3 回答
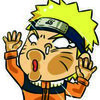
TA贡献1936条经验 获得超6个赞
在阅读了mlibby的评论(以百万为单位的值)之后,我决定从头开始。
结果真的很简单!
我还添加了一个界面以更易读的方式显示结果
const dataBase =
{ zero: 0, one: 1, two: 2, three: 3, four: 4
, five: 5, six: 6, seven: 7, eight: 8, nine: 9, ten: 10
, eleven: 11, twelve: 12, thirteen: 13, fourteen: 14
, fifteen: 15, sixteen: 16, seventeen: 17, eighteen: 18
, nineteen: 19, twenty: 20, thirty: 30, forty: 40
, fifty: 50, sixty: 60, seventy: 70, eighty: 80, ninety: 90
, hundred: 100, thousand: 1000, million: 1000000, and: 0
}
const parseVal=s=>
s.match(/\w+/g)
.reduce((tots,sVal)=>
{
let n = tots.length -1
switch (sVal) {
case 'hundred':
tots[n] *= 100
break
case 'thousand':
case 'million':
tots[n] *= dataBase[sVal]
tots.push(0)
break
default:
tots[n] += dataBase[sVal]
break;
}
return tots
},[0]).reduce((a,c)=>a+c);
// testing part...
const myTable = document.getElementById('my-table')
for(let r=1;r<myTable.rows.length;++r )
{
let rowCell = myTable.rows[r].cells
rowCell[1].textContent = parseVal(rowCell[0].textContent).toLocaleString('en')
if (rowCell[1].textContent === rowCell[3].textContent)
{
rowCell[2].textContent = 'OK'
rowCell[2].className = 'isOK'
}
else
{
rowCell[2].textContent = 'bad'
rowCell[2].className = 'isBad'
}
}
table {
border-collapse: collapse;
margin-top: 25px;
font-family: Arial, Helvetica, sans-serif;
font-size: 12px;
}
thead {
background-color: aquamarine;
}
tbody {
background-color: #e1e7ee;
}
td {
border: 1px solid grey;
padding: .3em .7em;
}
td:nth-child(2),
td:nth-child(4) {
text-align: right;
}
td:nth-child(3) { text-align: center; }
td.isOK { color:green }
td.isBad { color:red }
<table id="my-table">
<thead>
<tr>
<td>text value</td> <td>Parsed</td> <td>validation</td><td>expected</td>
</tr>
</thead>
<tbody>
<tr>
<td>two hundred forty-six</td>
<td>...</td>
<td>...</td>
<td>246</td>
</tr>
<tr>
<td>two hundred thousand</td>
<td>...</td>
<td>...</td>
<td>200,000</td>
</tr>
<tr>
<td>two hundred thousand and twelve</td>
<td>...</td>
<td>...</td>
<td>200,012</td>
</tr>
<tr>
<td>one hundred seventy-one</td>
<td>...</td>
<td>...</td>
<td>171</td>
</tr>
<tr>
<td>one thousand two hundred and thirty</td>
<td>...</td>
<td>...</td>
<td>1,230</td>
</tr>
<tr>
<td>three hundred and twenty one million four hundred thirty three thousand seven hundred and eight</td>
<td>...</td>
<td>...</td>
<td>321,433,708</td>
</tr>
</tbody>
</table>
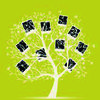
TA贡献1873条经验 获得超9个赞
正如我在评论中指出的那样,您的转换没有做您想要的,您需要反向迭代整个数组以使用您当前的技术。所以我重构了你的代码来演示。我改变了什么?
