3 回答
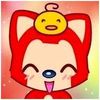
TA贡献1858条经验 获得超8个赞
如何在 Go 中使用递归(无循环)找到整数的总和?
程序应该询问每个“输入数量”的“数字数量”和“数字”,答案是这些数字的平方和。
这是问题的答案,Go 中的递归解决方案:
$ go run sumsq.go
Enter the number of inputs:
2
Enter the number of numbers:
2
Enter the numbers:
1
2
Enter the number of numbers:
2
Enter the numbers:
2
3
Sum of Squares:
5
13
$
package main
import (
"bufio"
"fmt"
"os"
"strconv"
"strings"
)
func readInt(r *bufio.Reader) int {
line, err := r.ReadString('\n')
line = strings.TrimSpace(line)
if err != nil {
if len(line) == 0 {
return 0
}
}
i, err := strconv.Atoi(line)
if err != nil {
return 0
}
return i
}
func nSquares(n int, r *bufio.Reader) int {
if n == 0 {
return 0
}
i := readInt(r)
return i*i + nSquares(n-1, r)
}
func nNumbers(n int, r *bufio.Reader, sums *[]int) int {
if n == 0 {
return 0
}
fmt.Println("\nEnter the number of numbers: ")
i := readInt(r)
fmt.Println("Enter the numbers: ")
*sums = append(*sums, nSquares(i, r))
return nNumbers(n-1, r, sums)
}
func nInputs(r *bufio.Reader) []int {
fmt.Println("Enter the number of inputs: ")
i := readInt(r)
sums := make([]int, 0, i)
nNumbers(i, r, &sums)
return sums
}
func sumSqrs(sums []int) {
if len(sums) == 0 {
return
}
fmt.Println(sums[0])
sumSqrs(sums[1:])
}
func main() {
r := bufio.NewReader(os.Stdin)
fmt.Println()
sums := nInputs(r)
fmt.Println("\nSum of Squares:")
sumSqrs(sums)
fmt.Println()
}
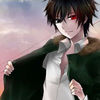
TA贡献1895条经验 获得超7个赞
我为您的场景创建了一个示例代码。您可以使用修改它bufio.NewReader(os.Stdin)
func process_array(arr []string) int {
res := 0
for _, v := range arr {
num, err := strconv.Atoi(v)
if err != nil {
panic(err)
}
fmt.Println("num :", num)
res += num * num
}
return res
}
func process_test_case() int {
fmt.Println(`Enter the number of numbers`)
num := 2
fmt.Println("number of numbers :", num)
fmt.Println(`Enter the numbers`)
input := "1 2"
fmt.Println("the numbers :", input)
arr := strings.Split(input, " ")
res := process_array(arr)
return res
}
func main() {
fmt.Println(`Enter the number of inputs`)
test_cases := 1
fmt.Println("number of inputs :", test_cases)
for test_cases >= 1 {
res := process_test_case()
fmt.Println(res)
test_cases -= 1
}
}
你可以在这里运行它:https ://go.dev/play/p/zGkAln2ghZp
或者
正如@phonaputer 评论的那样,您可以更改顺序。返回切片并从末尾打印。
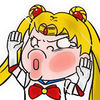
TA贡献1856条经验 获得超11个赞
我认为这段代码回答了您的问题标题:
package main
import "fmt"
func SumValues(x int, y ...int) (sum int) {
q := len(y) - 1
sum = y[q - x]
if x < q {
sum += SumValues(x + 1, y...)
}
return sum
}
func main() {
sum := SumValues(0,1,2,3,4,5)
fmt.Println("Sum is:", sum)
}
- 3 回答
- 0 关注
- 93 浏览
添加回答
举报