3 回答
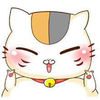
TA贡献1864条经验 获得超2个赞
先把它分组怎么样:
public string checkIfConnditions(string msg, int score, int age)
{
var response = "";
if (msg == "hello") {
response = score > 20 ?
age > 25 ? "Age not satisfied" : "All para success"
: age < 25 ? "Score not satisfied" : "Age & Score not satisfied";
} else {
if (score > 20)
{
response = age < 25 ? "Unmatching message" : "Unmatiching message & Age not satisfied" ;
} else {
response = age < 25 ? "Unmatiching message & Score not satisfied " : "All parameter unsatisfied" ;
}
}
return response;
}
如果相等,您还需要注意条件。例如
if (msg == "hello" && score > 20 && age < 25)
{
response = "All para success";
}
//and ...
if (msg == "hello" && score < 20 && age < 25)
{
response = "Score not satisfied";
}
// what if score == 20 ?
withif else声明,或者The conditional operator (?:)我们可以避免这种情况
更新
if(msg == "hello")
{
if(score < 20)
{
response = age > 25 ? "Age & Score not satisfied" : "Score not satisfied";
} else {
response = age > 25 ? "Age not satisfied" : "All para success";
}
} else {
if(score < 20)
{
response = age > 25 ? "All parameter unsatisfied" : "Unmatiching message & Score not satisfied ";
} else {
response = age > 25 ? "Unmatiching message & Age not satisfied" : "Unmatching message";
}
}
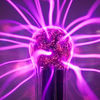
TA贡献1886条经验 获得超2个赞
List<String> Errors = new List<String>();
int chk = 3;
if ( msg != "hello" )
{
Errors.Add( "Unmatching message" );
}
if ( score < 20 )
{
Errors.Add( "Score not satisfied" );
}
if ( age > 25 )
{
Errors.Add( "Age not satisfied" );
}
if ( Errors.Count == 0 )
{
return "All para success";
}
else if ( Errors.Count == 3)
{
return "All parameter unsatisfied";
}
else
{
return String.Join( " & ", Errors );
}
** 代码已编辑,因为我将 String.Join 输入错误为 String.Format **
或者,如果您想逐个回答,也可以使用字节
int flag = 0x0;
if ( msg == "hello" )
{
flag |= 0x1;
}
if ( score > 20 )
{
flag |= 0x2;
}
if ( age < 25 )
{
flag |= 0x4;
}
switch ( flag )
{
case 0x7:
response = "All para success";
break;
case 0x6:
response = "Unmatching message";
break;
case 0x5:
response = "Score not satisfied";
break;
case 0x4:
response = "Unmatiching message & Age not satisfied";
break;
case 0x3:
response = "Score not satisfied";
break;
case 0x2:
response = "Unmatiching message & Score not satisfied ";
break;
case 0x1:
response = "Score not satisfied & Age not satisfied";
break;
default:
response = "All parameter unsatisfied";
break;
}
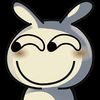
TA贡献2037条经验 获得超6个赞
一种可能的方法是创建字典,其中包含您的真值表和相应的响应,例如:
private readonly Dictionary<(bool isMsgValid, bool isScoreValid, bool isAgeValid), string> _responses
= new Dictionary<(bool, bool, bool), string>()
{
[(true, true, true)] = "All para success",
[(false, false, false)] = "All parameter unsatisfied",
[(false, true, true)] = "Unmatching message",
[(true, false, true)] = "Score not satisfied",
[(true, true, false)] = "Age not satisfied",
[(false, false, true)] = "Unmatiching message & Score not satisfied",
[(false, true, false)] = "Unmatiching message & Age not satisfied",
[(true, false, false)] = "Age & Score not satisfied"
};
public string checkIfConnditions(string msg, int score, int age)
=> _responses[(msg == "hello", score > 20, age < 25)];
由您决定哪个变体更优雅,这只是可能的解决方案之一。
请注意,这里使用C# 7.0 features, ValueTuple 字典键和表达式主体方法checkIfConnditions。
编辑
这是我用于测试的示例:
public static class Program
{
private static readonly Dictionary<(bool isMsgValid, bool isScoreValid, bool isAgeValid), string> _responses
= new Dictionary<(bool, bool, bool), string>()
{
[(true, true, true)] = "All para success",
[(false, false, false)] = "All parameter unsatisfied",
[(false, true, true)] = "Unmatching message",
[(true, false, true)] = "Score not satisfied",
[(true, true, false)] = "Age not satisfied",
[(false, false, true)] = "Unmatiching message & Score not satisfied",
[(false, true, false)] = "Unmatiching message & Age not satisfied",
[(true, false, false)] = "Age & Score not satisfied"
};
public static string checkIfConnditions(string msg, int score, int age)
=> _responses[(msg == "hello", score > 20, age < 25)];
public static void Main(string[] args)
{
Console.WriteLine(checkIfConnditions("hello", 45, 20));
Console.WriteLine(checkIfConnditions("hello", 45, 30));
Console.WriteLine(checkIfConnditions("hello", 10, 20));
Console.WriteLine(checkIfConnditions("hello", 10, 30));
Console.WriteLine(checkIfConnditions("goodbye", 45, 20));
Console.WriteLine(checkIfConnditions("goodbye", 10, 30));
Console.WriteLine(checkIfConnditions("goodbye", 45, 20));
Console.WriteLine(checkIfConnditions("goodbye", 10, 30));
}
}
请注意,在这种情况下,_responsesandcheckIfConnditions必须是静态的。
- 3 回答
- 0 关注
- 122 浏览
添加回答
举报