla Javascript 执行此操作的方法:
const overdue = [{
"user.employeeId": "10001440",
"objectives": [
"Understand the financial indexes."
]
},
{
"user.employeeId": "10000303",
"objectives": [
"Document preparation & writing skills"
]
},
{
"user.employeeId": "10002168",
"objectives": [
"Chia ratio setting for Fuze Tea products L11"
]
},
{
"user.employeeId": "10002168",
"objectives": [
"Brix parameter differences between Processing and Production of Fuze Tea Lemon-Lemongrass standardization"
]
},
{
"user.employeeId": "10002168",
"objectives": [
"Paramix Line 9 setting parameter standardization"
]
},
];
const combined = {};
overdue.forEach(o => {
const id = o["user.employeeId"];
const obj = o["objectives"][0];
if(!combined[id]) { combined[id] = [obj]; }
else { combined[id].push(obj); }
});
const result = [];
Object.keys(combined).forEach(key => {
result.push({"user.employeeId" : key, "objectives" : combined[key]});
});
console.log(result);
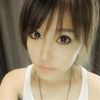
TA贡献1813条经验 获得超2个赞
如果你可以使用 lodash,那么你就可以超越 reduce 并使用更具体的东西来满足你的需求。在这种情况下,您可以考虑使用该_.groupBy
方法,该方法将创建一个由user.employeeId
值作为键的对象,其中包含值作为每个对象的数组。然后,您可以将对象的值(即:具有相同 employeeId 的对象数组)映射到每个数组合并的合并对象objectives
。最后,您可以获取分组对象的值以获得结果:
const overdue = [{ "user.employeeId": "10001440", "objectives": [ "Understand the financial indexes." ] }, { "user.employeeId": "10000303", "objectives": [ "Document preparation & writing skills" ] }, { "user.employeeId": "10002168", "objectives": [ "Chia ratio setting for Fuze Tea products L11" ] }, { "user.employeeId": "10002168", "objectives": [ "Brix parameter differences between Processing and Production of Fuze Tea Lemon-Lemongrass standardization" ] }, { "user.employeeId": "10002168", "objectives": [ "Paramix Line 9 setting parameter standardization" ] }, ];
const group = _.flow(
arr => _.groupBy(arr, "user.employeeId"),
g => _.mapValues(g, arr => _.mergeWith(...arr, (objV, srcV) => {
if (_.isArray(objV)) return objV.concat(srcV);
})),
_.values
);
console.log(group(overdue));
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.15/lodash.min.js"></script>
或者您可以将 vanilla JS 与.reduce()
a 一起使用Map
,这使用了 JS 的更多现代功能(节点 14.0.0 均支持),例如可选链接 ?.
和空合并运算符 ??
:
const overdue = [{ "user.employeeId": "10001440", "objectives": [ "Understand the financial indexes." ] }, { "user.employeeId": "10000303", "objectives": [ "Document preparation & writing skills" ] }, { "user.employeeId": "10002168", "objectives": [ "Chia ratio setting for Fuze Tea products L11" ] }, { "user.employeeId": "10002168", "objectives": [ "Brix parameter differences between Processing and Production of Fuze Tea Lemon-Lemongrass standardization" ] }, { "user.employeeId": "10002168", "objectives": [ "Paramix Line 9 setting parameter standardization" ] }, ];
const group = (arr, key) => Array.from(arr.reduce((m, obj) =>
m.set(
obj[key],
{...obj, objectives: [...(m.get(obj[key])?.objectives ?? []), ...obj.objectives]}
), new Map).values()
);
console.log(group(overdue, "user.employeeId"));
添加回答
举报