3 回答
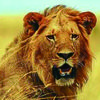
TA贡献1803条经验 获得超3个赞
另一种解决方案是使用指令来实现行为。
import {
AfterViewInit,
Component,
ComponentFactory,
ComponentFactoryResolver,
ContentChild,
Directive,
Injector,
Input,
Optional,
SkipSelf,
TemplateRef,
ViewContainerRef,
} from '@angular/core';
import { MatFormFieldControl } from '@angular/material/form-field';
@Directive({
selector: '[appClearable]'
})
export class ClearableDirective implements AfterViewInit {
@ContentChild(MatFormFieldControl) matInput: MatFormFieldControl<any>;
@Input() appClearable: TemplateRef<any>;
private factory: ComponentFactory<ClearButtonComponent>;
constructor(
private vcr: ViewContainerRef,
resolver: ComponentFactoryResolver,
private injector: Injector,
) {
this.factory = resolver.resolveComponentFactory(ClearButtonComponent);
}
ngAfterViewInit(): void {
if (this.appClearable) {
this.vcr.createEmbeddedView(this.appClearable);
} else {
this.vcr.createComponent(this.factory, undefined, this.injector);
}
}
/**
* This is used to clear the formControl oder HTMLInputElement
*/
clear(): void {
if (this.matInput.ngControl) {
this.matInput.ngControl.control.reset();
} else {
this.matInput.value = '';
}
}
}
/**
* This is the markup/component for the clear-button that is shown to the user.
*/
@Component({
selector: 'app-clear-button',
template: `
<button (click)="clearHost()">Clear</button>
`
})
export class ClearButtonComponent {
constructor(@Optional() @SkipSelf() private clearDirective: ClearableDirective) { }
clearHost(): void {
if (this.clearDirective) {
this.clearDirective.clear();
}
}
}
这会appClearable为后备布局创建一个名为的指令和一个可选的组件。确保将组件和指令添加到declarations模块的 -array 中。您可以指定用于提供用户界面的模板,也可以将其ClearButtonComponent用作通用的解决方案。标记如下所示:
<!-- Use it with a template reference -->
<mat-form-field [appClearable]="clearableTmpl">
<input type="text" matInput [formControl]="exampleInput">
</mat-form-field>
<!-- use it without a template reference -->
<mat-form-field appClearable>
<input type="text" matInput [formControl]="exampleInput2">
</mat-form-field>
<ng-template #clearableTmpl>
<button (click)="exampleInput.reset()">Marked-Up reference template</button>
</ng-template>
无论是否使用 ngControl/FormControl,这都适用,但您可能需要根据您的用例对其进行调整。
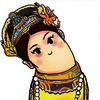
TA贡献1842条经验 获得超12个赞
从 2022 年的 Angular 14 开始,MatFormField 的问题已经针对angular/angular#37319关闭,没有解决方案。如果您需要它工作,以下似乎是现在可以使用<ng-content>
with的最佳解决方案mat-form-field
:
@Component({
selector: 'my-input-wrapper',
template: `
<mat-form-field appearance="standard">
<ng-content></ng-content>
<!-- make sure this is destroyed so all bindings/subscriptions are removed-->
<input *ngIf="isBeforeViewInit$$ | async" hidden matInput />
</mat-form-field>
`,
});
class MyInputWrapper implement AfterViewInit {
isBeforeViewInit$$ = new BehaviorSubject(true);
@ContentChild(MatFormFieldControl) matFormControl: MatFormFieldControl<unknown>;
@ViewChild(MatFormField) matFormField: MatFormField;
ngAfterViewInit() {
// replace the reference to the dummy control
this.matFormField._control = this.matFormControl;
// force the form field to rebind everything to the actual control
this.matFormField.ngAfterContentInit();
this.isBeforeViewInit$$.next(false);
}
}
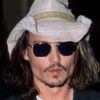
TA贡献1784条经验 获得超9个赞
更新:
选项 1 不适用于新的角度版本,因为在钩子@ViewChild()中返回未定义。ngOnInit()另一个技巧是使用假人MatFormFieldControl-
选项 2
<mat-form-field>
<input matInput hidden>
<ng-content></ng-content>
</mat-form-field>
编辑:
抛出该错误是因为MatFormField组件查询使用的子内容@ContentChild(MatFormFieldControl)如果您使用嵌套则不起作用ng-content(MatFormField 也使用内容投影)。
选项 1(已弃用)
以下是如何让它工作 -
@Component({
selector: 'mat-clearable-input',
template: `
<mat-form-field>
<ng-content></ng-content>
</mat-form-field>
`
})
export class FieldComponent implements OnInit {
@ContentChild(MatFormFieldControl) _control: MatFormFieldControl<any>;
@ViewChild(MatFormField) _matFormField: MatFormField;
ngOnInit() {
this._matFormField._control = this._control;
}
}
请检查这个 stackBlitz。此外,已经创建了这个问题github
。
添加回答
举报