2 回答
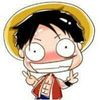
TA贡献1829条经验 获得超4个赞
//LStack.h
//堆栈的链式存储结构
#include<malloc.h>
#include<process.h>
//定义结点结构体
typedef struct StackNode
{
ElemType data; //数据域
struct StackNode *next; //指针域
}StackNode;
//定义栈顶指针
typedef struct
{
StackNode *top; //栈顶指示器
}LinkStack;
//初始化
void InitStack(LinkStack &S)
{
if(NULL==(S.top=(StackNode *)malloc(sizeof(StackNode)))) //申请栈顶结点空间
exit(0); //申请失败推出程序
S.top->next=NULL; //申请成功栈顶结点指针域初始化为空
S.top->data=NULL; //数据域初始化为NULL
}
//判断栈是否为空
int isEmpty(LinkStack &S)
{
if(NULL==S.top->next&&NULL==S.top->data) //栈顶没有后继结点表明为空栈
return 0; //返回0
return 1; //非空时返回1
}
//入栈
int Push(const ElemType &item,LinkStack &S) //此处最好把item定义为抽象数据类型ElemType
{
StackNode *snp;
if(NULL==(snp=(StackNode *)malloc(sizeof(StackNode)))) //申请新结点空间
return 0;
snp->data=item;
snp->next=S.top;
S.top=snp;
return 1;
}
//出栈
int Pop(LinkStack &S)
{
ElemType reData;
StackNode *indexp;
if(!isEmpty(S)) //栈空退出
return 0;
reData=S.top->data; //取出数据域存储的数据
indexp=S.top; //保存当前栈顶结点
S.top=S.top->next; //栈顶变为top的后继结点
free(indexp); //释放原栈顶结点空间
return reData; //返回栈顶数据
}
//取栈顶数据
int GetTop(LinkStack &S)
{
ElemType reData;
if(!isEmpty(S)) //栈空退出
return 0;
reData=S.top->data; //取出数据
return reData;
}
//清空栈
void MakeEmpty(LinkStack &S)
{
StackNode *p,*pp;
p=S.top;
while(p!=NULL)
{
pp=p;
p=p->next;
free(pp);
}
}
LStack.cpp
#include<stdio.h>
typedef int ElemType;
#include "LStack.h"
int main() //测试堆栈的链式存储结构
{
LinkStack T;
int a[10]={1,3,5,7,9,11,13,15,17,19};
InitStack(T); //初始化栈
for(int i=0;i<10;i++)
Push(a[i],T); // 入栈
printf("栈顶数据=%d\n",GetTop(T)); //取栈顶数据
printf("出栈:");
while(isEmpty(T)) //栈不空时继续出栈
{
printf("%-4d",Pop(T)); //出栈
}
MakeEmpty(T); //清空栈
}
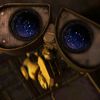
TA贡献2021条经验 获得超8个赞
/// 按照你的要求写出来了,并且改了两个你的错误,,
/// 给分吧,,呵呵,祝你学习愉快
// 刚刚把2个C++的内存分配改成c的了
#include <stdio.h>
#include <malloc.h>
#define ElemType int
typedef struct StackNode
{
ElemType data; //存放数据
struct StackNode * next; //指向下一个结点
}StackNode;
typedef struct LinkStack
{
struct StackNode * top; // 栈顶指针
}LinkStack;
void InitStack(LinkStack &S); // 栈的初始化
void Push(const int &item,LinkStack &S); // 入栈
int Pop(LinkStack &S); // 出栈
int GetTop(LinkStack &S); // 取栈顶元素
int IsEmpty(LinkStack &S); // 判断栈是否为空
void MakeEmpty(LinkStack &S); // 清空栈
// 打印堆栈内容
void PrintStack(LinkStack &S)
{
StackNode *pSN = S.top;
while (pSN->next != 0)
{
printf("%d " , pSN->data);
pSN = pSN->next;
}
printf("\n");
}
void main()
{
LinkStack Stack; // 栈顶
int iItem = 0 ,i = 0;
/// 初始化
InitStack(Stack);
// 压入内容
for (i = 0 ; i < 10 ; i ++)
{
Push(i , Stack);
}
printf("栈内内容为:");
PrintStack(Stack);
// 弹出
iItem = Pop(Stack);
printf("\n弹出%d:" , iItem);
printf("\n栈内内容为:");
PrintStack(Stack);
// 弹出
iItem = Pop(Stack);
printf("\n弹出%d:" , iItem);
printf("\n栈内内容为:");
PrintStack(Stack);
//清空栈
MakeEmpty(Stack);
}
// 栈的初始化
void InitStack(LinkStack &S)
{
S.top = (struct StackNode*)malloc(sizeof(StackNode));
S.top->data = 0;
S.top->next = 0;
}
// 入栈
void Push(const int &item , LinkStack &S)
{
StackNode *pSN = (struct StackNode*)malloc(sizeof(StackNode));
pSN->data = item;
pSN->next = S.top;
S.top = pSN;
}
// 出栈
int Pop(LinkStack &S)
{
if (IsEmpty(S))
{
return 0;
}
StackNode *pSN = S.top;
int iRet = pSN->data;
S.top = pSN->next;
delete pSN;
return iRet;
}
// 取栈顶元素
int GetTop(LinkStack &S)
{
if (IsEmpty(S))
{
return 0;
}
return S.top->data;
}
// 判断栈是否为空
int IsEmpty(LinkStack &S)
{
StackNode *pSN = S.top->next;
if (0 == pSN->next)
{
return 1;
}
return 0;
}
// 清空栈
void MakeEmpty(LinkStack &S)
{
while (!IsEmpty(S))
{
Pop(S);
}
if (S.top != 0)
{
delete S.top;
}
S.top = 0;
}
添加回答
举报