3 回答
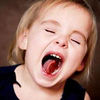
TA贡献1777条经验 获得超10个赞
# Error: RuntimeError: file <maya console> line 1: An invalid path was specified.
运行时会触发此错误,cmds.file(rename=your_path)但提供的路径的目录不存在,这是有道理的,因为它无效!
所以你所要做的就是在调用它之前创建文件夹。你可以使用os.makedirs它。您不想在完整路径中包含文件名,因此您也可以使用os.path.dirname将其剥离。所以不是传递它"/my/full/path/file_name.mb",而是使用os.path.dirname将它剥离到"/my/full/path".
因此,扩展 itypewithmyhands 的答案将如下所示:
import os
newVersion = getNextVersion()
versionFolder = os.path.dirname(newVersion)
if not os.path.exists(versionFolder):
os.makedirs(versionFolder)
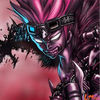
TA贡献1803条经验 获得超6个赞
下面的方法非常冗长,因为我使用的是字符串操作而不是正则表达式。这希望它对你来说更具可读性,但我建议只要你觉得足够舒服就改用正则表达式。
请注意,处理字符串格式以重命名场景的函数将使用最少 2 位数字(01、02 等)和最多 3 位数字(100、101 等)。如果超过 999 次迭代,则需要稍作修改。
注意: 此方法依赖于文件版本之前的下划线(例如 _01.mb),而不是当前的字符串索引方法。
import maya.cmds as cmds
def getNextVersion():
curName = cmds.file(query=True, expandName=True) # eg. D:/yourScene_01.mb
curVersionFull = curName.split('_')[-1] # eg. 01.mb
versionExtension = curVersionFull.split('.') # eg. ['01', 'mb']
curVersionNum = int(versionExtension[0]) # eg. 1
extension = versionExtension[1] # eg. 'mb'
nextVersionNum = curVersionNum + 1 # increment your version
if nextVersionNum < 100:
nextVersionFull = '{:02d}.{}'.format(nextVersionNum, extension) # eg. 02.mb
else:
nextVersionFull = '{:03d}.{}'.format(nextVersionNum, extension) # eg. 100.mb
newName = curName.replace(curVersionFull, nextVersionFull)
return newName
cmds.file(rename=getNextVersion())
cmds.file(save=True)
更新:
您已经接受了另一个答案,我相信您问题的核心是@green-cell 所描述的。至于这怎么可能,我真的不知道。Maya 通常不会让您保存到不存在的文件夹中。
例如:
import maya.cmds as cmds
cmds.file(rename="D:/doesntexist/somefile.mb")
# Error: An invalid path was specified. : D:/doesntexist/
# Traceback (most recent call last):
# File "<maya console>", line 2, in <module>
# RuntimeError: An invalid path was specified. : D:/doesntexist/ #
无论如何,我在这里为您整理了一个更全面的功能。请注意,很难满足所有可能的文件名模式,特别是如果允许用户在过程的任何部分指定自己的文件名。这次我使用regular expressions,这使您的替换更加健壮(尽管远非万无一失)。
import re
import os
import maya.cmds as cmds
def getNextVersion(createDir=True):
'''Find next available filename iteration based on current scene name
Args:
createDir (bool, optional): True to create the output directory if required. False to leave as-is (may trigger a failure)
Returns:
str|None: Full file path with incremented version number. None on failure
'''
# Grab current filename. This always returns something. If unsaved, it will return something unsavory, but still a string
curFile = cmds.file(query=True, expandName=True)
print('Current filename is {}'.format(curFile))
# This matches a digit between 1 and 4 numbers in length, followed immediately by a file extension between 2 and 3 characters long
m = re.match(r'(.+?)(\d{1,4})\.(\w{2,3})', curFile)
if m == None:
print('Failed at regex execution. Filename does not match our desired pattern')
return None
# Extract regex matches
basePath = m.group(1)
version = m.group(2)
extension = m.group(3)
# Failsafe. Should not trigger
if not basePath or not version or not extension:
print('Failed at failsafe. Filename does not match our desired pattern')
return None
# Increment file version
numDigits = len(version)
newFile = None
try:
version = int(version) + 1
# Deal with padding
newLength = len(str(version))
if newLength > numDigits:
numDigits = newLength
# Compile new path
newFile = '{base}{ver:{numDig:02d}d}.{ext}'.format(base=basePath, ver=version, numDig=numDigits, ext=extension)
except Exception as e:
print('Error parsing new version for path {}: {}'.format(curFile, e))
return None
# Another failsafe
if not newFile:
print('Failed at failsafe. Filename calculations succeeded, but new path is not valid')
return None
# Create output dir if needed
dirname = os.path.dirname(newFile)
if createDir and not os.path.isdir(dirname):
try:
os.makedirs(dirname)
print('Created all dirs required to build path {}'.format(dirname))
except Exception as e:
print('Error creating path {}: {}'.format(dirname, e))
return None
return newFile
nextVersion = getNextVersion()
if not nextVersion:
cmds.error('Error parsing new filename increment. Please see console for more information')
else:
cmds.file(rename=nextVersion)
cmds.file(save=True)
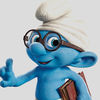
TA贡献1946条经验 获得超3个赞
更新#02:
我意识到错误只发生在第一个版本中。假设我有第一个版本为 sceneName_01.ma 并尝试对其进行版本升级并保存,Maya 会告诉我“指定了无效路径”。但是如果我手动将其重命名为sceneName_02.ma并再次重新运行代码,代码将正常工作。
这不是因为版本的数量。一旦我尝试将我的第一个版本保存为 sceneName_00.ma 并尝试脚本,它仍然给了我同样的错误。我不得不手动将其重命名为 sceneName_01.ma 并重新运行脚本,直到它工作为止。
添加回答
举报