2 回答
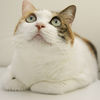
TA贡献1921条经验 获得超9个赞
这是您可以使用的一种方法Promise.all:
const fetchExternalData = () => {
return Promise.all([
fetch("file1.txt"),
fetch("file2.txt")
])
.then(
results => Promise.all(
results.map(result => result.text())
)
)
}
然后,在调用您的fetchExternalData函数时,您将获得一个包含两个文件中数据的项目数组:
fetchExternalData().then(
(response) => {
// [file1data, file2data]
}
)
这是一个例子:
const fetchExternalData = () => {
return Promise.all([
fetch("https://jsonplaceholder.typicode.com/todos/1"),
fetch("https://jsonplaceholder.typicode.com/todos/2")
]).then(results => {
return Promise.all(results.map(result => result.json()));
});
};
fetchExternalData()
.then(result => {
// console.log(result);
})
.catch(console.error);
或者,如果您想返回 anobject而不是 an array,您可以执行以下操作:
const fetchExternalData = items => {
return Promise.all(
items.map(item =>
fetch(`https://jsonplaceholder.typicode.com/todos/${item.id}`)
)
)
.then(
responses => Promise.all(
responses.map(response => response.json())
)
)
// use `Array.reduce` to map your responses to appropriate keys
.then(results =>
results.reduce((acc, result, idx) => {
const key = items[idx].key;
// use destructing assignment to add
// a new key/value pair to the final object
return {
...acc,
[key]: result
};
}, {})
);
};
fetchExternalData([
{ id: 1, key: "todo1" },
{ id: 2, key: "todo2" }
])
.then(result => {
console.log("result", result);
console.log('todo1', result["todo1"]);
})
.catch(console.error);
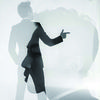
TA贡献1884条经验 获得超4个赞
通过将其放入对象中返回多个值。像这样:
const fetchExternalData = async() => {
const resp1 = await fetch('file1.txt');
const resp2 = await fetch('file2.txt');
return ({res1: resp1.text(), res2: resp2.text()});
}
添加回答
举报