2 回答
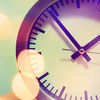
TA贡献1848条经验 获得超2个赞
以下都是调用API的C#例子
33.读取ini文件属性
//using System.Runtime.InteropServices;
//[DllImport("kernel32")]//返回取得字符串缓冲区的长度
//private static extern long GetPrivateProfileString(string section,string key, string def,StringBuilder retVal,int size,string filePath);
string Section=%%1;
string Key=%%2;
string NoText=%%3;
string iniFilePath="Setup.ini";
string %%4=String.Empty;
if(File.Exists(iniFilePath)){
StringBuilder temp = new StringBuilder(1024);
GetPrivateProfileString(Section,Key,NoText,temp,1024,iniFilePath);
%%4=temp.ToString();
}
35.写入ini文件属性
//using System.Runtime.InteropServices;
//[DllImport("kernel32")]//返回0表示失败,非0为成功
//private static extern long WritePrivateProfileString(string section,string key, string val,string filePath);
string Section=%%1;
string Key=%%2;
string Value=%%3;
string iniFilePath="Setup.ini";
bool %%4=false;
if(File.Exists(iniFilePath))
{
long OpStation = WritePrivateProfileString(Section,Key,Value,iniFilePath);
if(OpStation == 0)
{
%%4=false;
}
else
{
%%4=true;
}
}
83.注册全局热键
注册全局热键要用到Windows的API方法RegisterHotKey和UnregisterHotKey。
一、声明注册热键方法 [DllImport("user32.dll")]
private static extern int RegisterHotKey(IntPtr hwnd, int id, int fsModifiers, int vk);
[DllImport("user32.dll")]
private static extern int UnregisterHotKey(IntPtr hwnd, int id);
int Space = 32; //热键ID
private const int WM_HOTKEY = 0x312; //窗口消息-热键
private const int WM_CREATE = 0x1; //窗口消息-创建
private const int WM_DESTROY = 0x2; //窗口消息-销毁
private const int MOD_ALT = 0x1; //ALT
private const int MOD_CONTROL = 0x2; //CTRL
private const int MOD_SHIFT = 0x4; //SHIFT
private const int VK_SPACE = 0x20; //SPACE
二、注册热键方法 /// <summary>
/// 注册热键
/// </summary>
/// <param name="hwnd">窗口句柄</param>
/// <param name="hotKey_id">热键ID</param>
/// <param name="fsModifiers">组合键</param>
/// <param name="vk">热键</param>
private void RegKey(IntPtr hwnd, int hotKey_id, int fsModifiers, int vk)
{
bool result;
if (RegisterHotKey(hwnd,hotKey_id,fsModifiers,vk) == 0)
{
result = false;
}
else
{
result = true;
}
if (!result)
{
MessageBox.Show("注册热键失败!");
}
}
/// <summary>
/// 注销热键
/// </summary>
/// <param name="hwnd">窗口句柄</param>
/// <param name="hotKey_id">热键ID</param>
private void UnRegKey(IntPtr hwnd, int hotKey_id)
{
UnregisterHotKey(hwnd,hotKey_id);
}
三、重写WndProc方法,实现注册 protected override void WndProc(ref Message m)
{
base.WndProc(ref m);
switch(m.Msg)
{
case WM_HOTKEY: //窗口消息-热键
switch(m.WParam.ToInt32())
{
case 32: //热键ID
MessageBox.Show("Hot Key : Ctrl + Alt + Shift + Space");
break;
default:
break;
}
break;
case WM_CREATE: //窗口消息-创建
RegKey(Handle,Space,MOD_ALT | MOD_CONTROL | MOD_SHIFT,VK_SPACE); //注册热键
break;
case WM_DESTROY: //窗口消息-销毁
UnRegKey(Handle,Space); //销毁热键
break;
default:
break;
}
}
84.菜单勾选/取消完成后关闭计算机
/*
using System.Runtime.InteropServices;
[StructLayout(LayoutKind.Sequential, Pack = 1)]
internal struct TokPriv1Luid
{
public int Count;
public long Luid;
public int Attr;
}
[DllImport("kernel32.dll", ExactSpelling = true)]
internal static extern IntPtr GetCurrentProcess();
[DllImport("advapi32.dll", ExactSpelling = true, SetLastError = true)]
internal static extern bool OpenProcessToken(IntPtr h, int acc, ref IntPtr phtok);
[DllImport("advapi32.dll", SetLastError = true)]
internal static extern bool LookupPrivilegeValue(string host, string name, ref long pluid);
[DllImport("advapi32.dll", ExactSpelling = true, SetLastError = true)]
internal static extern bool AdjustTokenPrivileges(IntPtr htok, bool disall, ref TokPriv1Luid newst, int len, IntPtr prev, IntPtr relen);
