3 回答
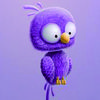
TA贡献1796条经验 获得超4个赞
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.util.Random;
public class testWord {
String[] article={"the", "a", "one", "some", "any"};
String[] noun={"boy", "girl", "dog", "town", "car"};
String[] verb={"drove", "jumped", "ran", "walk", "skipped"};
String[] preposition={"to", "from", "over", "under", "on"};
Frame frame=new Frame("test");
TextArea ta=new TextArea();
testWord(){
ta.setSize(350, 250);
frame.setSize(400,300);
frame.add(ta);
frame.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
private int[] getSerial(){
int[] result=new int[4];
Random r=new Random();
for(int i=0;i<4;i++)
result[i]=r.nextInt(5);
return result;
}
private String comb(String a, String n, String v, String p){
return a.substring(0,1).toUpperCase()+
a.substring(1,a.length())+" "+n+" "+v+" "+p+".";
}
public String getSentence(){
int[] serial=getSerial();
return comb(article[serial[0]], noun[serial[1]],
verb[serial[2]], preposition[serial[3]]);
}
private void output(){
String tmp="";
for(int i=0;i<20;i++) tmp=tmp+getSentence()+"\n";
ta.setText(tmp);
frame.setVisible(true);
}
public static void main(String[] args) {
testWord tw=new testWord();
tw.output();
}
}
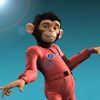
TA贡献1845条经验 获得超8个赞
import javax.swing.SwingUtilities;
import javax.swing.JPanel;
import javax.swing.JFrame;
import javax.swing.JLabel;
import java.awt.Rectangle;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import javax.swing.SwingConstants;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JButton;
public class HelpTest extends JFrame {
private static final long serialVersionUID = 1L;
/**
* 下面的几个数组是用来保存各个单词的,可以自己添加其他的
*/
private String[] article = {"the","a","one","some","any"};
private String[] noun = {"boy ","girl","dog","town","car"};
private String[] verb = {"drove","jumped","ran","walk","skipped"};
private String[] preposition = {"to","from","over","under","on"};
private String sentence = "",word = null;//用来保存生成的句子 // @jve:decl-index=0:
private JPanel jContentPane = null;
private JLabel jLabel = null;
private JScrollPane jScrollPane = null;
private JTextArea jTextArea = null;
private JButton jButton = null;
private List addToList(){
List list = new ArrayList();
list.add(0, article);
list.add(1, noun);
list.add(2, verb);
list.add(3, preposition);
return list;
}
private String getSentence(){//获取句子
List list = addToList();
Iterator it = list.iterator();
sentence = "";
for(int i=0;i<4;i++){
sentence = sentence + getWord((String[])it.next())+" ";
}
String cha = ""+sentence.charAt(0);
sentence = sentence.substring(1);
sentence = cha.toUpperCase()+sentence;
return sentence;
}
private String getWord(String[] words){
int len = words.length;
Random r = new Random();
int num = r.nextInt(len);
word = words[num];
return word;
}
private JScrollPane getJScrollPane() {
if (jScrollPane == null) {
jScrollPane = new JScrollPane();
jScrollPane.setBounds(new Rectangle(34, 57, 453, 123));
jScrollPane.setViewportView(getJTextArea());
}
return jScrollPane;
}
private JTextArea getJTextArea() {
if (jTextArea == null) {
jTextArea = new JTextArea();
jTextArea.setLineWrap(true);
jTextArea.setText("");
}
return jTextArea;
}
private JButton getJButton() {
if (jButton == null) {
jButton = new JButton();
jButton.setBounds(new Rectangle(229, 198, 62, 21));
jButton.setText("确定");
jButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent e) {
for(int i=0;i<20;i++){
jTextArea.append(getSentence()+"\n");
}
}
});
}
return jButton;
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
HelpTest thisClass = new HelpTest();
thisClass.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
thisClass.setVisible(true);
}
});
}
public HelpTest() {
super();
initialize();
}
private void initialize() {
this.setBounds(250, 200, 540, 275);
this.setContentPane(getJContentPane());
this.setTitle("句子生成器");
this.setResizable(false);
}
private JPanel getJContentPane() {
if (jContentPane == null) {
jLabel = new JLabel();
jLabel.setBounds(new Rectangle(145, 22, 224, 26));
jLabel.setHorizontalAlignment(SwingConstants.CENTER);
jLabel.setText("点击下面的按钮生成20句简单的话");
jContentPane = new JPanel();
jContentPane.setLayout(null);
jContentPane.add(jLabel, null);
jContentPane.add(getJScrollPane(), null);
jContentPane.add(getJButton(), null);
}
return jContentPane;
}
}
是想要这种结果的吗?我用的是Eclipse。不过在命令行下运行也是通过的。
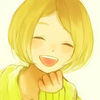
TA贡献1820条经验 获得超9个赞
好像挺简单的...
import java.awt.*;
import javax.swing.*;
public class RandomSentence extends JFrame {
private String[] article = { "the", "a", "one", "some", "any" };
private String[] noun = { "boy", "girl", "dog", "town", "car" };
private String[] verb ={"drove", "jumped", "ran", "walk", "skipped" };
private String[] preposition = { "to", "from", "over","under","on" };
private JTextArea ta;
public RandomSentence() {
super("随机创建句子");
ta = new JTextArea();
getContentPane().setLayout(new BorderLayout());
getContentPane().add("Center", new JScrollPane(ta));
setSize(300, 300);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void sentence() {
for (int i = 0; i < 20; i++) {
String s1 = article[(int) (Math.random() * article.length)];
String s11 = s1.substring(0, 1).toUpperCase().concat(s1.substring(1));
String s2 = noun[(int) (Math.random() * noun.length)];
String s3 = verb[(int) (Math.random() * verb.length)];
String s4 = preposition[(int) (Math.random() * preposition.length)];
StringBuffer s =
new StringBuffer(s11 + " " + s2 + " " + s3 + " "+ s4 + ".");
String str = s.toString();
ta.append(str + "\n");
}
}
public static void main(String[] args) {
new RandomSentence().sentence();
}
}
添加回答
举报