1 回答
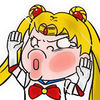
TA贡献1856条经验 获得超11个赞
发布我上面提供的代码实现提示,只需运行2次即可查看结果,这是控制器:
<?php
namespace App\Controller;
use App\Entity\TestEntity;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\Routing\Annotation\Route;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\Validator\Validator\ValidatorInterface;
class TestController extends AbstractController
{
/**
* @Route("/", name="test")
*/
public function test(Request $request, ValidatorInterface $validator)
{
$newEntity = new TestEntity();
$newEntity->setPosition('test');
// In addition $form->isValid() does this for you and displays form error specified in entity
if(($result = $validator->validate($newEntity))->count() === 0) {
$em = $this->getDoctrine()->getManager();
$em->persist($newEntity);
$em->flush();
dump('Entity persisted');
} else {
dump($result); // <- array of violations
dump('Sorry entity with this position exists');
}
die;
}
}
这是您的实体:
<?php
namespace App\Entity;
use Doctrine\ORM\Mapping as ORM;
use Symfony\Bridge\Doctrine\Validator\Constraints\UniqueEntity;
/**
* @ORM\Table(name="test_entity")
* @ORM\Entity(repositoryClass="App\Repository\TestEntityRepository")
*
* @UniqueEntity(
* fields={"position"},
* errorPath="position",
* message="Duplicate of position {{ value }}."
* )
*/
class TestEntity
{
/**
* @ORM\Column(name="id", type="integer")
* @ORM\Id
* @ORM\GeneratedValue(strategy="AUTO")
*/
private $id;
public function getId()
{
return $this->id;
}
/**
* @ORM\Column(name="position", type="string", length=190, unique=true) // key length limit for Mysql
*/
private $position;
public function getPosition()
{
return $this->position;
}
public function setPosition($position)
{
$this->position = $position;
return $this;
}
}
出于好奇,我测试了你的第一个想法,它似乎对我有用
<?php
namespace App\Controller;
use App\Entity\TestEntity;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\Routing\Annotation\Route;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\Validator\Validator\ValidatorInterface;
class TestController extends AbstractController
{
/**
* @Route("/", name="test")
*/
public function test(Request $request, ValidatorInterface $validator)
{
$newEntity = new TestEntity();
$newEntity->setPosition('test');
try {
$em = $this->getDoctrine()->getManager();
$em->persist($newEntity);
$em->flush();
} catch(\Doctrine\DBAL\Exception\UniqueConstraintViolationException $e) {
dump('First catch');
dump($e);
}
catch(\Exception $e) {
dump('Second catch');
dump($e);
}
die;
}
}
它导致“第一次捕获”的转储,但这可能与我使用的是mysql而不是SQlite有关,这也是对Symfony验证文档的引用,以防您想检查它
EDIT2
Simple Validator 类
class SomeSortOfValidator
{
public function exists($entity, $fieldName, $fieldValue)
{
// Lets suppose you have entity manager autowired.
$record = $this->em->getRepository($entity)->findOneBy([
$fieldName => $fieldValue
]);
return $record ? true : false;
}
}
- 1 回答
- 0 关注
- 128 浏览
添加回答
举报