[DllImport("user32.dll", ExactSpelling = true, SetLastError = true)]
internal static extern bool ExitWindowsEx(int flg, int rea);
internal const int SE_PRIVILEGE_ENABLED = 0x00000002;
internal const int TOKEN_QUERY = 0x00000008;
internal const int TOKEN_ADJUST_PRIVILEGES = 0x00000020;
internal const string SE_SHUTDOWN_NAME = "SeShutdownPrivilege";
internal const int EWX_SHUTDOWN = 0x00000001;
internal const int EWX_POWEROFF = 0x00000008;
internal const int EWX_FORCE = 0x00000004;
internal const int EWX_FORCEIFHUNG = 0x00000010;
*/
int flg=EWX_FORCE | EWX_POWEROFF;
bool ok;
TokPriv1Luid tp;
IntPtr hproc = GetCurrentProcess();
IntPtr htok = IntPtr.Zero;
ok = OpenProcessToken(hproc, TOKEN_ADJUST_PRIVILEGES | TOKEN_QUERY, ref htok);
tp.Count = 1;
tp.Luid = 0;
tp.Attr = SE_PRIVILEGE_ENABLED;
ok = LookupPrivilegeValue(null, SE_SHUTDOWN_NAME, ref tp.Luid);
ok = AdjustTokenPrivileges(htok, false, ref tp, 0, IntPtr.Zero, IntPtr.Zero);
ok = ExitWindowsEx(flg, 0);
85.菜单勾选/取消完成后重新启动计算机
/*
using System.Runtime.InteropServices;
[StructLayout(LayoutKind.Sequential, Pack = 1)]
internal struct TokPriv1Luid
{
public int Count;
public long Luid;
public int Attr;
}
[DllImport("kernel32.dll", ExactSpelling = true)]
internal static extern IntPtr GetCurrentProcess();
[DllImport("advapi32.dll", ExactSpelling = true, SetLastError = true)]
internal static extern bool OpenProcessToken(IntPtr h, int acc, ref IntPtr phtok);
[DllImport("advapi32.dll", SetLastError = true)]
internal static extern bool LookupPrivilegeValue(string host, string name, ref long pluid);
[DllImport("advapi32.dll", ExactSpelling = true, SetLastError = true)]
internal static extern bool AdjustTokenPrivileges(IntPtr htok, bool disall, ref TokPriv1Luid newst, int len, IntPtr prev, IntPtr relen);
[DllImport("user32.dll", ExactSpelling = true, SetLastError = true)]
internal static extern bool ExitWindowsEx(int flg, int rea);
internal const int SE_PRIVILEGE_ENABLED = 0x00000002;
internal const int TOKEN_QUERY = 0x00000008;
internal const int TOKEN_ADJUST_PRIVILEGES = 0x00000020;
internal const string SE_SHUTDOWN_NAME = "SeShutdownPrivilege";
internal const int EWX_SHUTDOWN = 0x00000001;
internal const int EWX_REBOOT = 0x00000002;
internal const int EWX_FORCE = 0x00000004;
internal const int EWX_FORCEIFHUNG = 0x00000010;
*/
int flg=EWX_FORCE | EWX_REBOOT;
bool ok;
TokPriv1Luid tp;
IntPtr hproc = GetCurrentProcess();
IntPtr htok = IntPtr.Zero;
ok = OpenProcessToken(hproc, TOKEN_ADJUST_PRIVILEGES | TOKEN_QUERY, ref htok);
tp.Count = 1;
tp.Luid = 0;
tp.Attr = SE_PRIVILEGE_ENABLED;
ok = LookupPrivilegeValue(null, SE_SHUTDOWN_NAME, ref tp.Luid);
ok = AdjustTokenPrivileges(htok, false, ref tp, 0, IntPtr.Zero, IntPtr.Zero);
ok = ExitWindowsEx(flg, 0);
86.菜单勾选/取消完成后注销计算机
/*
using System.Runtime.InteropServices;
[StructLayout(LayoutKind.Sequential, Pack = 1)]
internal struct TokPriv1Luid
{
public int Count;
public long Luid;
public int Attr;
}
[DllImport("kernel32.dll", ExactSpelling = true)]
internal static extern IntPtr GetCurrentProcess();
[DllImport("advapi32.dll", ExactSpelling = true, SetLastError = true)]
internal static extern bool OpenProcessToken(IntPtr h, int acc, ref IntPtr phtok);
[DllImport("advapi32.dll", SetLastError = true)]
internal static extern bool LookupPrivilegeValue(string host, string name, ref long pluid);
[DllImport("advapi32.dll", ExactSpelling = true, SetLastError = true)]
