3 回答
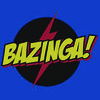
TA贡献1789条经验 获得超8个赞
(我希望这终于到了重点......)
此代码返回范围与滑块选择的价格范围重叠(完全或部分)的所有价格段。
/*
* NOTES: price segments should not overlap
*/
const priceSegments = ['20-50', '51-100']; // originally was ['20-50', '50-100'] see note
const sliderRange = [1, 50];
const pricesList = [{
value: 30.5,
count: 2
},
{
value: 27.7,
count: 1
},
{
value: 50.0,
count: 2
},
{
value: 55.5,
count: 2
}
];
// if you want an array of strings (e.g. ["20-50", "51-100"])
const priceSegmentsMatchingRange_arrayOfStrings = priceSegments.filter(segment => {
const [segmentMin, segmentMax] = segment.split('-');
const [rangeMin, rangeMax] = sliderRange;
return (
(parseInt(segmentMin) < parseInt(rangeMin) && parseInt(segmentMax) >= parseInt(rangeMin)) ||
(parseInt(segmentMin) >= parseInt(rangeMin) && parseInt(segmentMax) <= parseInt(rangeMax)) ||
(parseInt(segmentMin) <= parseInt(rangeMax) && parseInt(segmentMax) > parseInt(rangeMax))
);
});
console.log(priceSegmentsMatchingRange_arrayOfStrings);
// if you want an array of arrays of strings (e.g. [["20", "50"], ["51", "100"]])
const priceSegmentsMatchingRange_arrayArraysOfStrings = priceSegments.filter(segment => {
const [segmentMin, segmentMax] = segment.split('-');
const [rangeMin, rangeMax] = sliderRange;
return (
(parseInt(segmentMin) < parseInt(rangeMin) && parseInt(segmentMax) >= parseInt(rangeMin)) ||
(parseInt(segmentMin) >= parseInt(rangeMin) && parseInt(segmentMax) <= parseInt(rangeMax)) ||
(parseInt(segmentMin) <= parseInt(rangeMax) && parseInt(segmentMax) > parseInt(rangeMax))
);
}).map(segment => segment.split('-'));
console.log(priceSegmentsMatchingRange_arrayArraysOfStrings);
// if you want an array of arrays of numbers (e.g. [[20, 50], [51, 100]])
const priceSegmentsMatchingRange_arrayArraysOfNumbers = priceSegments.filter(segment => {
const [segmentMin, segmentMax] = segment.split('-');
const [rangeMin, rangeMax] = sliderRange;
return (
(parseInt(segmentMin) < parseInt(rangeMin) && parseInt(segmentMax) >= parseInt(rangeMin)) ||
(parseInt(segmentMin) >= parseInt(rangeMin) && parseInt(segmentMax) <= parseInt(rangeMax)) ||
(parseInt(segmentMin) <= parseInt(rangeMax) && parseInt(segmentMax) > parseInt(rangeMax))
);
}).map(segment => segment.split('-').map(item => parseInt(item)));
console.log(priceSegmentsMatchingRange_arrayArraysOfNumbers);
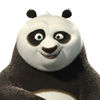
TA贡献2012条经验 获得超12个赞
你可以使用array.filter
例如:
allValues = [
{ val: 5, count: 2},
{ val: 6, count: 2},
{ val: 7, count: 2},
{ val: 8, count: 2},
{ val: 9, count: 2},
{ val: 10, count: 3}
];
var sliderStartVal = 6;
var sliderEndVal = 9;
console.log(allValues.filter(x => x.val > sliderStartVal && x.val < sliderEndVal));
// Gives [{"val":7,"count":2},{"val":8,"count":2}]
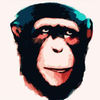
TA贡献1790条经验 获得超9个赞
如果我正确理解你想要实现的目标,你只需要使用Array.prototype.filter()(https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter)。
[编辑] 我仍然不是 100% 确定我得到了你想要实现的目标,但据我所知,这个编辑过的片段可能是你需要的。
/*
* NOTES:
* I think there are a couple of logical error I eliminated in this code snippet
* 1. price segments should not overlap
* 2. it should not be possible to select a price lower/higher than the lowes/highest price segment
*/
const priceSegments = ['20-50', '51-100']; // originally was ['20-50', '50-100'] see note (1.)
const sliderRange = [20, 50]; // originally was [1, 50] - see note (2.)
const pricesList = [{
value: 30.5,
count: 2
},
{
value: 27.7,
count: 1
},
{
value: 50.0,
count: 2
},
{
value: 55.5,
count: 2
}
];
const priceRange = priceSegments.reduce((outObj, segment) => {
/*
* if the slider minimum falls within the segment,
* set the minPrice to the segment minumum
*/
if (
parseInt(segment.split('-')[0]) <= parseInt(sliderRange[0]) &&
parseInt(segment.split('-')[1]) >= parseInt(sliderRange[0])
) {
outObj.minPrice = parseInt(segment.split('-')[0]);
}
/*
* if the slider maximum falls within the segment,
* set the maxPrice to the segment maximum
*/
if (
parseInt(segment.split('-')[0]) <= parseInt(sliderRange[1]) &&
parseInt(segment.split('-')[1]) >= parseInt(sliderRange[1])
) {
outObj.maxPrice = parseInt(segment.split('-')[1]);
}
return outObj;
}, {
minPrice: 0,
maxPrice: 0
});
const {
minPrice,
maxPrice
} = priceRange;
const pricesInRange = pricesList.filter((price) => price.value >= minPrice && price.value <= maxPrice);
console.log(pricesInRange);
添加回答
举报