3 回答
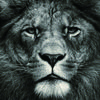
TA贡献1869条经验 获得超4个赞
你可以使用 .json() 方法:
const fetched = await fetch("/url", {
method: 'GET',
});
const fetchedJson: object = await fetched.json();
console.log(fetchedJson)
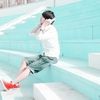
TA贡献1966条经验 获得超4个赞
有几种方法可以做到这一点。
使用承诺
获取响应对象本身包含一些方法,可以帮助您获得不同形式的响应,例如.json()、.text()和.status。点击此处了解详情。所以,如果你只是想将答案解析成一个 JSON 对象,你可以这样做
function doSomethingOnParsedJson(res) {
// Do something on the response here...
}
function readOperacions() {
fetch("http://localhost:8080/operaciones", {
method: "GET",
})
.then(res => res.json())
.then(doSomethingOnParsedJson) // Pass in a function without parentheses
.catch(console.error);
}
如果你定义一个单独的函数来执行你想对解析的响应做的工作并将函数(不带括号)传递给它会更干净,then但你也可以继续并直接给它一个函数,如:
function readOperacions() {
fetch("http://localhost:8080/operaciones", {
method: "GET",
})
.then(res => res.json())
.then(parsedResponse => {
// do something...
})
.catch(console.error);
}
使用异步/等待
您还可以使用异步/等待功能来实现这一点。
function doSomethingOnParsedJson(res) {
// Do something on the response here...
}
async function readOperacions() {
try {
// Get the response from the server.
const res = await fetch("http://localhost:8080/operaciones", {
method: "GET",
});
// Parse it into a JSON object.
const parsedJson = res.json();
// Do something on it.
doSomethingOnParsedJson(parsedJson);
} catch (error) {
// Show an error if something unexpected happened.
}
}
边注
在 Express 中有一种更简洁的方法来发送 JSON 响应。您可以.json在 Express 响应对象上使用该方法。
app.get("/operaciones", async (req, res) => {
const rows = await readAll();
/* Don't do this!
res.setHeader("content-type", "application/json")
res.send(JSON.stringify(rows))
*/
/* Do this instead ;-) */
res.json(rows);
})
瞧!就这么简单。
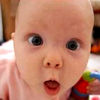
TA贡献1752条经验 获得超4个赞
将 .then 添加到获取链并打印结果:
fetch('http://example.com/movies.json')
.then(response => {
console.log('response: ' + JSON.stringify(response));
})
...
...
https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Using_Fetch
添加回答
举报