3 回答
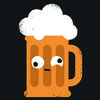
TA贡献2016条经验 获得超9个赞
您可以使用它collections.deque
来有效地实现您正在寻找的算法。这是因为双端队列两侧的追加和弹出O(1)
在任一方向的复杂性下都是有效的。
利用:
from collections import deque
def word_magic(string):
tokens = deque(string.split())
words = []
while tokens:
curr_word = tokens.popleft()
if not tokens:
words.append(curr_word)
break
next_word = tokens[0]
if curr_word[-1] == next_word[0]:
tokens.popleft()
tokens.appendleft(next_word + curr_word)
continue
words.append(curr_word)
return words
调用函数:
# Example 1
words = word_magic("are all levels lavendar lemon maverick king of gamblers")
print("\n".join(words))
# Example 2
words = word_magic("crab boy yesterday yellow wing game engine eat top")
print("\n".join(words))
这打印:
are
lemonlavendarlevelsall
kingmaverick
of
gamblers
boycrab
yellowyesterday
gamewing
eatengine
top
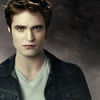
TA贡献1830条经验 获得超9个赞
s = "are all levels lavendar lemon maverick king of gamblers".split()
words = list(reversed(s)) # Reverse the list because we're poping from the end
while words:
first = words.pop()
rest = []
while words and words[-1][0] == first[-1]:
rest.append(words.pop())
print("".join([*rest, first]))
对于每个单词,当您发现以与该单词(您所在的单词)结尾相同的字母开头的单词时,请向前看。然后打印那些单词(以字母开头),然后是那个单词(以字母结尾的单词),然后跳到你找到的最后一个单词之后的单词。
您可以使用索引而不是编写相同的代码list.pop:
words = "are all levels lavendar lemon maverick king of gamblers".split()
i = 0
while i < len(words):
first = words[i]
i += 1
rest = []
while i < len(words) and words[i][0] == first[-1]:
rest.append(words[i])
i += 1
print("".join([*rest, first]))
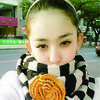
TA贡献1871条经验 获得超13个赞
没有对其进行基准测试,但我会尝试fold。functools 模块提供了reduce 功能:
>>> s = "are all levels lavendar lemon maverick king of gamblers"
在空格上拆分字符串:
>>> t = s.split(" ")
>>> t
['are', 'all', 'levels', 'lavendar', 'lemon', 'maverick', 'king', 'of', 'gamblers']
并折叠列表:
>>> import functools
>>> u = functools.reduce(lambda acc, x: acc[:-1] + [x+acc[-1]] if acc and x and acc[-1][-1] == x[0] else acc + [x], t, [])
>>> u
['are', 'lemonlavendarlevelsall', 'kingmaverick', 'of', 'gamblers']
我们从一个空列表开始[]。有两种情况:如果前一个词(或压缩词)的最后一个字母是当前词的第一个字母(acc[-1][-1] == x[0]),那么我们在前一个词(或压缩词)之前插入当前词:acc[:-1] + [x+acc[-1]]。否则,我们将当前单词添加到列表中。
现在只需打印结果:
>>> print("\\n".join(u))
are
lemonlavendarlevelsall
kingmaverick
of
gamblers
另一个版本,基于观察如果我们有一个单词w[i]的最后一个字母c=w[i][-1]so w[i+1][0] = c,那么我们构建w[i+1]+w[i]并且最后一个字母将c再次出现:我们只需要检查下一个单词是否w[i+2], w[i+3], ...以cetc 开头。如果没有,开始一个新的线。
s = "are all levels lavendar lemon maverick king of gamblers"
t = s.split(" ")
i = 0
while i < len(t):
c = t[i][-1]
j = i+1
cur = [t[i]]
while j<len(t) and t[j][0] == c:
cur.insert(0, t[j])
j += 1
print("".join(cur))
i = j
添加回答
举报