internal static extern bool AdjustTokenPrivileges(IntPtr htok, bool disall, ref TokPriv1Luid newst, int len, IntPtr prev, IntPtr relen);
[DllImport("user32.dll", ExactSpelling = true, SetLastError = true)]
internal static extern bool ExitWindowsEx(int flg, int rea);
internal const int SE_PRIVILEGE_ENABLED = 0x00000002;
internal const int TOKEN_QUERY = 0x00000008;
internal const int TOKEN_ADJUST_PRIVILEGES = 0x00000020;
internal const string SE_SHUTDOWN_NAME = "SeShutdownPrivilege";
internal const int EWX_SHUTDOWN = 0x00000001;
internal const int EWX_LOGOFF = 0x00000000;
internal const int EWX_FORCE = 0x00000004;
internal const int EWX_FORCEIFHUNG = 0x00000010;
*/
int flg=EWX_FORCE | EWX_LOGOFF;
bool ok;
TokPriv1Luid tp;
IntPtr hproc = GetCurrentProcess();
IntPtr htok = IntPtr.Zero;
ok = OpenProcessToken(hproc, TOKEN_ADJUST_PRIVILEGES | TOKEN_QUERY, ref htok);
tp.Count = 1;
tp.Luid = 0;
tp.Attr = SE_PRIVILEGE_ENABLED;
ok = LookupPrivilegeValue(null, SE_SHUTDOWN_NAME, ref tp.Luid);
ok = AdjustTokenPrivileges(htok, false, ref tp, 0, IntPtr.Zero, IntPtr.Zero);
ok = ExitWindowsEx(flg, 0);
87.菜单勾选/取消开机自启动程序
public void RunWhenStart(bool Started)
{
string name=%%1;
string path=Application.ExecutablePath;
RegistryKey HKLM = Registry.LocalMachine;
RegistryKey Run = HKLM.CreateSubKey(@"SOFTWARE\Microsoft\Windows\CurrentVersion\Run\");
if (Started == true)
{
try
{
Run.SetValue(name, path);
HKLM.Close();
}
catch (Exception)
{
MessageBox.Show("注册表修改错误(开机自启未实现)");
}
}
else
{
try
{
if (Run.GetValue(name) != null)
{
Run.DeleteValue(name);
HKLM.Close();
}
else
return;
}
catch (Exception e)
{
//ExceptionTransact.WriteErrLog(base.GetType().Name, e.Message);
MessageBox(e.Message);
}
}
}
89.模拟键盘输入字符串
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using KenZhang.Free.VirtualInput;
using System.Runtime.InteropServices;
namespace VirtualInputDemo
{
public partial class Form1 : Form
{
public const int INPUT_KEYBOARD = 1;
public const int KEYEVENTF_KEYUP = 0x0002;
[DllImport("user32.dll")]
public static extern UInt32 SendInput(UInt32 nInputs, ref INPUT pInputs, int cbSize);
[StructLayout(LayoutKind.Explicit)]
public struct INPUT
{
[FieldOffset(0)]
public Int32 type;
[FieldOffset(4)]
public KEYBDINPUT ki;
[FieldOffset(4)]
public MOUSEINPUT mi;
[FieldOffset(4)]
public HARDWAREINPUT hi;
}
[StructLayout(LayoutKind.Sequential)]
public struct MOUSEINPUT
{
public Int32 dx;
public Int32 dy;
public Int32 mouseData;
public Int32 dwFlags;
public Int32 time;
public IntPtr dwExtraInfo;
}
[StructLayout(LayoutKind.Sequential)]
public struct KEYBDINPUT
{
public Int16 wVk;
public Int16 wScan;
public Int32 dwFlags;
public Int32 time;
public IntPtr dwExtraInfo;
}
[StructLayout(LayoutKind.Sequential)]
public struct HARDWAREINPUT
{
public Int32 uMsg;
public Int16 wParamL;
public Int16 wParamH;
}
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
textBox1.Focus();
INPUT inDown = new INPUT();
inDown.type = INPUT_KEYBOARD;
inDown.ki.wVk = (int)Keys.A;
//INPUT inUp = new INPUT();
//inUp.type = INPUT_KEYBOARD;
//inUp.ki.wVk = (int)Keys.A;
//inUp.ki.dwFlags = KEYEVENTF_KEYUP;
SendInput(1, ref inDown, Marshal.SizeOf(inDown));
//SendInput(1, ref inUp, Marshal.SizeOf(inUp));
}
}
}
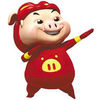
TA贡献2080条经验 获得超4个赞
如果查看所有进程的DLL文件,运行CMD:
tasklist /m
运行后显示所有进程加载的DLL文件.
假如我们查看进程iexplore.exe调用或加载的模块文件,运行:
tasklist /m iexplore.exe
运行后显示所有该应用程序的DLL文件.
查看DLL:
一个很简单的方法就是用.NET自带的命令行工具
1.首先启动vs的命令行工具
2.定位到你的DLL的那个目录
3.然后输入" ildasm a.dll;注意:a.dll是你的dll的名字 就可以了
不知你问的是不是这个
希望能帮上你
- 2 回答
- 0 关注
- 63 浏览
添加回答
举报