4 回答
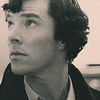
TA贡献2011条经验 获得超2个赞
更新:
因为OP似乎对语言不是很有经验,所以我选择提供一个易于阅读的答案。正确的方法是使用正则表达式捕获组:https://stackoverflow.com/a/18647776/16805283
这将检查“message.content”中是否有任何引号,并更改获取 args 数组的方式。如果未找到引号,它将回退到您自己的代码以生成 args 数组。请记住,这只有在“message.content”上正好有2个**引号时才有效,因此不应在原因中使用引号
// Fake message object as an example
const message = {
content: '!command "Player name with a lot of spaces" reason1 reason2 reason3'
};
const { content } = message;
let args = [];
if (content.indexOf('"') >= 0) {
// Command
args.push(content.slice(0, content.indexOf(' ')));
// Playername
args.push(content.slice(content.indexOf('"'), content.lastIndexOf('"') + 1));
// Reasons
args.push(content.slice(content.lastIndexOf('"') + 2, content.length));
} else {
args = content.split(' ');
}
// More code using args
如果你想要不带引号的玩家名称:
playerName = args[1].replace(/"/g, '');`
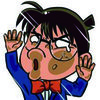
TA贡献1872条经验 获得超3个赞
你可以在这里使用正则表达式:
const s = `!command "player one" some reason`;
function getArgsFromMsg1(s) {
const args = []
if ((/^!command/).test(s)) {
args.push(s.match(/"(.*)"/g)[0].replace(/\"/g, ''))
args.push(s.split(`" `).pop())
return args
} else {
return 'not a command'
}
}
// more elegant, but may not work
function getArgsFromMsg2(s) {
const args = []
if ((/^!command/).test(s)) {
args.push(...s.match(/(?<=")(.*)(?=")/g))
args.push(s.split(`" `).pop())
return args
} else {
return 'not a command'
}
}
console.log(getArgsFromMsg1(s));
console.log(getArgsFromMsg2(s));
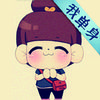
TA贡献1772条经验 获得超5个赞
我建议你单独解析命令和它的参数。它将承认编写更灵活的命令。例如:
var command = msg.content.substring(0, msg.content.indexOf(' '));
var command_args_str = msg.content.substring(msg.content.indexOf(' ') + 1);
switch(command) {
case '!command':
var player = command_args_str.substring(0, command_args_str.lastIndexOf(' '));
var reason = command_args_str.substring(command_args_str.lastIndexOf(' ') + 1);
break;
}
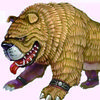
TA贡献1833条经验 获得超4个赞
这是可能的,但对于机器人的用户来说,只使用不同的字符来拆分两个参数可能不那么复杂。
例如 如果您知道(或 ,等)不是有效用户名的一部分。!command player one, reasons,|=>
如果您只支持用户名部分的引号,则更容易:
const command = `!command`;
const msg1 = {content: `${command} "player one" reasons ggg`};
const msg2 = {content: `${command} player reasons asdf asdf`};
function parse({content}) {
if (!content.startsWith(command)) {
return; // Something else
}
content = content.replace(command, '').trim();
const quotedStuff = content.match(/"(.*?)"/);
if (quotedStuff) {
return {player: quotedStuff[1], reason: content.split(`"`).reverse()[0].trim()};
} else {
const parts = content.split(' ');
return {player: parts[0], reason: parts.slice(1).join(' ')};
}
console.log(args);
}
[msg1, msg2].forEach(m => console.log(parse(m)));
添加回答
举报