2 回答
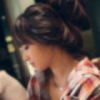
TA贡献1818条经验 获得超7个赞
下面是一个使用倒计时批处理的示例。
package chapter13;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class BST {
public static void main(String[] args) throws InterruptedException {
final CountDownLatch latch = new CountDownLatch(1);
final ExecutorService executorService = Executors.newCachedThreadPool();
Runnable runnableA = () -> {
System.out.println("Runnable A");
latch.countDown();
System.out.println("Runnable A finished");
};
Runnable runnableB = () -> {
System.out.println("Runnable B");
executorService.submit(runnableA);
try {
System.out.println("Runnable B waiting for A to complete");
latch.await();
System.out.println("Runnable B finished");
} catch (InterruptedException e) {
System.out.println("Thread interrupted");
Thread.currentThread().interrupt();
}
};
executorService.submit(runnableB);
Thread.sleep(10);
shutDown(executorService);
}
private static void shutDown(ExecutorService executorService) {
executorService.shutdown();
try {
if (!executorService.awaitTermination(1, TimeUnit.SECONDS)) {
executorService.shutdownNow();
}
} catch (InterruptedException e) {
executorService.shutdownNow();
}
}
}
我使用方法使主线程休眠,因为在提交任务 B 后立即关闭池可能会导致池在任务 B 提交任务 A 之前停止接受新任务。Thread.sleep()
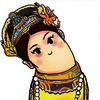
TA贡献1820条经验 获得超2个赞
一种方法是使用java锁定方法。例如:
private AtomicBoolean processed = new AtomicBoolean(true) ;
private String result = null ;
public String doAndWait()
{
synchronized(processed) {
doSomethingAsync() ;
processed.wait();
}
return result ;
}
public void doSomethingAsync()
{
...
result="OK";
synchronized(processed) {
processed.notify();
}
}
添加回答
举报