3 回答
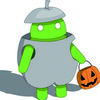
TA贡献1846条经验 获得超7个赞
您可以遍历 中的每个键值对对象,以查看哪个键属于当前对象。获得适用于当前对象的键后,您可以访问其关联的数组以及需要查看的索引。找到键值对后,可以从条目中删除键值对,然后再次以递归方式查找新对象中的下一个键。您可以继续此操作,直到找不到属于当前对象的键(即:findIndex返回-1);menuIndexespathToParsemenuIndexesdata
const pathToParse = { buildings: [ { name: 'office', building_styles: [ { name: 'interior', rooms: [ { name: 'open space', filling_types: [ { name: 'furniture', inventories: [{ id: 1, name: 'tv' }, { id: 2, name: 'chair' }, { id: 3, name: 'table' }, ] } ] } ] }, ] }, ] }
const menuIndexes = {
buildings: 0,
building_styles: 0,
rooms: 0,
room_fillings: 0,
filling_types: 0,
}
function getPath(data, entries) {
const keyIdx = entries.findIndex(([key]) => key in data);
if(keyIdx <= -1)
return [];
const [objKey, arrIdx] = entries[keyIdx];
const obj = data[objKey][arrIdx];
entries.splice(keyIdx, 1);
return [obj.name].concat(getPath(obj, entries));
}
console.log(getPath(pathToParse, Object.entries(menuIndexes)));
的用途是搜索对象以查找要查看的键(因为我们不想依赖于对象的键排序)。如果您对 有更多的控制权,则可以将其存储在一个数组中,您可以在其中安全地依赖元素的顺序,从而依赖键:Object.entries()datamenuIndexesmenuIndexes
const pathToParse = { buildings: [ { name: 'office', building_styles: [ { name: 'interior', rooms: [ { name: 'open space', filling_types: [ { name: 'furniture', inventories: [{ id: 1, name: 'tv' }, { id: 2, name: 'chair' }, { id: 3, name: 'table' }, ] } ] } ] }, ] }, ] };
const menuIndexes = [{key: 'buildings', index: 0}, {key: 'building_styles', index: 0}, {key: 'rooms', index: 0}, {key: 'filling_types', index: 0}, {key: 'inventories', index: 0}, ];
function getPath(data, [{key, index}={}, ...rest]) {
if(!key)
return [];
const obj = data[key][index];
return [obj.name, ...getPath(obj, rest)];
}
console.log(getPath(pathToParse, menuIndexes));
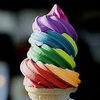
TA贡献1780条经验 获得超1个赞
像往常一样,我会在一些可重用的部件上构建这样的函数。这是我的方法:
// Utility functions
const path = (obj, names) =>
names .reduce ((o, n) => (o || {}) [n], obj)
const scan = (fn, init, xs) =>
xs .reduce (
(a, x, _, __, n = fn (a .slice (-1) [0], x)) => [...a, n],
[init]
) .slice (1)
const pluck = (name) => (xs) =>
xs .map (x => x [name])
// Main function
const getBreadCrumbs = (data, indices) =>
pluck ('name') (scan (path, data, Object .entries (indices)))
// Sample data
const data = {buildings: [{name: "office", building_styles: [{building_style: 0}, {building_style: 1}, {building_style: 2}, {name: "interior", rooms: [{room: 0}, {room: 1}, {name: "open space", filling_types: [{filling_type: 0}, {name: "furniture", room_fillings: [{name: "items", inventories: [{id: 1, name: "tv"}, {id: 2, name: "chair"}, {id: 3, name: "table"}]}, {room_filling: 1}]}, {filling_type: 2}]}, {room: 3}]}, {building_style: 4}]}]}
const menuIndexes = {buildings: 0, building_styles: 3, rooms: 2, filling_types: 1, room_fillings: 0}
// Demo
console .log (getBreadCrumbs (data, menuIndexes))
我们这里有三个可重用的函数:
path
采用一个对象和节点名称(字符串或整数)列表,并在给定路径处返回值,或者如果缺少任何节点,则返回值。1 例如:undefined
path ({a: {b: [{c: 10}, {c: 20}, {c: 30}, {c: 40}]}}, ['a', 'b', 2, 'c']) //=> 30.
scan
与 非常相似,不同之处在于它不返回最终值,而是返回在每个步骤中计算的值的列表。例如,如果 是 ,则:Array.prototype.reduce
add
(x, y) => x + y
scan (add, 0, [1, 2, 3, 4, 5]) //=> [1, 3, 6, 10, 15]
拔出
将命名属性从每个对象列表中拉出:pluck ('b') ([{a: 1, b: 2, c: 3}, {a: 10, b: 20, c: 30}, {a: 100, b: 200, c: 300}]) //=> [2, 20, 200]
在实践中,我实际上会进一步考虑这些帮助器,根据 定义来定义,并在定义中使用和。但这对这个问题并不重要。path
const prop = (obj, name) => (obj || {}) [name]
const last = xs => xs.slice (-1) [0]
const tail = (xs) => xs .slice (-1)
scan
然后,我们的 main 函数可以简单地使用这些条目以及 2,首先从索引中获取条目,将该条目、我们的函数和数据传递给相关对象节点的列表,然后将结果与我们要提取的字符串一起传递给。Object.entries
path
scan
pluck
'name'
我几乎每天都使用。 不太常见,但它足够重要,它被包含在我通常的实用程序库中。有了这样的功能,编写类似 的东西就非常简单了。path
pluck
scan
getBreadCrumbs
1 旁注,我通常将其定义为,我发现这是最常用的。此表单恰好更适合所使用的代码,但是将代码调整为我的首选形式很容易:而不是 ,我们可以只编写(names) => (obj) => ...
scan (path, data, ...)
scan ((a, ns) => path (ns) (a), data, ...)
2 正如尼克·帕森斯(Nick Parsons)的回答和感谢的评论所指出的那样,将此信息存储在一个显式排序的数组中是有一个很好的论据,而不是依赖于一般对象获得的奇怪和任意的顺序。如果这样做,则此代码只能通过删除 main 函数中的调用来更改。Object .entries
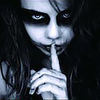
TA贡献1840条经验 获得超5个赞
您可以像这样定义一个递归函数,它循环访问键并在数据中找到相应的对象。找到数据后,它会将名称推送到输出数组中,并使用此对象再次调用该函数,并且menuIndexesmenuIndexes
const displayBreadCrumbs = (data, menuIndexes) => {
const output = [];
Object.keys(menuIndexes).forEach(key => {
if (data[key] && Array.isArray(data[key]) && data[key][menuIndexes[key]]) {
output.push(data[key][menuIndexes[key]].name);
output.push(...displayBreadCrumbs(data[key][menuIndexes[key]], menuIndexes));
}
});
return output;
};
const data = { buildings: [ { name: 'office', building_styles: [ { name: 'interior', rooms: [ { name: 'open space', filling_types: [ { name: 'furniture', inventories: [{ id: 1, name: 'tv' }, { id: 2, name: 'chair' }, { id: 3, name: 'table' }, ] } ] } ] }, ] }, ] };
const menuIndexes = {
buildings: 0,
building_styles: 0,
rooms: 0,
room_fillings: 0,
filling_types: 0,
};
displayBreadCrumbs(data, menuIndexes); // ["office", "interior", "open space", "furniture"]
添加回答
举报