1 回答
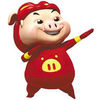
TA贡献2080条经验 获得超4个赞
当透视投影对称时,给定(y轴)视场角FOV、纵横比aspectRatio以及到近平面(NEAR_PLANE)和远平面(FAR_PLANE)的距离,然后视锥体的8个角点在视图中空间可以计算:
float aspectRatio = (float)Display.getWidth() / (float)Display.getHeight();
float y_scale = 1.0f / (float)Math.tan( Math.toRadians(FOV / 2.0) );
float x_scale = y_scale * aspectRatio;
float near_x = NEAR_PLANE * x_scale;
float near_y = NEAR_PLANE * y_scale;
float far_x = FAR_PLANE * x_scale;
float far_y = FAR_PLANE * y_scale;
Vector3f left_bottom_near = new Vector3f(-near_x, -near_y, FAR_PLANE);
Vector3f right_bottom_near = new Vector3f( near_x, -near_y, FAR_PLANE);
Vector3f left_top_near = new Vector3f(-near_x, near_y, FAR_PLANE);
Vector3f right_top_near = new Vector3f( near_x, near_y, FAR_PLANE);
Vector3f left_bottom_far = new Vector3f(-far_x, -far_y, FAR_PLANE);
Vector3f right_bottom_far = new Vector3f( far_x, -far_y, FAR_PLANE);
Vector3f left_top_far = new Vector3f(-far_x, far_y, FAR_PLANE);
Vector3f right_top_far = new Vector3f( far_x, far_y, FAR_PLANE);
如果您想知道世界空间中视锥体的 8 个角点,则必须将这些点从视图空间转换到世界空间。
从世界空间转换到视图空间的矩阵,就是“视图”矩阵。可以从视图空间转换到世界空间的矩阵是逆视图矩阵(invert())。
逆视图矩阵是由视图位置、视图方向和视图的向上向量定义的矩阵。Matrix4f可以设置如下:
Vector3f eyePos; // position of the view (eye position)
Vector3f targetPos; // the point which is looked at
Vector3f upVec; // up vector of the view
Vector3f zAxis = Vector3f.sub(eyePos, targetPos, null);
zAxis.normalise();
Vector3f xAxis = Vector3f.cross(upVec, zAxis, null);
xAxis.normalise();
Vector3f yAxis = Vector3f.cross(zAxis, upVec, null);
Matrix4f inverseView = new Matrix4f();
inverseView.m00 = xAxis.x; inverseView.m01 = xAxis.y; inverseView.m02 = xAxis.z; inverseView.m03 = 0.0f;
inverseView.m10 = yAxis.x; inverseView.m11 = yAxis.y; inverseView.m12 = yAxis.z; inverseView.m13 = 0.0f;
inverseView.m20 = zAxis.x; inverseView.m21 = zAxis.y; inverseView.m22 = zAxis.z; inverseView.m23 = 0.0f;
inverseView.m30 = eyePos.x; inverseView.m31 = eyePos.y; inverseView.m32 = eyePos.z; inverseView.m33 = 1.0f;
注意,视图空间的坐标系是右手系,其中X轴指向左侧,Y轴指向上方,然后Z轴指向视图外(注意在右手系中Z 轴是 X 轴和 Y 轴的叉积)。
最后角点可以通过矩阵变换:
例如
Vector4f left_bottom_near_v4 = new Vector4f(
left_bottom_near.x, left_bottom_near.y, left_bottom_near.z, 1.0f
);
Vector4f left_bottom_near_world_v4 = new Vector4f();
Matrix4f.transform(Matrix4f left, left_bottom_near_v4, left_bottom_near_world_v4);
Vector3f left_bottom_near_world = new Vector3f(
left_bottom_near_world_v4 .x, left_bottom_near_world_v4 .y, left_bottom_near_world_v4 .z
);
添加回答
举报