3 回答
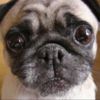
TA贡献1735条经验 获得超5个赞
我认为你只需要缓存原始console方法并从缓存中调用它们——你现在拥有它的方式调用你的 stubbed log,这会导致无限递归(从而导致堆栈溢出):
$(document).ready(function(){
console.log('You should know...');
console.error('Something went wrong...');
console.warn('Look out for this...');
})
// This should work
var native = window;
var originalConsole = native.console;
native.console = {
log: function(message){
$('ul.messages').append('<li>Log: ' + message + '</li>');
originalConsole.log(message);
},
error: function(message){
$('ul.messages').append('<li>Error: ' + message + '</li>');
originalConsole.error(message);
},
warn: function(message){
$('ul.messages').append('<li>Warn: ' + message + '</li>');
originalConsole.warn(message);
}
}
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<h3>messages</h3>
<ul class="messages"></ul>
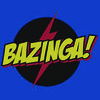
TA贡献1789条经验 获得超8个赞
您可以创建一个包装函数,该函数接受一个函数并输出您修改后的函数。
const wrapper = (fn, name) => {
return function(msg) {
$('ul.messages').append(`<li>${name}: ${msg}</li>`);
fn(msg);
};
}
$(document).ready(() => {
window.console.log = wrapper(console.log, "Log");
window.console.warn = wrapper(console.warn, "Warn");
window.console.error = wrapper(console.error, "Error");
});
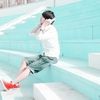
TA贡献1719条经验 获得超6个赞
我不建议您修改原始功能,您可以创建一个新功能,并且可以在控制台和您的节点上显示消息。
Blow 是纯JavaScript 代码。
示例 1
function decorator(wrap, func) {
return (...para) => {
func(para)
return wrap(para)
}
}
const mylog = decorator(window.console.log, (...para)=>{
const ul = document.querySelector(`ul[class=msg]`)
const range = document.createRange()
const frag = range.createContextualFragment(`<li>${para}</li>`)
ul.append(frag)
})
mylog("Hello world!")
<h3>messages</h3>
<ul class="msg"></ul>
示例 2
window.onload = () => {
const decoratorFunc = (methodName, msg) => {
const symbol = methodName === "info" ? "🐬" :
methodName === "error" ? "❌" :
methodName === "warn" ? "⚠" : ""
const ul = document.querySelector(`ul[class=msg]`)
const range = document.createRange()
const frag = range.createContextualFragment(`<li>${symbol} ${msg}</li>`)
ul.append(frag)
}
const myConsole = new MethodDecorator(window.console, decoratorFunc)
myConsole.Apply(["log", "info", "error", "warn", // those will run {decoratorFunc + ``window.console.xxx``}
"others" // 👈 I created, it doesn't belong method of window.console so it only run ``decoratorFunc``
])
myConsole.log("log test")
myConsole.info("info test")
console.info("not influenced") // not influenced
myConsole.error("error test")
myConsole.warn("warn test")
myConsole.others("others test")
myConsole.Reset()
// myConsole.warn("warn test") // error
}
class MethodDecorator {
constructor(obj, func) {
this.obj = obj
this.func = func
}
Apply(nameArray) {
const orgMethodNameArray = Object.getOwnPropertyNames(this.obj)
for (const methodName of nameArray) {
const orgMethodName = orgMethodNameArray.find(e => e === methodName) // if not found return undefined
const prop = {}
prop[methodName] = {
"configurable": true,
"value": (...args) => {
this.func(methodName, args) // decorator function
if (orgMethodName) {
this.obj[orgMethodName](args) // for example, console.log(args)
}
}
}
Object.defineProperties(this, prop)
}
}
Reset() {
const extraMethodNameArray = Object.getOwnPropertyNames(this).filter(name => name !== "obj" || name !== "func")
for (const extraMethodName of extraMethodNameArray) {
const prop = {}
prop[extraMethodName] = {
value: undefined
}
Object.defineProperties(this, prop)
}
}
}
<h3>messages</h3>
<ul class="msg"></ul>
👆 Run code snippet的控制台会屏蔽部分内容。单击运行代码片段后单击整页以查看所有内容。
添加回答
举报