4 回答
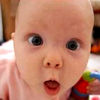
TA贡献1830条经验 获得超3个赞
第一个条件是将单个值与列表进行比较。放下它,然后改用:
for w in listA:
if melt == w[1] and boil == w[2]:
print("Is", w[0], "your chosen element?")
break
而不是第二个条件(也有错误的比较),您可以else在循环中添加一个子句(不要识别这个else词,因为它属于第一个for而不是属于if):
else:
for x in listB:
print(x[0])
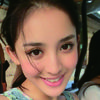
TA贡献1880条经验 获得超4个赞
如果您输入时melt用户给出一个数字,例如 1541,那么该变量melt将包含一个整数 1541。但是,您listA的元素包含 3 个项目的列表、一个字符串和 2 个整数。
因此,当您测试 if meltis in thelistA时,它什么也找不到,因为比较1541to["scandium", 1541, 2830]将返回 False,因为两者都不相等。
此外,使用列表来存储元素及其沸点并不理想。很难访问例如元素沸点,因为它嵌套在列表中。字典实现,或者更好的数据帧实现(cf pandas)会更有效率。
回到你的代码,这里有一个快速修复:
for w in listA:
if melt == w[1] and boil == w[2]:
print("Is", w[0], "your chosen element?")
最后,您还必须处理输入错误检查以确认用户正在输入有效数据。
编辑:字典
使用字典,您可以将键链接到值。键和值可以是任何东西:数字、列表、对象、字典。唯一的条件是密钥必须具有hash()定义的方法。
例如,您可以使用字符串作为键存储元素,将 2 个温度作为值存储。
elements = {"scandium": (1541, 2830), "titanium": (1668, 3287)}
要访问一个元素,您调用elements["scandium"]which 将返回 (1541, 2830)。您还可以使用 3 个函数访问字典的元素:
elements.keys(): 返回键
elements.values(): 返回值
elements.items():返回元组(键,值)
然而,再次单独访问温度并不容易。但是这次你可以这样比较:
for key, value in elements.items():
if (melt, boil) == value:
print (key)
但更好的是,由于您正在从熔化和沸腾温度中查找元素,您可以创建以下字典,其中键是元组(沸腾,熔化),值是相应的元素。
elements = {(1541, 2830): "scandium", (1668, 3287): "titanium"}
然后,一旦用户输入melt和boil,您就可以找到对应的元素:elements[(melt, boil)]。这一次,不需要使用循环。可以直接访问相应的元素。可以使用 pandas 数据框完成类似的实现。
最后,您还可以使用一个类来实现它。
class Element:
def __init__(self, name, melt, boil):
self.name = name
self.melt = melt
self.boil = boil
elements = [Element("scandium", 1541, 2830), Element("titanium", 1668, 3287)]
事实上,Element该类不能用作字典的键。要将其用作键,hash必须定义方法。
class Element:
def __init__(self, name, melt, boil):
self.name = name
self.melt = melt
self.boil = boil
def __key(self):
return (self.name, self.melt, self.boil)
def __hash__(self):
return hash(self.__key())
melt但同样,从和boil变量访问元素并不是那么简单。再次需要循环。
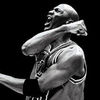
TA贡献1847条经验 获得超11个赞
为了确保您的输入确实是数字,您可以这样做:
# Check input validity.
while True:
try:
melt = int(input("What is the melting point of your chosen element (in degrees Celcius): "))
boil = int(input("What is the boiling point of your chosen element (in degrees Celcius): "))
break
except ValueError:
continue
# Rest of the work.
...
基本上,在一个循环中,您要求用户输入。如果int()成功,由于break关键字而退出循环,您可以放心地假设melt和boil在程序的其余部分中是整数。如果int()失败,它会引发 aValueError并且continue关键字只是确保我们再次循环。
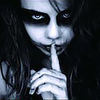
TA贡献1802条经验 获得超6个赞
见下文(请注意,代码使用namedtuple以提高可读性)
from collections import namedtuple
ElementProperties = namedtuple('ElementProperties', 'name melting_point boiling_point')
elements = [ElementProperties("scandium", 1541, 2830),
ElementProperties("titanium", 1668, 3287),
ElementProperties("vanadium", 1910, 3407),
ElementProperties("chromium", 1907, 2671),
ElementProperties("manganese", 1246, 2061),
ElementProperties("iron", 1538, 2862)]
melt = int(input("What is the melting point of your chosen element (in degrees Celcius): "))
boil = int(input("What is the boiling point of your chosen element (in degrees Celcius): "))
for element in elements:
if element.melting_point == melt and element.boiling_point == boil:
print('Your element is: {}'.format(element.name))
break
else:
print('Could not find element having melting point {} and boilong point {}'.format(melt, boil))
如果元素在几个列表中,下面的代码应该可以工作:
from collections import namedtuple
ElementProperties = namedtuple('ElementProperties', 'name melting_point boiling_point')
elements1 = [ElementProperties("scandium", 1541, 2830),
ElementProperties("titanium", 1668, 3287),
ElementProperties("vanadium", 1910, 3407),
ElementProperties("chromium", 1907, 2671),
ElementProperties("manganese", 1246, 2061),
ElementProperties("iron", 1538, 2862)]
elements2 = [ElementProperties("hydrogen", 5, 9)]
melt = int(input("What is the melting point of your chosen element (in degrees Celcius): "))
boil = int(input("What is the boiling point of your chosen element (in degrees Celcius): "))
found = False
for element_lst in [elements1,elements2]:
if found:
break
for element in element_lst:
if element.melting_point == melt and element.boiling_point == boil:
print('Your element is: {}'.format(element.name))
found = True
break
if not found:
print('Could not find element having melting point {} and boilong point {}'.format(melt, boil))
添加回答
举报