2 回答
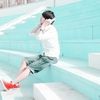
TA贡献1966条经验 获得超4个赞
RegEx.Split是你的朋友,这很好用。
void Main()
{
// fun fact, the @ in front of the string means it's literal, so you
// literally get the new lines
var input =
@"id score ping guid name lastmsg address qport rate
-- - -------------------------------------------------------------------------
1 11 45 176387877 Player 1 3250 101.102.103.104:555 3647 25000
2 23 61 425716719 Player 2 3250 105.106.107.108:555 5978 25000";
//Gets you each line
var lines = input.Split('\n');
// Skip 2 because I doubt you care about the column title
// or the row with the dashes
foreach (var line in lines.Skip(2))
{
// For each line, Regex split will return an array with each entry
// Set a breakpoint with the debugger and inspect to see what I mean.
// Splits using regex - assumes at least 2 spaces between items
// so space in 'Player 1' is handled it's a fickle solution though
// Trim the line before RegEx split to avoid extra data in the split
var r = Regex.Split(line.Trim(), @"\s{2,}");
}
}
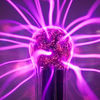
TA贡献1886条经验 获得超2个赞
您可以使用 Regex 和命名组来执行此操作。
样本输入
var str = @"id score ping guid name lastmsg address qport rate
--- ----- ---- ---------- --------------- ------- --------------------- ------ -----
1 11 45 176387877 Player 1 3250 101.102.103.104:555 3647 25000
2 23 61 425716719 Player 2 3250 105.106.107.108:555 5978 25000";
正则表达式定义
var regex = new Regex(@"^(?<id>[\d]+)(\s{2,})(?<score>[\d]+)(\s{2,})(?<ping>[\d]+)(\s{1,})(?<guid>[\d]+)(\s{2,})(?<name>([\w]+\s[\w]+))(\s{2,})(?<lastmsg>[\d]+)(\s{2,})(?<ip>[\d.:]+)(\s{2,})(?<port>[\d]+)(\s{2,})(?<rate>[\d]+)$",RegexOptions.Compiled);
解析代码
var lines = str.Split(new []{Environment.NewLine},StringSplitOptions.RemoveEmptyEntries);
foreach(var line in lines)
{
var match = regex.Match(line.Trim());
if(!match.Success) continue;
Console.WriteLine($"ID = {match.Groups["id"].Value}");
Console.WriteLine($"Score = {match.Groups["score"].Value}");
Console.WriteLine($"Ping = {match.Groups["ping"].Value}");
Console.WriteLine($"Guid = {match.Groups["guid"].Value}");
Console.WriteLine($"Name = {match.Groups["name"].Value}");
Console.WriteLine($"Last Msg = {match.Groups["lastmsg"].Value}");
Console.WriteLine($"Port = {match.Groups["port"].Value}");
Console.WriteLine($"Rate = {match.Groups["rate"].Value}");
}
输出
ID = 1
Score = 11
Ping = 45
Guid = 176387877
Name = Player 1
Last Msg = 3250
Port = 3647
Rate = 25000
ID = 2
Score = 23
Ping = 61
Guid = 425716719
Name = Player 2
Last Msg = 3250
Port = 5978
Rate = 25000
- 2 回答
- 0 关注
- 209 浏览
添加回答
举报