1 回答
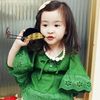
TA贡献1773条经验 获得超3个赞
首先,您需要确定文本文件的结构。这是你如何做到这一点。
示例文本文件:
3
%%%
@@@
&&&
What is Java?%%%a. Food@@@b. Programming Language@@@c. Person%%%b
Is this a question?%%%a. Yes@@@b. No@@@c. Maybe%%%a
Are you correct?%%%a. Yes@@@b. No@@@c. Maybe%%%c
是文件中
的问题数 是问题各部分的分隔符 是选项各
部分的分隔符 是答案
各部分的分隔符(如果存在具有多个答案的问题),
后续部分是问题。格式为:3%%%@@@&&&(question part)%%%(choice part)%%%(answer part)
由于有许多可能的选择,因此您可以像这样格式化可能的选项:这是来自上面的选项。(choice a)@@@(choice b)@@@(choice c)(choice part)
由于可能有很多可能的答案,因此您可以像这样格式化可能的答案:这是来自上面的答案。(answer a)@@@(answer b)@@@(answer c)(answer part)
一旦你决定了你的文本文件的外观,你可以创建一个类来保存这些问题,选择和答案。例如:
public class Question {
private String question; // This will hold the question
private String[] choices;// This will hold the choices
private String[] answers;// This will hold the correct answer/s, I made it an array since there might be
// a question that has multiple answers
public Question(String question, String[] choices, String[] answers) {
this.question = question;
this.choices = choices;
this.answers = answers;
}
/**
* @return the question
*/
public String getQuestion() {
return question;
}
/**
* @param question
* the question to set
*/
public void setQuestion(String question) {
this.question = question;
}
/**
* @return the choices
*/
public String[] getChoices() {
return choices;
}
/**
* @param choices
* the choices to set
*/
public void setChoices(String[] choices) {
this.choices = choices;
}
/**
* @return the answer
*/
public String[] getAnswers() {
return answers;
}
/**
* @param answer
* the answer to set
*/
public void setAnswer(String[] answer) {
this.answers = answer;
}
}
现在你有一个班级,可以容纳这些问题,选择和答案。然后,您将创建一个读者类,该类将读取所有这些信息并将其传输到您的问题类。下面是一个示例:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class TextFileStuff {
public static Question[] fileAsString(String path) {
try {
BufferedReader br = new BufferedReader(new FileReader(path));
String line = br.readLine(); // get the number of questions
Question[] questionList = new Question[Integer.parseInt(line)]; // initialize the question array
String partsDelimiter = br.readLine(); // get the delimiter for the question parts
String choicesDelimiter = br.readLine(); // get the delimiter for the choices
String answersDelimiter = br.readLine(); // get the delimiter for the answers
int questionNumber = 0; // initialize the question number
while ((line = br.readLine()) != null) { // loop through the file to get the questions
if (!line.trim().isEmpty()) {
String[] questionParts = line.split(partsDelimiter); // split the line to get the parts of the
// question
String question = questionParts[0]; // get the question
String[] choices = questionParts[1].split(choicesDelimiter); // get the choices
String[] answers = questionParts[2].split(answersDelimiter); // get the answers
Question q = new Question(question, choices, answers); // declare and initialize the question
questionList[questionNumber] = q; // add the question to the question list
questionNumber++; // increment the question number
}
}
br.close();
return questionList;
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
}
现在您已经拥有了所有必需的部分,剩下的就是制作主要方法并将这些问题呈现给用户。你可以这样做:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
String path = "\\Test\\src\\res\\questions.txt"; // the path of the text file
// that contains the
// questions
Question[] questionList = TextFileStuff.fileAsString(path);
takeTheQuiz(questionList);
}
public static void takeTheQuiz(Question[] questions) {
int score = 0;
Scanner userInput = new Scanner(System.in);
for (int i = 0; i < questions.length; i++) {
System.out.print("======================");
System.out.print("Question " + (i + 1));
System.out.println("======================");
System.out.println(questions[i].getQuestion()); // Print the question
String[] choices = questions[i].getChoices(); // get the choices
for (int j = 0; j < choices.length; j++) { // loop through the choices
System.out.println(choices[j]); // Print the choices
}
System.out.print("Input an answer : "); // Print the choices
String answer = userInput.nextLine().toLowerCase();
String[] answers = questions[i].getAnswers(); // get the answers
for (int j = 0; j < answers.length; j++) { // loop through the answers
if (answer.equals(answers[j])) { // check if the user's answer is correct
score++; // increment score
}
}
}
System.out.println("You scored " + score + " out of " + questions.length);
if (score == questions.length) {
System.out.println("Cheater!");
} else if (score <= 2) {
System.out.println("You suuuuck.");
} else {
System.out.println("Mediocre performance.");
}
userInput.close();
}
}
以下是该程序的示例输出:
======================Question 1======================
What is Java?
a. Food
b. Programming Language
c. Person
Input an answer : a
======================Question 2======================
Is this a question?
a. Yes
b. No
c. Maybe
Input an answer : v
======================Question 3======================
Are you correct?
a. Yes
b. No
c. Maybe
Input an answer : a
You scored 0 out of 3
You suuuuck.
现在你有一个有点动态的问答程序。如果要添加/删除问题,则只需修改文本文件即可。请随时发表评论以进行澄清和提出问题。
添加回答
举报