2 回答
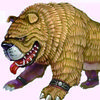
TA贡献1810条经验 获得超5个赞
此代码可以帮助您:
# Assuming a random initial list:
data = [["a",1,3,4,4,2,"b",2,2,3,5,2,3,"c",4,3,5,5]]
# An empty list where it will be added the result:
new_data = []
# Variable to accumulate the sum of every letter:
sume = 0
# FOR loop to scan the "data" variable:
for i in data[0]:
# If type of the i variable is string, we assume it's a letter:
if type(i) == str:
# Add accumulated sum
new_data.append(sume)
# We restart *sume* variable:
sume = 0
# We add a new letter read:
new_data.append(i)
else:
# We accumulate the sum of each letter:
sume += i
# We extract the 0 added initially and added the last sum:
new_data = new_data[1::]+[sume]
# Finally, separate values in pairs with a FOR loop and add it to "new_data2":
new_data2 = []
for i in range(len(new_data)//2):
pos1 = i*2
pos2 = pos1+1
new_data2.append([new_data[pos1],new_data[pos2]])
# Print data and new_data2 to verify results:
print (data)
print (new_data2)
# Pause the script:
input()
此代码可以通过脚本运行一次,但它可以转换为嵌套函数,以便以您正在寻找的方式使用它。
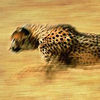
TA贡献1860条经验 获得超9个赞
通常希望您首先发布您的解决方案,但您似乎已经尝试了一些事情并需要帮助。对于未来的问题,请确保包含您的尝试,因为它有助于我们提供更多帮助,说明您的解决方案为何不起作用,以及您可以采取哪些额外步骤来改进您的解决方案。
假设您的列表总是以字母 or 开头str,并且所有数字都是 type int,您可以使用字典来进行计数。我添加了注释来解释逻辑。
def group_consecutive(lst):
groups = {}
key = None
for item in lst:
# If we found a string, set the key and continue to next iteration immediately
if isinstance(item, str):
key = item
continue
# Add item to counts
# Using dict.get() to initialize to 0 if ket doesn't exist
groups[key] = groups.get(key, 0) + item
# Replacing list comprehension: [[k, v] for k, v in groups.items()]
result = []
for k, v in groups.items():
result.append([k, v])
return result
然后你可以这样调用函数:
>>> group_consecutive(["a",1,3,4,"b",2,2,"c",4,5])
[['a', 8], ['b', 4], ['c', 9]]
更好的解决方案可能会使用collections.Counter
或collections.defaultdict
进行计数,但由于您提到没有导入,因此上述解决方案坚持这一点。
添加回答
举报