2 回答
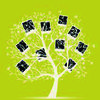
TA贡献1873条经验 获得超9个赞
您可以从Jackson
库中使用JsonTypeInfo
和JsonSubTypes
注释。它们是句柄多态类型处理:
@JsonTypeInfo
用于指示序列化中包含哪些类型信息的详细信息@JsonSubTypes
用于表示注解类型的子类型@JsonTypeName
用于定义用于注释类的逻辑类型名称
您的示例适合此解决方案,除了看起来更像简单POJO
类的根对象。在您的情况下,我们应该创建有助于使用以下 3 种类型的类型结构:string
, date
, map
:
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, property = "type")
@JsonSubTypes({
@JsonSubTypes.Type(value = StringValue.class, name = "string"),
@JsonSubTypes.Type(value = DateValue.class, name = "date"),
@JsonSubTypes.Type(value = MapValue.class, name = "map")
})
abstract class HasValue<T> {
protected T value;
public HasValue() {
this(null);
}
public HasValue(T value) {
this.value = value;
}
public T getValue() {
return value;
}
public void setValue(T value) {
this.value = value;
}
@Override
public String toString() {
return getClass().getSimpleName() + "{" +
"value=" + value +
"}";
}
}
class StringValue extends HasValue<String> {
public StringValue() {
this(null);
}
public StringValue(String value) {
super(value);
}
}
class DateValue extends HasValue<String> {
public DateValue(String value) {
super(value);
}
public DateValue() {
this(null);
}
}
class MapValue extends HasValue<Map<String, HasValue>> {
public MapValue(Map<String, HasValue> value) {
super(value);
}
public MapValue() {
this(new LinkedHashMap<>());
}
public void put(String key, HasValue hasValue) {
this.value.put(key, hasValue);
}
}
现在,我们需要引入POJO根值。它可能如下所示,但您可以根据需要添加 getter/setter。对于这个例子,下面的代码就足够了:
class Root {
public HasValue firstObject;
public HasValue secondObject;
public HasValue thirdObject;
public HasValue fourthObject;
@Override
public String toString() {
return "Root{" +
"firstObject=" + firstObject +
", secondObject=" + secondObject +
", thirdObject=" + thirdObject +
", fourthObject=" + fourthObject +
'}';
}
}
现在,我们终于可以尝试序列化和反序列化这些对象了:
MapValue customerName = new MapValue();
customerName.put("customerName", new StringValue("customerValue"));
MapValue innerMap = new MapValue();
innerMap.put("anotherObj1", new StringValue("TEST"));
innerMap.put("anotherObj2", new DateValue("01/12/2018"));
innerMap.put("anotherObj3", new DateValue("31/01/2018"));
MapValue fourthObject = new MapValue();
fourthObject.put("firstObject", innerMap);
Root root = new Root();
root.firstObject = new StringValue("productValue");
root.secondObject = new StringValue("statusValue");
root.thirdObject = customerName;
root.fourthObject = fourthObject;
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writerWithDefaultPrettyPrinter().writeValueAsString(root);
System.out.println(json);
System.out.println(mapper.readValue(json, Root.class));
Aboce 代码打印JSON:
{
"firstObject" : {
"type" : "string",
"value" : "productValue"
},
"secondObject" : {
"type" : "string",
"value" : "statusValue"
},
"thirdObject" : {
"type" : "map",
"value" : {
"customerName" : {
"type" : "string",
"value" : "customerValue"
}
}
},
"fourthObject" : {
"type" : "map",
"value" : {
"firstObject" : {
"type" : "map",
"value" : {
"anotherObj1" : {
"type" : "string",
"value" : "TEST"
},
"anotherObj2" : {
"type" : "date",
"value" : "01/12/2018"
},
"anotherObj3" : {
"type" : "date",
"value" : "31/01/2018"
}
}
}
}
}
}
和toString表示:
Root{firstObject=StringValue{value=productValue}, secondObject=StringValue{value=statusValue}, thirdObject=MapValue{value={customerName=StringValue{value=customerValue}}}, fourthObject=MapValue{value={firstObject=MapValue{value={anotherObj1=StringValue{value=TEST}, anotherObj2=DateValue{value=01/12/2018}, anotherObj3=DateValue{value=31/01/2018}}}}}}
您可以通过添加/删除任何类型的HasValue实例轻松地操作输出。
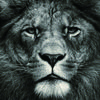
TA贡献1834条经验 获得超8个赞
从这里下载 JSON jar 。从客户端使用 json.stringify 将您的 JSON 转换为字符串(因为 JSONObject 的构造函数只接受字符串)。收到客户端的请求后,执行以下操作:
public void doPost(request,response) throws ParseException, JSONException {
parseMapFromJSON(request.getParameter("JSONFromClient"));
}
private void parseMapFromJSON(String JSONParam) throws JSONException
{
JSONObject requestJSON = new JSONObject(JSONParam);
for(int i=0; i<requestJSON.length();i++)
{
String key = (String) requestJSON.names().get(i);
if(key.endsWith("Object"))
{
parseMapFromJSON(requestJSON.get(key).toString());
}
else if(key.startsWith("type") && (requestJSON.get(key).equals("date") || requestJSON.get(key).equals("string")))
{
System.out.println(requestJSON.get("value"));
break;
}
else if(key.startsWith("type") && requestJSON.get(key).equals("map"))
{
parseMapFromJSON(requestJSON.get("value").toString());
}
else if(!key.equals("value"))
{
parseMapFromJSON(requestJSON.get(key).toString());
}
}
}
添加回答
举报