1 回答
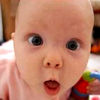
TA贡献1808条经验 获得超4个赞
正如评论中提到的@fabian - 您应该首先解析文件内容,修改并覆盖文件。这是一个示例代码如何实现这一点:
首先,我不知道您使用的是什么 json 库,但我强烈建议使用以下内容:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.8</version>
</dependency>
它通常会简化您使用 json 的工作。如果您不想使用库,您仍然可以按照说明进行操作,但可以根据您的需要进行调整。整个实现是这样的:
public class JSONWriteExample {
private static final String FILE_NAME = "jsonArray.json";
private static final Path FILE_PATH = Paths.get(FILE_NAME);
private final String type;
private final int quantity;
public JSONWriteExample(String type, int quantity) {
this.type = type;
this.quantity = quantity;
}
public void jsonParse() throws IOException {
ObjectMapper objectMapper = new ObjectMapper();
if (Files.notExists(FILE_PATH)) {
Files.createFile(FILE_PATH);
objectMapper.writeValue(FILE_PATH.toFile(), createItems(new ArrayList<>()));
}
Items items = objectMapper.readValue(FILE_PATH.toFile(), Items.class);
final List<Item> itemsList = items.getItems();
objectMapper.writeValue(FILE_PATH.toFile(), createItems(itemsList));
}
private Items createItems(List<Item> itemsList) {
final Item item = new Item();
item.setType(type);
item.setQuantity(quantity);
itemsList.add(item);
final Items items = new Items();
items.setItems(itemsList);
return items;
}
public static class Items {
private List<Item> items;
// Setters, Getters
}
public static class Item {
private String type;
private int quantity;
// Setters, Getters
}
}
好的,这段代码发生了什么?
首先,请注意 Java 7 NIO 的用法——推荐的在 java 中处理文件的方法。
在
jsonParse
方法中,我们首先检查文件是否存在。如果是 - 然后我们将其读取到
Items
描述我们模型的数据类 ( ) 中。阅读部分是在这个库的底层完成的,只是你的 json 文件的文件应该与数据类的字段同名(或用JsonAlias
annotation.xml 指定)。如果没有 - 那么我们首先创建它并填充初始值。
ObjectMapper
是库中的类,用于读取\写入 json 文件。
现在,如果我们运行这段代码,例如
public static void main(String[] args) throws IOException {
JSONWriteExample example = new JSONWriteExample("TV", 3);
example.jsonParse();
JSONWriteExample example2 = new JSONWriteExample("phone", 3);
example2.jsonParse();
}
json 文件将如下所示:
{
"items": [
{
"type": "TV",
"quantity": 3
},
{
"type": "TV",
"quantity": 3
},
{
"type": "phone",
"quantity": 3
}
]
}
添加回答
举报