4 回答
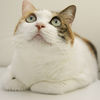
TA贡献1798条经验 获得超3个赞
您可以使用 safenet SDK 开发您的加密功能,这些功能可以与 Java 中的 HSM 进行交互。例如:Gemalto HSM 为 Java 开发人员提供 JSP 和 JCProv API 作为 SDK 的一部分。
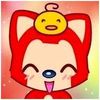
TA贡献1858条经验 获得超8个赞
以下命令显示如何向 Thales HSM 发送命令。
import java.io.ByteArrayOutputStream;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
import java.net.SocketTimeoutException;
import java.net.UnknownHostException;
public class ThalesHSMConnect2 {
//@formatter:off
public static final String send(final String command) throws UnknownHostException, IOException {
try(final Socket sc = new Socket(host, port);
final DataInputStream din = new DataInputStream(sc.getInputStream());
final DataOutputStream dos = new DataOutputStream(sc.getOutputStream())) {
sc.setSoTimeout(5000);
dos.writeUTF(command);
dos.flush();
final String response = din.readUTF();
return response;
}
}
public static final byte[] send(final byte[] command) throws Exception {
try(Socket sc = new Socket(host, port);
InputStream in = sc.getInputStream();
OutputStream os = sc.getOutputStream()) {
sc.setSoTimeout(5000);
command[0] = (byte) ((command.length-2)/256); //two byte command length
command[1] = (byte) ((command.length-2)%256); //two byte command length
os.write(command);
os.flush();
final byte b1 = (byte) in.read();
final byte b2 = (byte) in.read();
if(b1 < 0 || b2 < 0) throw new SocketTimeoutException("no response from hsm.");
final byte[] response = new byte[b1*256+b2];
in.read(response);
return response;
}
}
public static void main(String[] args) throws IOException {
final String cvvGenerationResponse = send("0000CWAAAAAAAAAAAAAAAABBBBBBBBBBBBBBBB4484070020000310;2105000");
}
}
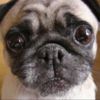
TA贡献1735条经验 获得超5个赞
以下代码显示了如何准备并向 safenet HSM 发送命令。
public static final String send(String command) {
try (Socket socket = new Socket(HSMIP, HSMPORT);
InputStream in = socket.getInputStream();
OutputStream os = socket.getOutputStream()) {
byte[] commandbytes = DatatypeConverter.parseHexBinary(command);
byte[] request = new byte[6 + commandbytes.length];
request[0] = 0x01; //constant as per setting during installation
request[1] = 0x01; //constant as per setting during installation
request[2] = 0x00; //constant as per setting during installation
request[3] = 0x00; //constant as per setting during installation
request[4] = (byte) (commandbytes.length / 256); //length of command
request[5] = (byte) (commandbytes.length % 256); //length of command
System.arraycopy(commandbytes, 0, request, 6, commandbytes.length);
//logger.info("request : " + DatatypeConverter.printHexBinary(request));
os.write(request);
os.flush();
byte[] header = new byte[6];
in.read(header);
logger.info("header : " + DatatypeConverter.printHexBinary(header));
int len = (header[4] & 0xFF) * 256 + (header[5] & 0xFF); //length of response
logger.info("len : " + len);
byte[] response = new byte[len];
in.read(response);
logger.info("response : " + DatatypeConverter.printHexBinary(response));
return DatatypeConverter.printHexBinary(response);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
添加回答
举报