3 回答
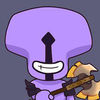
TA贡献1909条经验 获得超7个赞
使用类而不是 ID,例如,而不是
echo "<div id='rallyPrintJan'>";
利用
echo "<div class='rallyPrint'>";
然后,当单击日历的容器时,遍历每个.rallyPrint类(使用事件委托而不是内联处理程序 - 内联处理程序是非常糟糕的做法,应尽可能避免使用)。检查被点击的元素是否有months祖先,如果有,确定months其父容器中的索引。rallyPrint从该索引中,您可以确定需要显示哪个元素:
document.querySelector('.calander-container').addEventListener('click', (e) => {
const months = e.target.closest('.months');
if (!months) {
return;
}
const thisIndex = [...months.parentElement.children].indexOf(months);
const rallyPrint = document.querySelectorAll('.rallyPrint');
rallyPrint.forEach((div) => {
div.style.display = 'none';
});
rallyPrint[thisIndex].style.display = 'block';
});
现场演示:
document.querySelector('.calander-container').addEventListener('click', (e) => {
const months = e.target.closest('.months');
if (!months) {
return;
}
const thisIndex = [...months.parentElement.children].indexOf(months);
const rallyPrint = document.querySelectorAll('.rallyPrint');
rallyPrint.forEach((div) => {
div.style.display = 'none';
});
rallyPrint[thisIndex].style.display = 'block';
});
<div class="calander-container">
<div class="months">
<p class="months-text">January</p>
</div>
<div class="months">
<p class="months-text">February</p>
</div>
<div class="months">
<p class="months-text">March</p>
</div>
<div class="months">
<p class="months-text">April</p>
</div>
<div class="months">
<p class="months-text">May</p>
</div>
<div class="months">
<p class="months-text">June</p>
</div>
<div class="months">
<p class="months-text">July</p>
</div>
<div class="months">
<p class="months-text">August</p>
</div>
<div class="months">
<p class="months-text">September</p>
</div>
<div class="months">
<p class="months-text">October</p>
</div>
<div class="months">
<p class="months-text">November</p>
</div>
<div class="months">
<p class="months-text">December</p>
</div>
</div>
<div class='rallyPrint'>1</div>
<div class='rallyPrint'>2</div>
<div class='rallyPrint'>3</div>
<div class='rallyPrint'>4</div>
<div class='rallyPrint'>5</div>
<div class='rallyPrint'>6</div>
<div class='rallyPrint'>7</div>
<div class='rallyPrint'>8</div>
<div class='rallyPrint'>9</div>
<div class='rallyPrint'>10</div>
<div class='rallyPrint'>11</div>
<div class='rallyPrint'>12</div>
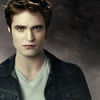
TA贡献1830条经验 获得超9个赞
创建一个包含所有月份的数组,然后遍历每个月份并将函数参数与每个月份的名称进行比较。如果它们相同,则显示元素。否则,隐藏元素。
function showMonth(monthName) {
const months = ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"];
months.forEach(month => {
const el = document.getElementById("rallyPrint" + month);
if (monthName === month) {
el.style.display = "block"; // Show current month
} else {
el.style.display = "none"; // Not the current month, hide
}
});
}
showMonth("Jan") // Only shows January
<div id="rallyPrintJan">Jan</div>
<div id="rallyPrintFeb">Feb</div>
<div id="rallyPrintMar">Mar</div>
<div id="rallyPrintApr">Apr</div>
<div id="rallyPrintMay">May</div>
<div id="rallyPrintJun">Jun</div>
<div id="rallyPrintJul">Jul</div>
<div id="rallyPrintAug">Aug</div>
<div id="rallyPrintSep">Sep</div>
<div id="rallyPrintOct">Oct</div>
<div id="rallyPrintNov">Nov</div>
<div id="rallyPrintDec">Dec</div>
现在您可以将showMonth("Jan")函数传递给onclickPHP 中的属性。
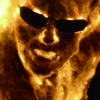
TA贡献1876条经验 获得超6个赞
这与@CertainPerformance 答案有关。
<html>
<head>
<style>
ul {list-style-type: none;}
body {font-family: Verdana, sans-serif;}
/* Month header */
.month {
padding: 70px 25px;
width: 100%;
background: #1abc9c;
text-align: center;
}
/* Month list */
.month ul {
margin: 0;
padding: 0;
}
.month ul li {
color: white;
font-size: 20px;
text-transform: uppercase;
letter-spacing: 3px;
}
/* Previous button inside month header */
.month .prev {
float: left;
padding-top: 10px;
}
/* Next button */
.month .next {
float: right;
padding-top: 10px;
}
/* Weekdays (Mon-Sun) */
.weekdays {
margin: 0;
padding: 10px 0;
background-color:#ddd;
}
.weekdays li {
display: inline-block;
width: 13.6%;
color: #666;
text-align: center;
}
/* Days (1-31) */
.days {
padding: 10px 0;
background: #eee;
margin: 0;
}
.days li {
list-style-type: none;
display: inline-block;
width: 13.6%;
text-align: center;
margin-bottom: 5px;
font-size:12px;
color: #777;
}
/* Highlight the "current" day */
.days li .active {
padding: 5px;
background: #1abc9c;
color: white !important
}
</style>
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script type="text/javascript">
$( document ).ready(function() {
document.querySelector('.calander-container').addEventListener('click', (e) => {
const months = e.target.closest('.months');
if (!months) {
return;
}
const thisIndex = [...months.parentElement.children].indexOf(months);
const rallyPrint = document.querySelectorAll('.rallyPrint');
rallyPrint.forEach((div) => {
div.style.display = 'none';
});
rallyPrint[thisIndex].style.display = 'block';
});
});
</script>
</head>
<body>
<div class="calander-container">
<?php
$month=array(
"1"=>"Jan",
"2"=>"Feb",
"3"=>"Mar",
"4"=>"April",
"5"=>"May",
"6"=>"June",
"7"=>"July",
"8"=>"Aug",
"9"=>"Sep",
"10"=>"Oct",
"11"=>"Nov",
"12"=>"Dec"
);
foreach($month as $k=>$v){
//echo "<br>Key :: ".$k." Val :: ".$v;
echo '<div class="months">
<p class="months-text">'.$v.'</p>
</div>';
}
?>
<?php
foreach($month as $k=>$v){
echo "<div class='rallyPrint'>$k</div>";
}
?>
</div>
</body>
添加回答
举报