2 回答
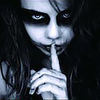
TA贡献1840条经验 获得超5个赞
package main
import (
"fmt"
"sort"
)
func main() {
people := []struct {
Name string
Age int
}{
{"Gopher", 7},
{"Alice", 55},
{"Vera", 24},
{"Bob", 75},
}
sort.Slice(people, func(i, j int) bool { return people[i].Name < people[j].Name })
fmt.Println("By name:", people)
sort.Slice(people, func(i, j int) bool { return people[i].Age < people[j].Age })
fmt.Println("By age:", people)
}
如果将匿名函数分解为两个单独的函数(例如,comparePeopleByName(i, j int)和comparePeopleByAge(i, j, int)),您将如何测试它们?
将函数绑定到闭包上似乎是个奇怪的想法。
注意:我认为测试sort.Slice()自己是一种不好的形式;它随语言一起提供,不应该(需要?)进行测试。
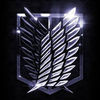
TA贡献1860条经验 获得超8个赞
胡思乱想后,我写了这段代码。它使用工厂来创建方法,以便切片包含在函数的闭包中。
我把它放在一个要点中以便于玩弄:https ://gist.github.com/docwhat/e3b13265d24471651e02f7d7a42e7d2c
// main.go
package main
import (
"fmt"
"sort"
)
type Person struct {
Name string
Age int
}
func comparePeopleByName(people []Person) func(int, int) bool {
return func(i, j int) bool {
return people[i].Name < people[j].Name
}
}
func comparePeopleByAge(people []Person) func(int, int) bool {
return func(i, j int) bool {
return people[i].Age < people[j].Age
}
}
func main() {
people := []Person{
{"Gopher", 7},
{"Alice", 55},
{"Vera", 24},
{"Bob", 75},
}
sort.Slice(people, comparePeopleByName(people))
fmt.Println("By name:", people)
sort.Slice(people, comparePeopleByAge(people))
fmt.Println("By age:", people)
}
// main_test.go
package main
import "testing"
func TestComparePeopleByName(t *testing.T) {
testCases := []struct {
desc string
a, b Person
expected bool
}{
{"a < b", Person{"bob", 1}, Person{"krabs", 2}, true},
{"a > b", Person{"krabs", 1}, Person{"bob", 2}, false},
{"a = a", Person{"plankton", 1}, Person{"plankton", 2}, false},
}
for _, testCase := range testCases {
t.Run(testCase.desc, func(t *testing.T) {
people := []Person{testCase.a, testCase.b}
got := comparePeopleByName(people)(0, 1)
if testCase.expected != got {
t.Errorf("expected %v, got %v", testCase.expected, got)
}
})
}
}
func TestComparePeopleByAge(t *testing.T) {
testCases := []struct {
desc string
a, b Person
expected bool
}{
{"a < b", Person{"sandy", 10}, Person{"patrick", 20}, true},
{"a > b", Person{"sandy", 30}, Person{"patrick", 20}, false},
{"a = b", Person{"sandy", 90}, Person{"patrick", 90}, false},
}
for _, testCase := range testCases {
t.Run(testCase.desc, func(t *testing.T) {
people := []Person{testCase.a, testCase.b}
got := comparePeopleByAge(people)(0, 1)
if testCase.expected != got {
t.Errorf("expected %v, got %v", testCase.expected, got)
}
})
}
}
- 2 回答
- 0 关注
- 95 浏览
添加回答
举报