3 回答
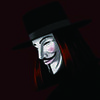
TA贡献1836条经验 获得超13个赞
您可以使用这样的组合列表和字典理解来做到这一点:
import json
from pprint import pprint
response = b'{"id":1005672,"messages":[{"id":4461048,"body":"Mnow test test","conversationId":1005672,"locationId":2045,"contactId":12792806,"assignedUserId":0,"status":"RECEIVED","error":null,"kind":"INCOMING","outgoing":false,"reviewRequest":false,"type":"SMS","readDate":0,"respondedDate":0,"sentDate":1576783232355,"attachments":[]},{"id":4461049,"body":"THIS NUMBER DOES NOT CURRENTLY ACCEPT TEXT MESSAGES PLEASE CALL (716) 444-4444 TO WORK WITH ONE OF OUR INTAKE SPECIALISTS","conversationId":1005672,"locationId":2045,"contactId":12792806,"assignedUserId":0,"status":"RECEIVED","error":null,"kind":"AUTO_RESPONSE","outgoing":true,"reviewRequest":false,"type":"SMS","readDate":0,"respondedDate":0,"sentDate":1576783233546,"attachments":[]},{"id":4620511,"body":"test sms,test sms","conversationId":1005672,"locationId":2045,"contactId":12792806,"assignedUserId":17297,"status":"DELIVERED","error":null,"kind":"API","outgoing":true,"reviewRequest":false,"type":"SMS","readDate":0,"respondedDate":0,"sentDate":1577987093930,"attachments":[]}]}'
data = json.loads(response)
messages = [
{'id': message['id'],
'conversationId': message['conversationId'],
'body': message['body']} for message in data['messages']
]
pprint(messages, sort_dicts=False)
输出:
[{'id': 4461048, 'conversationId': 1005672, 'body': 'Mnow test test'},
{'id': 4461049,
'conversationId': 1005672,
'body': 'THIS NUMBER DOES NOT CURRENTLY ACCEPT TEXT MESSAGES PLEASE CALL '
'(716) 444-4444 TO WORK WITH ONE OF OUR INTAKE SPECIALISTS'},
{'id': 4620511, 'conversationId': 1005672, 'body': 'test sms,test sms'}]
您可以使处理更加数据驱动,并消除理解中的大量重复编码,从而使其更加简洁,如下所示:
import json
from pprint import pprint
data_points = 'id', 'conversationId', 'body'
response = b'{"id":1005672,"messages":[{"id":4461048,"body":"Mnow test test","conversationId":1005672,"locationId":2045,"contactId":12792806,"assignedUserId":0,"status":"RECEIVED","error":null,"kind":"INCOMING","outgoing":false,"reviewRequest":false,"type":"SMS","readDate":0,"respondedDate":0,"sentDate":1576783232355,"attachments":[]},{"id":4461049,"body":"THIS NUMBER DOES NOT CURRENTLY ACCEPT TEXT MESSAGES PLEASE CALL (716) 444-4444 TO WORK WITH ONE OF OUR INTAKE SPECIALISTS","conversationId":1005672,"locationId":2045,"contactId":12792806,"assignedUserId":0,"status":"RECEIVED","error":null,"kind":"AUTO_RESPONSE","outgoing":true,"reviewRequest":false,"type":"SMS","readDate":0,"respondedDate":0,"sentDate":1576783233546,"attachments":[]},{"id":4620511,"body":"test sms,test sms","conversationId":1005672,"locationId":2045,"contactId":12792806,"assignedUserId":17297,"status":"DELIVERED","error":null,"kind":"API","outgoing":true,"reviewRequest":false,"type":"SMS","readDate":0,"respondedDate":0,"sentDate":1577987093930,"attachments":[]}]}'
data = json.loads(response)
messages = [{dp: message.get(dp) for dp in data_points}
for message in data['messages']]
pprint(messages, sort_dicts=False)
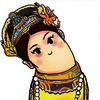
TA贡献1842条经验 获得超12个赞
我的理解是,您希望您的消息采用可通过对话检索的数据结构。这是我要做的:
from pprint import pprint
#with this data structure whenever we refer to a conversation, if it
#doesn't exist, it gets created
from collections import defaultdict
inbound_dict = defaultdict(dict)
inbound=json.loads(data)
for item in inbound['messages']:
print (item)
current_conversation=inbound_dict[item['conversationId']]
#inbound_dict retrives the apropriate conversation,
# or creates a new one for us to fill
current_conversation[item["id"]] = item['body'] #add our item to it.
#or if there's a chance we might want *everything* else about the
#message later even if just the date in order to preserve
#conversation ordering or whatever:
#currentconversation[item["id"]] = item
pprint(inbound_dict)
但这可能是矫枉过正,这取决于你以后要做什么处理,以及它是什么处理。如果您只是让他们选择一个对话,并显示最后 20 条消息,那么可切片的列表可能是内部数据结构的最佳选择,在这种情况下,我会这样做:
from pprint import pprint
#with this data structure whenever we refer to a conversation, if it
#doesn't exist, it gets created
from collections import defaultdict
inbound_dict = defaultdict(list)
inbound=json.loads(data)
for item in inbound['messages']:
print (item)
current_conversation=inbound_dict[item['conversationId']]
#inbound_dict retrives the apropriate conversation,
# or creates a new one for us to fill
current_conversation.append(
(item["id"], item['body'])
) # here we add our item to it, in this case a tuple of id and body
#or if there's a chance we might want *everything* else about the
#message later even if just the date in order to preserve
#conversation ordering or whatever:
#currentconversation.append(item)
pprint(inbound_dict)
基本相同的操作,但 defaultdict 为我们提供了不同类型的内部集合来填充。
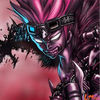
TA贡献1794条经验 获得超8个赞
如果您可以拥有多个具有相同会话 ID 的项目,那么您可以执行以下操作:
r=requests.get(url + id, headers=h, params=p).json()
inbound_dict = {}
for item in r['messages']:
conv_id = item['conversationId']
if conv_id not in inbound_dict:
inbound_dict[conv_id]=[{'msg_id' : item['id'], 'body' : item['body']}]
else:
inbound_dict[conv_id].append({'msg_id' : item['id'], 'body' : item['body']})
print(inbound_dict)
生成的数据结构是一个以 conversation_id 作为键的字典,每个 conversation_id 映射到一个list项目dict。每个项目都存储特定消息的 message_id 和正文。然后,您可以通过检索为 conv_id 键存储的消息列表来迭代特定对话的消息。
或者,您可以选择以下数据结构进行映射: {conv_id -> { message_id : {message info ...}, ...}.
这可以像这样实现:
r=requests.get(url + id, headers=h, params=p).json()
inbound_dict = {}
for item in r['messages']:
conv_id = item['conversationId']
if conv_id not in inbound_dict:
inbound_dict[conv_id]={item['id'] : {'msg_id' : item['id'], 'body' : item['body']}}
else:
inbound_dict[conv_id][item['id']] = {'msg_id' : item['id'], 'body' : item['body']}
print(inbound_dict)
在这种情况下,如果您知道 con_id 和 message_id,则可以直接从对话中访问消息。
所以它真的取决于这个数据结构的下游实用程序。
请注意,上述内容也可以通过列表推导来完成。
添加回答
举报