3 回答
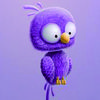
TA贡献1796条经验 获得超4个赞
也许您最好的选择是弄清楚如何将其转化为形式的线性方程组的形式c[1]x[1] + c[2]x[2] … + c[n]x[n] = 0
。从那里,您可以使用广泛建立的线性系统技术来求解系统。有关大量信息,请参阅Wikipedia页面。您可以让用户以这种形式为您的方法提供输入,或者您可以对每个方程进行少量处理以对其进行转换(例如,如果所有方程在 LHS 上都有一个变量,如您的示例所示,则翻转签名并将其放在 RHS 的末尾)。
解释求解线性方程组的理论超出了这个答案的范围,但基本上,如果有一个有效的分配,你的系统要么是唯一确定的,如果不存在有效的分配是过度确定的,或者如果有无限多的分配是可能的. 如果有一个独特的任务,你会得到数字;如果系统未确定,您至少会得到一组约束,这些约束必须包含无限多个解决方案中的任何一个;如果不确定,您将一无所获并且知道原因。
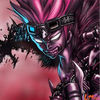
TA贡献1803条经验 获得超6个赞
这就是我解决这个问题的方法。首先,我通过将所有变量放在左侧并将值 & 0 放在右侧来创建方程:
CTC = 1000000
(0.4)CTC - Basic = 0
(0.5)Basic-HRA = 0
ConvAll = 10000
(0.12)Basic-PF = 0
(0.0481)Basic - Gratuity = 0
CTC - (Basic + HRA + ConvAll + PF+ Gratuity + OtherAll) = 0
然后我创建了一个这样的矩阵:
|1 0 0 0 0 0 0| |CTC | = |1000000|
|0.4 -1 0 0 0 0 0| |Basic | = |0 |
|0 0.5 -1 0 0 0 0| |HRA | = |0
|0 0 0 1 0 0 0| |ConvAll | = |10000 |
|0 0.12 0 0 -1 0 0| |PF | = |0 |
|0 0.0481 0 0 0 -1 0| |Gratuity| = |10000 |
|1 -1 -1 -1 -1 -1 -1| |OtherAll| = |0 |
在此之后,我计算了(上面第一个矩阵的逆)和(最右边的矩阵)的乘积,并使用下面的代码得到了每个分量的相应值:
public class Matrix
{
static int n = 0;
public static void main(String argv[]) {
Scanner input = new Scanner(System.in);
System.out.println("Enter the dimension of square matrix: ");
n = input.nextInt();
double a[][] = new double[n][n];
System.out.println("Enter the elements of matrix: ");
for (int i = 0; i < n; i++)
for (int j = 0; j < n; j++)
a[i][j] = input.nextDouble();
double d[][] = invert(a);
System.out.println();
System.out.println("Enter the equation values: ");
System.out.println();
double b[][] = new double[n][1];
for (int i = 0; i < n; i++) {
b[i][0] = input.nextDouble();
}
double e[][] = multiplyMatrix(d, b);
System.out.println();
System.out.println("The final solution is: ");
System.out.println();
for (int i = 0; i < n; i++) {
for (int j = 0; j < 1; j++) {
System.out.printf(e[i][j] + " ");
}
System.out.println();
}
input.close();
}
public static double[][] invert(double a[][]) {
int n = a.length;
double x[][] = new double[n][n];
double b[][] = new double[n][n];
int index[] = new int[n];
for (int i = 0; i < n; ++i)
b[i][i] = 1;
// Transform the matrix into an upper triangle
gaussian(a, index);
// Update the matrix b[i][j] with the ratios stored
for (int i = 0; i < n - 1; ++i)
for (int j = i + 1; j < n; ++j)
for (int k = 0; k < n; ++k)
b[index[j]][k] -= a[index[j]][i] * b[index[i]][k];
// Perform backward substitutions
for (int i = 0; i < n; ++i) {
x[n - 1][i] = b[index[n - 1]][i] / a[index[n - 1]][n - 1];
for (int j = n - 2; j >= 0; --j) {
x[j][i] = b[index[j]][i];
for (int k = j + 1; k < n; ++k) {
x[j][i] -= a[index[j]][k] * x[k][i];
}
x[j][i] /= a[index[j]][j];
}
}
return x;
}
// Method to carry out the partial-pivoting Gaussian
// elimination. Here index[] stores pivoting order.
public static void gaussian(double a[][], int index[]) {
int n = index.length;
double c[] = new double[n];
// Initialize the index
for (int i = 0; i < n; ++i)
index[i] = i;
// Find the rescaling factors, one from each row
for (int i = 0; i < n; ++i) {
double c1 = 0;
for (int j = 0; j < n; ++j) {
double c0 = Math.abs(a[i][j]);
if (c0 > c1)
c1 = c0;
}
c[i] = c1;
}
// Search the pivoting element from each column
int k = 0;
for (int j = 0; j < n - 1; ++j) {
double pi1 = 0;
for (int i = j; i < n; ++i) {
double pi0 = Math.abs(a[index[i]][j]);
pi0 /= c[index[i]];
if (pi0 > pi1) {
pi1 = pi0;
k = i;
}
}
// Interchange rows according to the pivoting order
int itmp = index[j];
index[j] = index[k];
index[k] = itmp;
for (int i = j + 1; i < n; ++i) {
double pj = a[index[i]][j] / a[index[j]][j];
// Record pivoting ratios below the diagonal
a[index[i]][j] = pj;
// Modify other elements accordingly
for (int l = j + 1; l < n; ++l)
a[index[i]][l] -= pj * a[index[j]][l];
}
}
}
public static double[][] multiplyMatrix(double a[][], double b[][]) {
double c[][] = new double[n][1];
for (int i = 0; i < n; i++) {
for (int j = 0; j < 1; j++) {
for (int k = 0; k < n; k++) {
c[i][j] = c[i][j] + a[i][k] * b[k][j];
}
}
}
return c;
}
}
谢谢大家的线索。
添加回答
举报