2 回答
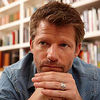
TA贡献1784条经验 获得超7个赞
如果我改变文本,它也会自动改变风格
选项 1:按 id
控制样式 可以通过使用自定义标签来实现此目的,该标签在更改文本时更改样式。我将通过更改标签的 ID 来演示它。这个简化的示例使用文本作为 id:
import javafx.geometry.Pos;
import javafx.scene.control.Label;
public class CustomLabel extends Label{
public CustomLabel() {
setAlignment(Pos.CENTER);
setPrefSize(50, 25);
}
void setTextAndId(String s){
super.setText(s);
/*To keep this demo simple and clear id is changed.
If used, care must be taken to keep id unique.
Using setStyle() or PseudoClass should be preferred
*/
setId(s);
}
}
自定义标签可用于 fxml ():Root.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.layout.StackPane?>
<?import tests.CustomLabel?>
<StackPane fx:id="root" xmlns="http://javafx.com/javafx/10.0.1" xmlns:fx="http://javafx.com/fxml/1"
fx:controller="tests.Controller">
<children>
<CustomLabel fx:id="cLabel" text="""" />
</children>
</StackPane>
一个简单的 css,它根据 id () 更改背景颜色:Root.css
#1{
-fx-background-color: red;
-fx-text-fill: white;
}
#2{
-fx-background-color: yellow;
-fx-text-fill: red;
}
#3{
-fx-background-color: green;
-fx-text-fill: yellow;
}
测试类:
import java.io.IOException;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class LabelCssTest extends Application {
@Override public void start(Stage stage) throws IOException {
Parent root = FXMLLoader.load(getClass().getResource("Root.fxml"));
stage.setScene(new Scene(root));
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
和测试控制器:
import javafx.animation.PauseTransition;
import javafx.fxml.FXML;
import javafx.scene.Parent;
import javafx.util.Duration;
public class Controller {
@FXML
CustomLabel cLabel;
@FXML Parent root;
private static final int MIN_VALUE = 1, MAX_VALUE = 3;
private int counter = MIN_VALUE;
@FXML
private void initialize() {
root.getStylesheets().add(getClass().getResource("Root.css").toExternalForm());
cLabel.setTextAndId(String.valueOf(counter++));
PauseTransition pause = new PauseTransition(Duration.seconds(2));
pause.setOnFinished(event ->{
cLabel.setTextAndId(String.valueOf(counter++));
if(counter > MAX_VALUE) {
counter = MIN_VALUE;
}
pause.play();
});
pause.play();
}
}
选项 2:通过更改样式类
来控制样式 使用与选项 1 相同的测试类。
Root.fxml:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.layout.StackPane?>
<StackPane fx:id="root" xmlns="http://javafx.com/javafx/10.0.1" xmlns:fx="http://javafx.com/fxml/1" fx:controller="tests.Controller">
<children>
<Label fx:id="label" alignment="CENTER" contentDisplay="CENTER" prefHeight="20.0" prefWidth="70.0" text="" "" />
</children>
</StackPane>
Root.css:
.style1{
-fx-background-color: red;
-fx-text-fill: white;
}
.style2{
-fx-background-color: yellow;
-fx-text-fill: red;
}
.style3{
-fx-background-color: green;
-fx-text-fill: yellow;
}
和控制器:
import javafx.animation.PauseTransition;
import javafx.beans.binding.Bindings;
import javafx.beans.property.SimpleIntegerProperty;
import javafx.beans.value.ChangeListener;
import javafx.fxml.FXML;
import javafx.scene.Parent;
import javafx.scene.control.Label;
import javafx.util.Duration;
public class Controller {
@FXML Label label;
@FXML Parent root;
private static final int MIN_VALUE = 1, MAX_VALUE = 3;
private SimpleIntegerProperty counter = new SimpleIntegerProperty();
@FXML
private void initialize() {
root.getStylesheets().add(getClass().getResource("Root.css").toExternalForm());
counter = new SimpleIntegerProperty();
counter.addListener((ChangeListener<Number>) (observable, oldValue, newValue) -> {
label.getStyleClass().clear();
label.getStyleClass().add("style"+counter.get());
});
label.textProperty().bind(Bindings.createStringBinding(()->String.valueOf(counter.get()), counter));
counter.set(1);
PauseTransition pause = new PauseTransition(Duration.seconds(2));
pause.setOnFinished(event ->{
counter.set(counter.get() >= MAX_VALUE ? MIN_VALUE : counter.get()+1);
pause.play();
});
pause.play();
}
}
Option 3: control style by using :
Changes from option 2:
:PseudoClassRoot.css
.root:style1 #label{
-fx-background-color: red;
-fx-text-fill: white;
}
.root:style2 #label{
-fx-background-color: yellow;
-fx-text-fill: red;
}
.root:style3 #label{
-fx-background-color: green;
-fx-text-fill: yellow;
}
And controller:
import javafx.animation.PauseTransition;
import javafx.beans.binding.Bindings;
import javafx.beans.property.SimpleIntegerProperty;
import javafx.beans.value.ChangeListener;
import javafx.css.PseudoClass;
import javafx.fxml.FXML;
import javafx.scene.Parent;
import javafx.scene.control.Label;
import javafx.util.Duration;
public class Controller {
@FXML Label label;
@FXML Parent root;
private static final int MAX_VALUE = 3;
private SimpleIntegerProperty counter = new SimpleIntegerProperty(1);
@FXML
private void initialize() {
root.getStylesheets().add(getClass().getResource("Root.css").toExternalForm());
counter = new SimpleIntegerProperty();
counter.addListener((ChangeListener<Number>) (observable, oldValue, newValue) -> {
updateStates();
});
label.textProperty().bind(Bindings.createStringBinding(()->String.valueOf(counter.get()), counter));
counter.set(1);
PauseTransition pause = new PauseTransition(Duration.seconds(2));
pause.setOnFinished(event ->{
counter.set(counter.get() >= MAX_VALUE ? 1 : counter.get()+1);
pause.play();
});
pause.play();
}
private void updateStates() {
for( int index = 1; index <= MAX_VALUE; index++){
PseudoClass pc = PseudoClass.getPseudoClass("style"+String.valueOf(index));
root.pseudoClassStateChanged(pc, index == counter.get() ? true : false);
}
}
}
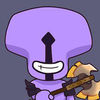
TA贡献1909条经验 获得超7个赞
如果您只想根据文本更改 的背景颜色,也可以创建一个这样的方法,并在需要时为每个方法调用它。LabelLabel
import javafx.scene.control.Label;
import javafx.geometry.Insets;
import javafx.scene.layout.Background;
import javafx.scene.layout.BackgroundFill;
import javafx.scene.layout.CornerRadii;
import javafx.scene.paint.Color;
private void backgroundColorTextDependent(Label label) {
if (label.getText().equals("1")) {
label.setBackground(new Background(new BackgroundFill(Color.BLACK, CornerRadii.EMPTY, Insets.EMPTY)));
} else if (label.getText().equals("2")) {
label.setBackground(new Background(new BackgroundFill(Color.YELLOW, CornerRadii.EMPTY, Insets.EMPTY)));
} else if (label.getText().equals("3")) {
label.setBackground(new Background(new BackgroundFill(Color.GREEN, CornerRadii.EMPTY, Insets.EMPTY)));
} else {
label.setBackground(new Background(new BackgroundFill(Color.BLUE, CornerRadii.EMPTY, Insets.EMPTY)));
}
添加回答
举报