2 回答
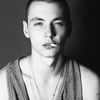
TA贡献1887条经验 获得超5个赞
以下是一些可以用作起点的 PHP 代码。https://github.com/docusign/eg-03-php-auth-code-grant/blob/master/src/EG001EmbeddedSigning.php
代码如下:
# 1. Create the envelope request object
$envelope_definition = $this->make_envelope($envelope_args);
# 2. call Envelopes::create API method
# Exceptions will be caught by the calling function
$config = new \DocuSign\eSign\Configuration();
$config->setHost($args['base_path']);
$config->addDefaultHeader('Authorization', 'Bearer ' . $args['ds_access_token']);
$api_client = new \DocuSign\eSign\ApiClient($config);
$envelope_api = new \DocuSign\eSign\Api\EnvelopesApi($api_client);
$results = $envelope_api->createEnvelope($args['account_id'], $envelope_definition);
$envelope_id = $results->getEnvelopeId();
# 3. Create the Recipient View request object
$authentication_method = 'None'; # How is this application authenticating
# the signer? See the `authenticationMethod' definition
# https://developers.docusign.com/esign-rest-api/reference/Envelopes/EnvelopeViews/createRecipient
$recipient_view_request = new \DocuSign\eSign\Model\RecipientViewRequest([
'authentication_method' => $authentication_method,
'client_user_id' => $envelope_args['signer_client_id'],
'recipient_id' => '1',
'return_url' => $envelope_args['ds_return_url'],
'user_name' => $envelope_args['signer_name'], 'email' => $envelope_args['signer_email']
]);
# 4. Obtain the recipient_view_url for the signing ceremony
# Exceptions will be caught by the calling function
$results = $envelope_api->createRecipientView($args['account_id'], $envelope_id,
$recipient_view_request);
我们有一个PHP库,可以在这里为您提供帮助 - https://github.com/docusign/docusign-php-client
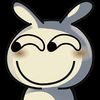
TA贡献1817条经验 获得超14个赞
function docusignSignature( $docusignTemplate, $userInput, $emailSettings )
{
// create configuration object and configure custom auth header
$config = new DocuSign\eSign\Configuration();
$config->setHost(ESIGN_HOST);
$config->addDefaultHeader("X-DocuSign-Authentication", "{\"Username\":\"" . ESIGN_USER . "\",\"Password\":\"" . ESIGN_PASS . "\",\"IntegratorKey\":\"" . ESIGN_KEY . "\"}");
//enable SSL_verification since we're on prod
$config->setSSLVerification(true);
// instantiate a new docusign api client
$apiClient = new DocuSign\eSign\ApiClient($config);
$accountId = null;
$user_id = $_SESSION['user']['id'];
$user_email = $_SESSION['user']['email'];
$user_name = $_SESSION['user']['name']." ({$_SESSION['user']['name']})";
if(empty($user_id) || empty($user_email)) {
echo json_encode(array( 'error'=>"Not logged in, please reload the page."
));
exit;
}
try
{
//*** STEP 1 - Login API: get first Account ID and baseURL
$authenticationApi = new DocuSign\eSign\Api\AuthenticationApi($apiClient);
$options = new \DocuSign\eSign\Api\AuthenticationApi\LoginOptions();
$loginInformation = $authenticationApi->login($options);
if(isset($loginInformation) && count($loginInformation) > 0)
{
$loginAccount = $loginInformation->getLoginAccounts()[0];
$host = $loginAccount->getBaseUrl();
$host = explode("/v2",$host);
$host = $host[0];
// UPDATE configuration object
$config->setHost($host);
// instantiate a NEW docusign api client (that has the correct baseUrl/host)
$apiClient = new DocuSign\eSign\ApiClient($config);
$accountId = $loginAccount->getAccountId();
if(!empty($accountId))
{
//*** STEP 2 - Signature Request from a Template
// create envelope call is available in the EnvelopesApi
$envelopeApi = new DocuSign\eSign\Api\EnvelopesApi($apiClient);
//Prepare an emailNotification
$emailNotification=new DocuSign\eSign\Model\RecipientEmailNotification();
//set email content
$emailNotification->setEmailBody($emailSettings['emailBody'])
->setEmailSubject($emailSettings['emailSubject'])
->setSupportedLanguage("en");
$textTabs=array();//prepare tabs (TextTab[])
$radioGroups=array();//prepare radioGroups
foreach ($userInput as $key => $value) {
if(is_null($value))continue;
if (is_array($value) && isset($value['type']) && $value['type']=='radio') {
$radio=new DocuSign\eSign\Model\Radio(
array(
'tab_id' =>$value['value'],
'value' =>$value['value'],
'selected' =>true
));
$radioGroups[]=new DocuSign\eSign\Model\RadioGroup(array(
'group_name' =>$key,
'radios' =>array($radio)
));
continue;
}
if(is_bool($value) || $value=='Yes') {
//just skip it (we changed checkboxes by radio)
continue;
}
$textTabs[]=new DocuSign\eSign\Model\Text(
array(
'tab_label' =>$key,//tab_label is the input name, see the template in the Account
'value' =>$value//value to be set to the input
));
}
$tabs=new DocuSign\eSign\Model\Tabs(['text_tabs'=>$textTabs]);
//radio buttons
if(!empty($radioGroups)){
$tabs->setRadioGroupTabs($radioGroups);
}
$templateRole = new DocuSign\eSign\Model\TemplateRole();
$templateRole->setEmail($user_email);
$templateRole->setName($user_name);
$templateRole->setTabs($tabs);
$templateRole->setEmailNotification($emailNotification);
$templateRole->setRoleName("role1");//check defined roles in your settings
$templateRoles=array($templateRole);
//a logic to Add a CC to all forms
$ccEmailNotif=new DocuSign\eSign\Model\RecipientEmailNotification();
//set email content
$ccEmailNotif->setEmailBody( CC_EMAIL_BODY )
->setEmailSubject( CC_EMAIL_SUBJECT )
->setSupportedLanguage("en");
$supportRole = new DocuSign\eSign\Model\TemplateRole();
$supportRole->setEmail( CC_EMAIL_DEFAULT )
->setName('Support')
->setEmailNotification($ccEmailNotif)
->setRoleName("support");
$templateRoles[]=$supportRole;
// instantiate a new envelope object and configure settings
$envelop_definition = new DocuSign\eSign\Model\EnvelopeDefinition();
$envelop_definition->setTemplateId($docusignTemplate);
$envelop_definition->setTemplateRoles($templateRoles);
// set envelope status to "sent" to immediately send the signature request
$envelop_definition->setStatus("sent");
// create and send the envelope (signature request)
$envelop_summary = $envelopeApi->createEnvelope($accountId, $envelop_definition);
if(!empty($envelop_summary))
{
//grap some infos from the evelope_summary
$envelopeID =$envelop_summary->getEnvelopeId();
$envelopeStatus =$envelop_summary->getStatus();
$envelopeDate =$envelop_summary->getStatusDateTime();
$filePath =__FILE__;
echo json_encode(
array( 'success'=>'An electronic signature is sent.')
);
exit;
}
}
}
}
catch (DocuSign\eSign\ApiException $ex)
{
// do something with the exception
}
}
- 2 回答
- 0 关注
- 117 浏览
添加回答
举报