2 回答
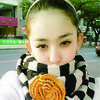
TA贡献1786条经验 获得超11个赞
您可能需要以下内容:
function validateKeys(object, expectedKeys) {
let keys = Object.keys(object);
// Check if both arrays have the same length
// if not, we can exit early
if (keys.length !== expectedKeys.length) {
return false;
}
// If they do have the same length, then let's see if they
// have all the keys, if not, return false
for (let index = 0; index < expectedKeys.length; index++) {
if (!expectedKeys.includes(keys[index])) {
return false;
};
}
// else return true, the keys are valid
return true;
}
//running the function with `objectA` and `expectedKeys`
// should return `true`
const objectA = {
id: 2,
name: "Jane Doe",
age: 34,
city: "Chicago",
};
// running the function with `objectB` and `expectedKeys`
// should return `false`
const objectB = {
id: 3,
age: 33,
city: "Peoria",
};
const expectedKeys = ["id", "name", "age", "city"];
function validateKeys(object, expectedKeys) {
let keys = Object.keys(object);
// Check if both arrays have the same length
// if not, we can exit early
if (keys.length !== expectedKeys.length) {
return false;
}
// If they do have the same length, then let's see if they
// have all the keys, if not, return false
for (let index = 0; index < expectedKeys.length; index++) {
if (!expectedKeys.includes(keys[index])) {
return false;
};
}
// else return true, the keys are valid
return true;
}
/* From here down, you are not expected to
understand.... for now :)
Nothing to see here!
*/
function testIt() {
const objectA = {
id: 2,
name: "Jane Doe",
age: 34,
city: "Chicago",
};
const objectB = {
id: 3,
age: 33,
city: "Peoria",
};
const objectC = {
id: 9,
name: "Billy Bear",
age: 62,
city: "Milwaukee",
status: "paused",
};
const objectD = {
foo: 2,
bar: "Jane Doe",
bizz: 34,
bang: "Chicago",
};
const expectedKeys = ["id", "name", "age", "city"];
if (typeof validateKeys(objectA, expectedKeys) !== "boolean") {
console.error("FAILURE: validateKeys should return a boolean value");
return;
}
if (!validateKeys(objectA, expectedKeys)) {
console.error(
`FAILURE: running validateKeys with the following object and keys
should return true but returned false:
Object: ${JSON.stringify(objectA)}
Expected keys: ${expectedKeys}`
);
return;
}
if (validateKeys(objectB, expectedKeys)) {
console.error(
`FAILURE: running validateKeys with the following object and keys
should return false but returned true:
Object: ${JSON.stringify(objectB)}
Expected keys: ${expectedKeys}`
);
return;
}
if (validateKeys(objectC, expectedKeys)) {
console.error(
`FAILURE: running validateKeys with the following object and keys
should return false but returned true:
Object: ${JSON.stringify(objectC)}
Expected keys: ${expectedKeys}`
);
return;
}
if (validateKeys(objectD, expectedKeys)) {
console.error(
`FAILURE: running validateKeys with the following object and keys
should return false but returned true:
Object: ${JSON.stringify(objectD)}
Expected keys: ${expectedKeys}`
);
return;
}
console.log("SUCCESS: validateKeys is working");
}
testIt();
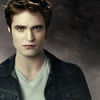
TA贡献1830条经验 获得超9个赞
您正在将string(expectedKeys[i]) 与 number(length)进行比较。Javascript不会给你一个错误,但它总是会评估为假。此外,您在 for 循环中放置了一个 return,当遇到它时会中断循环。
添加回答
举报