3 回答
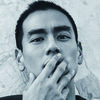
TA贡献1796条经验 获得超7个赞
好吧,简单的方法是这样的:
// Raw data
const raw = `HEADER1|HEADER2|HEADER3
Value1|Value2|Value3
Value4|Value5|Value6
Value7|Value8|Value9`;
// Or load raw data from file
const fs = require('fs');
const raw = (fs.readFileSync('file.txt')).toString();
// Split data by lines
const data = raw.split('\n');
// Extract array of headers and
// cut it from data
const headers = (data.shift()).split('|');
// Define target JSON array
let json = [];
// Loop data
for(let i = 0; i < data.length; i++) {
// Remove empty lines
if(/^\s*$/.test(data[i])) continue;
// Split data line on cells
const contentCells = data[i].split('|');
// Loop cells
let jsonLine = {};
for(let i = 0; i < contentCells.length; i++) jsonLine[headers[i]] = contentCells[i];
// Push new line to json array
json.push(jsonLine);
}
// Result
console.log(json);
例子在这里
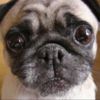
TA贡献1735条经验 获得超5个赞
一个名为readline的核心模块将是最好的选择
const fs = require('fs');
const readline = require('readline');
async function processLineByLine() {
const fileStream = fs.createReadStream('input.txt');
const rl = readline.createInterface({
input: fileStream,
crlfDelay: Infinity
});
// Note: we use the crlfDelay option to recognize all instances of CR LF
// ('\r\n') in input.txt as a single line break.
const finalResult = [];
let keys = [];
let i = 0;
for await (const line of rl) {
//Extract first line as keys
if(i === 0) keys = line.split('|');
else {
const lineArray = line.split('|');
const result = keys.reduce((o, k, i) => ({...o, [k]: lineArray[i]}), {});
// Or try
// result = Object.assign(...keys.map((k, i) => ({[k]: lineArray[i]})));
// Or
// let result = {};
// keys.forEach((key, i) => result[key] = lineArray[i]);
finalResult.push(result);
console.log(result);
}
i++;
}
return finalResult;
}
processLineByLine();
添加回答
举报