2 回答
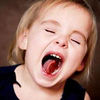
TA贡献1777条经验 获得超10个赞
这样做,代码对它们有注释,您可以阅读并适应您的需求。
package main
import "database/sql"
import "fmt"
import _ "github.com/go-sql-driver/mysql"
type trade_history struct {
// best practice in golang is to name your field in upper camel case if you intend to export it
// or lower camel case if you don't.
id, final_meta_report_id, trading_account_id, lp, lp2, lp3 int
// `type` is reserved keyword, therefore for example, i use `ptype`
symbol, price, price_type, time, ptype, status, created_at, updated_at string
qty, pegged_distance, price_limit double
}
// you don't need to declare global variable like this, if you want it to be accessible to all your packages
// it's best to use struct.
// var db *sql.DB
// var err error -- this one is shadowed by declaration of err in your getTradingHistory anyway.
func getTradingHistory(reportId int) (err error) {
// Your dsn is unquoted hence error
db, err := sql.Open("mysql", "username:password@trade.asdewx134.us-east-2.rds.amazonaws.com:3306/trading_dashboard")
// your trade data is here
defer db.Close()
if err != nil {
fmt.Println(err.Error())
}
err = db.Ping()
if err != nil {
fmt.Println(err.Error())
}
var th trade_history
// this one is also unquoted and your SQL statement is not correct
// here i add `FROM [YOUR TABLE] that you can edit to your needs`
err = db.QueryRow("select id, final_meta_report_id, trading_account_id, symbol, qty, price, price_type, time, lp, lp2, lp3, pegged_distance, price_limit, time_limit, type, status, created_at FROM [YOUR TABLE] WHERE id = ?", reportId).Scan( /* Scan your fields here */ ) // This is where you should scan your fields.
if err != nil {
fmt.Println(err.Error())
}
fmt.Printf("id: %d\n Final_meta_report_id: %d\n trading_account_id: %d\n symbol: %s\n qty: %.2f\n price: %s\n price_type: %s\n time: %s\n lp: %d\n lp2: %d\n lp3: %d\n pegged_distance: %.2f\n pr$")
return err
}
func main() {
getTradingHistory(2074)
}
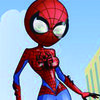
TA贡献1807条经验 获得超9个赞
您应该修复错误,查看代码注释如下:
package main
import "database/sql"
import "fmt"
import _ "github.com/go-sql-driver/mysql"
type trade_history struct {
id, final_meta_report_id, trading_account_id, lp, lp2, lp3 int
symbol, price, price_type, time, typeField, status, created_at, updated_at string
qty, pegged_distance, price_limit double
}
var db *sql.DB
var err error
func getTradingHistory(final_Meta_report_ID int) (err error) {
host := "trade.asdewx134.us-east-2.rds.amazonaws.com"
port := 3306
db := "trading_dashboard"
//use Sprintf if you wanna parse a string with values
conn := fmt.Sprintf("%s:%s@%s:%s/%s", username, password, host, port, db)
db, err = sql.Open("mysql", conn)
defer db.Close()
if err != nil {
fmt.Println(err.Error())
}
err = db.Ping()
if err != nil {
fmt.Println(err.Error())
}
var p trade_history
//if you have multiple lines of texts use ` instead of "
err = db.QueryRow(`select id, final_meta_report_id,
trading_account_id, symbol, qty, price, price_type, time, lp,
lp2, lp3, pegged_distance, price_limit, time_limit, type, status, created_at`).Scan(&p)
if err != nil {
fmt.Println(err.Error())
}
fmt.Printf("id: %d\n Final_meta_report_id: %d\n trading_account_id: %d\n symbol: %s\n qty: %.2f\n price: %s\n price_type: %s\n time: %s\n lp: %d\n lp2: %d\n lp3: %d\n pegged_distance: %.2f\n")
return err
}
func main() {
getTradingHistory(2074)
}
- 2 回答
- 0 关注
- 266 浏览
添加回答
举报