2 回答
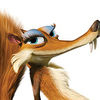
TA贡献1946条经验 获得超4个赞
顺便说一句,已经有一个用于排序的库
https://pkg.go.dev/golang.org/x/exp/slices#Sort
1. 您可以使用泛型创建接口,然后为其键入断言。
例子:
type Lesser[T SupportedType] interface {
Less(T) bool
}
type Vec[T SupportedType] []T
func (vec Vec[T]) Less(a, b int) bool {
return any(vec[a]).(Lesser[T]).Less(vec[b])
}
func main() {
vs := Vec[String]([]String{"a", "b", "c", "d", "e"})
vb := Vec[Bool]([]Bool{false, true})
fmt.Println(vs.Less(3, 1))
fmt.Println(vb.Less(0, 1))
}
2.可以保存Vec上的类型。
例子:
type Lesser[T SupportedType] interface {
Less(T) bool
}
type Vec[T SupportedType, L Lesser[T]] []T
func (vec Vec[T, L]) Less(a, b int) bool {
return any(vec[a]).(L).Less(vec[b])
}
func main() {
vs := Vec[String, String]([]String{"a", "b", "c", "d", "e"})
fmt.Println(vs.Less(3, 1))
}
3.嵌套类型约束
谢谢@blackgreen
例子 :
type SupportedType interface {
Int8 | Time | Bool | String
}
type Lesser[T SupportedType] interface {
Less(T) bool
}
type Vec[T interface {
SupportedType
Lesser[T]
}] []T
func (vec Vec[T]) Less(a, b int) bool {
return vec[a].Less(vec[b])
}
func main() {
vs := Vec[String]([]String{"a", "b", "c", "d", "e"})
fmt.Println(vs.Less(3, 1))
}
基准:
benchmark 1 : 28093368 36.52 ns/op 16 B/op 1 allocs/op
benchmark 2 : 164784321 7.231 ns/op 0 B/op 0 allocs/op
benchmark 3 : 212480662 5.733 ns/op 0 B/op 0 allocs/op
Embedding a container inside type specific structs:
benchmark 4 : 211429621 5.720 ns/op 0 B/op 0 allocs/op
哪一个最适合您取决于您。但 IMO 3 号是最好的。
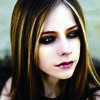
TA贡献2051条经验 获得超10个赞
就我个人而言,我认为最好不要在联合中包含许多彼此无关的类型,因为它们不会共享许多通用操作,并且您最终会编写特定于类型的代码。那么使用泛型的意义何在……?
无论如何,可能的策略取决于SupportedType约束类型集中包含的内容,以及您希望对这些内容执行的操作:
只有确切的类型,没有方法
使用类型开关T并运行任何对具体类型有意义的操作。当方法实现仅使用 type 的一个值时,这种方法效果最好T,因为您可以直接使用 switch guard ( v := any(vec[a]).(type)) 中的变量。当您T在 switch guard 中的值旁边有更多值时,它就不再漂亮了,因为您必须单独转换和断言所有这些值:
func (vec Vec[T]) Less(a, b int) bool {
switch v := any(vec[a]).(type) {
case int64:
return v < any(vec[b]).(int64)
case time.Time:
return v.Before(any(vec[b]).(time.Time))
// more cases...
}
return false
}
用方法
参数化包含方法的接口并将其约束T为支持的类型。然后将Vector类型参数约束为两者。这个的优点是确保Vector不能使用您忘记实现Less(T) bool的类型实例化并摆脱类型断言,否则可能会在运行时出现恐慌。
type Lesser[T SupportedType] interface {
Less(T) bool
}
type Vec[T interface { SupportedType; Lesser[T] }] []T
func (vec Vec[T]) Less(a, b int) bool {
return vec[a].Less(vec[b])
}
使用方法和预先声明的类型
不可能的。考虑以下:
type SupportedTypes interface {
// exact predeclared types
int | string
}
type Lesser[T SupportedTypes] interface {
Less(T) bool
}
约束Lesser有一个空类型集,因为既int不能也string不能有方法。所以在这里你回到了“精确类型和无方法”的情况。
具有近似类型 ( ~T)
将上面的约束条件改成近似类型:
type SupportedTypes interface {
// approximate types
~int | ~string
}
type Lesser[T SupportedTypes] interface {
Less(T) bool
}
类型开关不是一个选项,因为case ~int:它不合法。约束上存在的方法会阻止您使用预先声明的类型进行实例化:
Vector[MyInt8]{} // ok when MyInt8 implements Lesser
Vector[int8] // doesn't compile, int8 can't implement Lesser
所以我看到的选项是:
强制客户端代码使用定义的类型,在许多情况下这可能很好
将约束的范围缩小到支持相同操作的类型
反射(用于查看性能损失是否对您来说太多的基准),但反射实际上无法找到基础类型,因此您只能使用reflect.Kindor进行一些黑客攻击CanConvert。
当/如果该提案通过时,这可能会改善并可能胜过其他选项。
- 2 回答
- 0 关注
- 85 浏览
添加回答
举报