4 回答
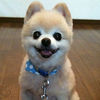
TA贡献1806条经验 获得超8个赞
最简单的方法是首先获取所有元素,然后过滤掉不需要的元素:
const elems = [...document.querySelectorAll('h2,h4')]
.filter( (elem) => !elem.matches('.docs-body__heading *') );
console.log(elems);
<div class="docs-body__heading">
<h2>do not select me</h2>
<div><h4>me neither</h4></div>
</div>
<div>
<h2>select me</h2>
<div><h4>and me</h4></div>
</div>
最快的(就性能而言)可能是使用TreeWalker:
const walker = document.createTreeWalker(document.body, NodeFilter.SHOW_ELEMENT, {
acceptNode(elem) {
return elem.matches('.docs-body__heading') ?
NodeFilter.FILTER_REJECT : NodeFilter.FILTER_ACCEPT;
}
}, true);
const elems = [];
while (walker.nextNode()) {
const tagname = walker.currentNode.tagName;
if (tagname === 'H2' || tagname === 'H4') {
elems.push(walker.currentNode);
}
}
console.log(elems);
<div class="docs-body__heading">
<h2>do not select me</h2>
<div>
<h4>me neither</h4>
</div>
</div>
<div>
<h2>select me</h2>
<div>
<h4>and me</h4>
</div>
</div>
但是,如果您不是在具有数百万个 DOM 节点的千米长文档中执行此操作,那么第一个版本应该足够了。
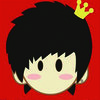
TA贡献1898条经验 获得超8个赞
这一行将满足您的所有需求!
document.querySelectorAll("*:not(.docs-body__heading) > h1, *:not(.docs-body__heading) > h2, *:not(.docs-body__heading) > h4");
更容易阅读:
let not = "*:not(.docs-body__heading)";
document.querySelectorAll(`${not} > h1, ${not} > h2, ${not} > h4`);
使用样品:
window.addEventListener('load', () => {
var elems = document.querySelectorAll("*:not(.docs-body__heading) > h1, *:not(.docs-body__heading) > h2, *:not(.docs-body__heading) > h4");
for(var i = 0 ; i < elems.length ; i++) {
elems[i].style.backgroundColor = "red";
}
});
<html>
<head>Selector</head>
<body>
<h1>1</h1>
<h2>2</h2>
<h4>3</h4>
<div class="docs-body__heading">
<h1>1</h1>
<h2>2</h2>
<h4>3</h4>
</div>
</body>
</html>
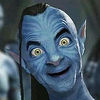
TA贡献1843条经验 获得超7个赞
我认为只有当 h2/h4 是docs-body__heading
const x = document.querySelectorAll(":not(.docs-body__heading) > h2,h4");
console.log(x);
<div class="docs-body__heading">
<h2>A</h2>
</div>
<h4>B</h1>
<h2>C</h1>
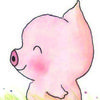
TA贡献1900条经验 获得超5个赞
现在您正在排除具有docs-body__heading
该类的元素,以排除其中使用的元素:
document.querySelectorAll(`:not(.docs-body__heading > *) h2, h2, h4`);
添加回答
举报