3 回答
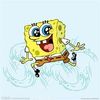
TA贡献1809条经验 获得超8个赞
这似乎做到了:
package main
type toto struct { name string }
func transform (data ...interface{}) {
t := data[0].(*toto)
t.name = "tutu"
}
func main() {
var titi toto
transform(&titi)
println(titi.name == "tutu")
}
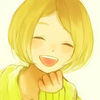
TA贡献1796条经验 获得超10个赞
听起来你想要一个指针。
在您的示例中,您使用了 一个数组,这有特殊原因吗?一般来说,你应该在 Go 中显式说明你的类型,特别是因为你正在处理一个简单的结构。interface{}
package main
import (
"log"
)
type toto struct {
Name string
}
// to help with printing
func (t *toto) String() string {
return t.Name
}
// transform takes an array of pointers to the toto struct
func transform(totos ...*toto) {
log.Printf("totos before: %v", totos)
// unsafe array access!
totos[0].Name = "tutu"
log.Printf("totos after: %v", totos)
}
func main() {
// variables in Go are usually defined like this
titi := toto{}
transform(&titi)
}听起来你想要一个指针。
在您的示例中,您使用了 一个数组,这有特殊原因吗?一般来说,你应该在 Go 中显式说明你的类型,特别是因为你正在处理一个简单的结构。interface{}
package main
import (
"log"
)
type toto struct {
Name string
}
// to help with printing
func (t *toto) String() string {
return t.Name
}
// transform takes an array of pointers to the toto struct
func transform(totos ...*toto) {
log.Printf("totos before: %v", totos)
// unsafe array access!
totos[0].Name = "tutu"
log.Printf("totos after: %v", totos)
}
func main() {
// variables in Go are usually defined like this
titi := toto{}
transform(&titi)
}
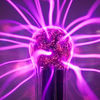
TA贡献1886条经验 获得超2个赞
在 Go 中,在函数中作为参数传递的变量实际上是变量的副本,而不是实际变量本身。如果要修改传递的变量,需要将其作为指针传入。
就个人而言,每当我创建一个接受结构的函数时,我都会将该函数设置为接受指向该结构的实例的指针。这具有更高的内存效率的好处(因为我的程序不必在每次调用函数时都创建变量的副本),并且它允许我修改我传递的结构的实例。
这就是我的做法:
package main
import (
"github.com/sirupsen/logrus"
)
type toto struct {
name string
}
func transform (t *toto) {
logrus.Info("t before: ", t)
// since t is a pointer to a toto struct,
// I can directly assign "tutu" to the "name" field
// using the "dot" operator
t.name = "tutu"
logrus.Info("t after: ", t)
}
func main() {
// init a pointer to a toto instance
titi := &toto{}
logrus.Info("titi before: ", titi) // -> empty
// this works because transform() accepts a pointer
// to a toto struct and and titi is a pointer to a toto instance
transform(titi)
logrus.Info("titi after (as a pointer): ", titi) // -> not empty
logrus.Info("titi after (as a value): ", *titi) // -> also not empty
}
- 3 回答
- 0 关注
- 71 浏览
添加回答
举报