4 回答
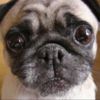
TA贡献2065条经验 获得超14个赞
使用 Object.entires()andisArray检查它是否是一个数组
obj = {
name: "jane",
age: 22,
interested_books: [
{ book_name: "xxx", author_name: "yyy" },
{ book_name: "aaa", author_name: "zzz" },
],
hobbies: ["reading", "football"],
address: { street_name: "dace street", door_no: 12 },
};
function getArrWithOb(obj) {
return Object.fromEntries(
Object.entries(obj).filter((o) => {
if (Array.isArray(o[1])) {
if (o[1].every((ob) => typeof ob == "object")) {
return o;
}
}
})
);
}
console.log(getArrWithOb(obj));
从评论看来你想要返回的是你可以使用的属性的名称reduce
obj = { name: "jane", age: 22, interested_books: [ { book_name: "xxx", author_name: "yyy" }, { book_name: "aaa", author_name: "zzz" }, ], hobbies: ["reading", "football"], address: { street_name: "dace street", door_no: 12 }, };
function getArrWithOb(obj) {
return Object.entries(obj).reduce((r, o) => {
if (Array.isArray(o[1])) {
if (o[1].every((ob) => typeof ob == "object")) {
r.push(o[0]);
}
}
return r;
}, []);
}
console.log(getArrWithOb(obj));
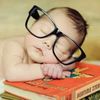
TA贡献1757条经验 获得超7个赞
使用isArray检查值是否为数组
const obj = {
name: 'jane',
age: 22,
interested_books: [{
book_name: 'xxx',
author_name: 'yyy'
}, {
book_name: 'aaa',
author_name: 'zzz'
}],
hobbies: ['reading', 'football'],
address: {
street_name: 'dace street',
door_no: 12
}
}
for (let keys in obj) {
if (Array.isArray(obj[keys])) {
obj[keys].forEach(item => console.log(item))
}
}
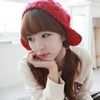
TA贡献1876条经验 获得超7个赞
const obj = {
name: 'jane',
age: 22,
interested_books: [{
book_name: 'xxx',
author_name: 'yyy'
}, {
book_name: 'aaa',
author_name: 'zzz'
}],
hobbies: ['reading', 'football'],
address: {
street_name: 'dace street',
door_no: 12
}
}
for (const key in obj) {
if (obj[key] instanceof Array) {
console.log('I am array', key);
const isArrayOfObject = obj[key].every(item => typeof item === "object");
if (isArrayOfObject) {
// your_logic_here
}
}
}
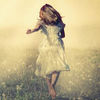
TA贡献1712条经验 获得超3个赞
试试下面的功能。这是为了获取对象中的数组项。
const obj = {
name: 'jane',
age: 22,
interested_books: [{ book_name: 'xxx', author_name: 'yyy' }, { book_name: 'aaa', author_name: 'zzz' }],
hobbies: ['reading', 'football'],
address: { street_name: 'dace street', door_no: 12 }
};
const getArraysFromObject = obj => {
const results = {};
Object.keys(obj).map(field => {
if (Array.isArray(obj[field])) {
results[field] = obj[field];
}
});
return results;
};
console.log(results);
// {
// "interested_books": [
// { "book_name": "xxx", "author_name": "yyy" },
// { "book_name": "aaa", "author_name": "zzz" }
// ],
// "hobbies": ["reading", "football"]
// }
添加回答
举报