我用我将使用的名称替换了变量名称(命名是主观的,但我更喜欢它们是描述性的)。
下一步: String.split() 可以采用正则表达式,并且能够一次拆分多个不同的字符。正则表达式/[ -]/仅表示在空格或破折号的任何字符上拆分。
然后: Array.map() 允许我们通过使用指定的函数转换每个元素来从现有数组创建一个新数组(在这种情况下,该函数正在从您的单词“数据库”中查找整数)。
最后,我们遍历数字数组,将它们适当地添加到最终总和中。
function parseInt(string) {
const wordNumbers = {
'zero': 0,
'one': 1,
'two': 2,
'three': 3,
'four': 4,
'five': 5,
'six': 6,
'seven': 7,
'eight': 8,
'nine': 9,
'ten': 10,
'eleven': 11,
'twelve': 12,
'thirteen': 13,
'fourteen': 14,
'fifteen': 15,
'sixteen': 16,
'seventeen': 17,
'eighteen': 18,
'nineteen': 19,
'twenty': 20,
'thirty': 30,
'forty': 40,
'fifty': 50,
'sixty': 60,
'seventy': 70,
'eighty': 80,
'ninety': 90,
'hundred': 100,
'thousand': 1000,
'million': 1000000,
'and': 0
}
let words = string.split(/[ -]/);
console.log(words);
let numbers = words.map(word => wordNumbers[word]);
numbers.reverse();
console.log(numbers);
let result = 0;
for(let i = 0; i < numbers.length; i++) {
if(numbers[i] < 100) {
result += numbers[i];
}
else {
if(i + 2 < numbers.length && numbers[i + 1] < numbers[i] && numbers[i + 2] < numbers [i + 1]) {
result += numbers[i] * numbers[i + 1] * numbers[i + 2];
i++; i++;// need to skip the next two elements of ints in the for loop
}
else if(i + 1 < numbers.length && numbers[i + 1] < numbers[i]) {
result += numbers[i] * numbers[i + 1];
i++; // need to skip the next element of ints in the for loop
}
else {
result += numbers[i];
}
}
}
return result;
}
console.log(parseInt('two hundred forty-six')); //246
console.log(parseInt("one hundred seventy-one")); //171
console.log(parseInt('one thousand two hundred and thirty')); // 1230
console.log(parseInt('twenty million')); // 20000000
console.log(parseInt('two hundred thousand')); // 200000
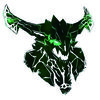
TA贡献1785条经验 获得超4个赞
这适用于诸如二十万之类的条目...
function parseInt(string) {
var output = [];
var dataBase = {
'zero': 0,
'one': 1,
'two': 2,
'three': 3,
'four': 4,
'five': 5,
'six': 6,
'seven': 7,
'eight': 8,
'nine': 9,
'ten': 10,
'eleven': 11,
'twelve': 12,
'thirteen': 13,
'fourteen': 14,
'fifteen': 15,
'sixteen': 16,
'seventeen': 17,
'eighteen': 18,
'nineteen': 19,
'twenty': 20,
'thirty': 30,
'forty': 40,
'fifty': 50,
'sixty': 60,
'seventy': 70,
'eighty': 80,
'ninety': 90,
'hundred': 100,
'thousand': 1000,
'million': 1000000,
'and': 0
}
let clarray=[]
filteroutp=[]
fo=[]
var f
var acc=[]
var res=0
const strarray= string.split(' ')
strarray.forEach((n,i)=>{
if(n.includes('-')) {
nm=n.split('-'), clarray.push(...nm)
}
else clarray.push(n)
})
Object.entries(dataBase).forEach(o=>{
clarray.forEach((x,i)=>{if (x==o[0]) output[i]=o[1] })})
if(output[output.length-1]&&output[output.length-2]<100&&output.length-2!=0){
n=output.pop() , f=output.pop()+n
}
else f=0
for(let i=0;i<output.length;i++){
n=output[i]
m= output[i+1]
if(m=="undefined") m=1
if(output.length>1){
if(n<m ){
if(acc.length!=0){
accn= acc.shift()
accn<n? fo.push(accn*n*m):fo.push(accn+n*m)
output.splice(i,2)
i=-2
}
else if(acc.length==0) {
acc.push(n*m)
if(output.length>1){
output.splice(i,2)
i=-2
}
if(output.length==1){
fo.push(acc.shift()*output[0])
output.splice(0,1)
i=-1
}
}
}
}
if(output.length==0&&acc.length!=0) fo.push(acc.shift())
}
fo.push(f)
for(let n of fo){
res+=n
}
return "output "+`${fo}` + " number "+res
}
console.log(parseInt('two hundred forty-six')); //246
console.log(parseInt("one hundred seventy-one")); //171
console.log(parseInt('one thousand two hundred and thirty')); //1230
console.log(parseInt('one million one thousand two hundred and thirty')); // 1001230
console.log(parseInt('ten million one-thousand two hundred and thirty-two')); // 10001232
console.log(parseInt('one thousand seventeen hundred and thirty')); // 2730
console.log(parseInt('two hundred thousand')) //200000
添加回答
举报