3 回答
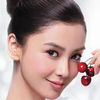
TA贡献1853条经验 获得超6个赞
如果我们假设它DistGroup基于hashCode/equalsand size,color你可以这样做:
bumperCars
.stream()
.map(x -> {
List<String> list = new ArrayList<>();
list.add(x.getCarCode());
return new SimpleEntry<>(x, list);
})
.map(x -> new DistGroup(x.getKey().getSize(), x.getKey().getColor(), x.getValue()))
.collect(Collectors.toMap(
Function.identity(),
Function.identity(),
(left, right) -> {
left.getCarCodes().addAll(right.getCarCodes());
return left;
}))
.values(); // Collection<DistGroup>
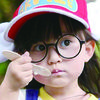
TA贡献1815条经验 获得超13个赞
解决方案-1
只需将两个步骤合并为一个:
List<DistGroup> distGroups = bumperCars.stream()
.collect(Collectors.groupingBy(t -> new SizeColorCombination(t.getSize(), t.getColor())))
.entrySet().stream()
.map(t -> {
DistGroup d = new DistGroup(t.getKey().getSize(), t.getKey().getColor());
d.addCarCodes(t.getValue().stream().map(BumperCar::getCarCode).collect(Collectors.toList()));
return d;
})
.collect(Collectors.toList());
解决方案-2
groupingBy如果您可以使用属性两次并将值映射为List代码,那么您的中间变量会好得多,例如:
Map<Integer, Map<String, List<String>>> sizeGroupedData = bumperCars.stream()
.collect(Collectors.groupingBy(BumperCar::getSize,
Collectors.groupingBy(BumperCar::getColor,
Collectors.mapping(BumperCar::getCarCode, Collectors.toList()))));
并简单地使用forEach添加到最终列表中:
List<DistGroup> distGroups = new ArrayList<>();
sizeGroupedData.forEach((size, colorGrouped) ->
colorGrouped.forEach((color, carCodes) -> distGroups.add(new DistGroup(size, color, carCodes))));
注意:我已经更新了您的构造函数,使其接受卡代码列表。
DistGroup(int size, String color, List<String> carCodes) {
this.size = size;
this.color = color;
addCarCodes(carCodes);
}
进一步将第二个解决方案组合成一个完整的陈述(尽管我自己喜欢forEach老实说):
List<DistGroup> distGroups = bumperCars.stream()
.collect(Collectors.groupingBy(BumperCar::getSize,
Collectors.groupingBy(BumperCar::getColor,
Collectors.mapping(BumperCar::getCarCode, Collectors.toList()))))
.entrySet()
.stream()
.flatMap(a -> a.getValue().entrySet()
.stream().map(b -> new DistGroup(a.getKey(), b.getKey(), b.getValue())))
.collect(Collectors.toList());
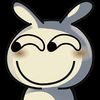
TA贡献1817条经验 获得超14个赞
查看我的图书馆AbacusUtil:
StreamEx.of(bumperCars) .groupBy(c -> Tuple.of(c.getSize(), c.getColor()), BumperCar::getCarCode) .map(e -> new DistGroup(e.getKey()._1, e.getKey()._2, e.getValue()) .toList();
添加回答
举报