3 回答
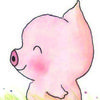
TA贡献1900条经验 获得超5个赞
使用这个我希望它能解决你的问题。 如果您在遵循代码方面需要任何帮助,请告诉我。
import React, {Component} from 'react';
import {View, TouchableOpacity, Text, TextInput} from 'react-native';
export class AddInputs extends Component {
state = {
textInput: [],
inputData: [],
};
//function to add TextInput dynamically
addTextInput = index => {
let textInput = this.state.textInput;
textInput.push(
<TextInput
style={{
height: 40,
width: '100%',
borderColor: '#2B90D8',
borderBottomWidth: 3,
}}
placeholder={'Add Text'}
onChangeText={text => this.addValues(text, index)}
/>,
);
this.setState({textInput});
};
//function to add text from TextInputs into single array
addValues = (text, index) => {
let dataArray = this.state.inputData;
let checkBool = false;
if (dataArray.length !== 0) {
dataArray.forEach(value => {
if (value.index === index) {
value.text = text;
checkBool = true;
}
});
}
if (checkBool) {
this.setState({
inputData: dataArray,
});
} else {
dataArray.push({text: text, index: index});
this.setState({
inputData: dataArray,
});
}
};
render() {
return (
<View
style={{
flex: 1,
}}>
<View
style={{
width: '100%',
alignItems: 'center',
}}>
{this.state.textInput.map(value => {
return value;
})}
</View>
<TouchableOpacity
onPress={() => {
this.addTextInput(
'your desired number of inputs here like 5, 20 etc',
);
}}>
<Text>Add Inputs</Text>
</TouchableOpacity>
</View>
);
}
}
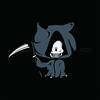
TA贡献1848条经验 获得超10个赞
你可以这样做:
this.state = { values: [] };
const textInputs = [];
for(let i=0; i< this.state.noOfStores; i++) {
textInputs.push(
<TextInput
style ={[styles.input,{ backgroundColor: theme.colors.lightGray }]}
placeholder=" Name "
keyboardType={'default'}
placeholderTextColor="gray"
onChangeText={text => this.onChangeText(text,i)}
/>
)
this.setState({ values: [...this.state.values, ''] });
}
onChangeText = (text,index) => {
const values = [...this.state.values];
values[index] = text;
this.setState({ values });
}
然后在渲染方法中:
<View>
{textInputs}
</View>
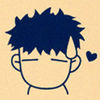
TA贡献1796条经验 获得超4个赞
你可以像下面这样,根据你的选择器设置一个给定长度的数组,并显示基于它的 UI。
setRows 设置行数,您可以轻松更新数组。
从您的选择器中调用 setRows。
export default function App() {
const [textArr, setTextArr] = useState([]);
const onChangeText = (text, index) => {
const arr = [...textArr];
arr[index] = text;
setTextArr(arr);
};
const setRows=(rowCount)=>{
setTextArr(Array(rowCount).fill('Default text'))
};
return (
<View style={{ paddingTop: 100 }}>
<Button
title="set"
onPress={()=>setRows(6)}
/>
{textArr.map((item, index) => (
<TextInput
style={[styles.input, { backgroundColor: 'grey' }]}
placeholder=" Name "
keyboardType={'default'}
placeholderTextColor="gray"
value={item}
onChangeText={(text)=>onChangeText(text,index)}
/>
))}
</View>
);
}
添加回答
举报