3 回答
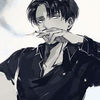
TA贡献1828条经验 获得超3个赞
这是一个正确执行您想要的程序的程序。它甚至可以确保您没有重复的乐透号码。
void Main()
{
const int count = 10;
const int max = 25;
//an array named "input" to hold the users' 10 guesses
int[] inputs = new int[count];
//a for loop to loop over the inputs array. each loop will ask the user for a number
Console.WriteLine("Enter your {0} lottery numbers one at a time. The numbers must be between 1 and {1}.", count, max);
for (int i = 0; i < inputs.Length; i++)
{
inputs[i] = Convert.ToInt32(Console.ReadLine());
}
//a random number generator
Random ranNum = new Random();
//an array named "allNums" to hold all the random numbers
int[] allNums = new int[max];
for (int i = 0; i < allNums.Length; i++)
{
allNums[i] = i + 1;
}
//shuffle
for (int i = 0; i < allNums.Length; i++)
{
int j = ranNum.Next(0, allNums.Length);
int temporary = allNums[j];
allNums[j] = allNums[i];
allNums[i] = temporary;
}
//an array named "lotNum" to hold 10 random numbers
int[] lotNums = new int[count];
Array.Copy(allNums, lotNums, lotNums.Length);
//writes out the randomly generated lotto numbers
Console.Write("\nThe lottery numbers are: ");
for (int i = 0; i < lotNums.Length; i++)
{
Console.Write("{0} ", lotNums[i]);
}
int correct = 0;
Console.Write("\nThe correct numbers are: ");
for (int i = 0; i < lotNums.Length; i++)
{
for (int j = 0; j < inputs.Length; j++)
{
if (lotNums[i] == inputs[j])
{
Console.Write("{0} ", lotNums[i]);
correct++;
};
}
}
Console.Write("\nYou got {0} correct. ", correct);
Console.WriteLine("\n\nPress any key to end the program:");
Console.ReadLine();
}
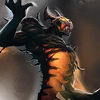
TA贡献1829条经验 获得超6个赞
你走对了。
我的实现将是:
foreach (var input in inputs)
{
for (int i = 0; i < lotNums.Length; i++){
if(input == lotNums[i]){
Console.WriteLine(lotNums[i]);
}
}
}
这会将输入数组的每个数字与彩票数组进行比较。我正在打印每个匹配项,但是如果找到匹配项,您可以将变量设置为 True,或者如果需要,可以将每个匹配的数字添加到数组中。
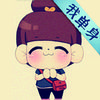
TA贡献1772条经验 获得超5个赞
您必须计算两个序列的交集。你有三个选择:
双 foreach 循环。这是需要避免的,因为它的时间复杂度为 O(m*n)。对于 10 个项目来说这不是问题,但我们应该制作可扩展的程序。
使用哈希连接。您可以为此使用 HashSet,这将是我的首选方法。但由于它本质上意味着使用
Contains
,因此这里不是选项。合并排序的序列。这将是去这里的方式。
该程序是不言自明的,它产生并交叉两个随机序列。
static Random rnd = new Random((int)DateTime.Now.Ticks);
static int[] GetRandomArray(int arrSize, int minNumber, int maxNumber)
{
int[] tmpArr = new int[maxNumber - minNumber + 1];
for (int i = 0; i < tmpArr.Length; ++i)
{
tmpArr[i] = i + minNumber; // fill with 1, 2, 3, 4,...
}
int[] ret = new int[arrSize];
for (int i = 0; i < ret.Length; ++i)
{
int index = rnd.Next(tmpArr.Length - i); //choose random position
ret[i] = tmpArr[index];
tmpArr[index] = tmpArr[tmpArr.Length - 1 - i]; //fill last of the sequence into used position
}
return ret;
}
static IEnumerable<int> GetMatches(int[] a, int[] b)
{
Array.Sort(a);
Array.Sort(b);
for (int i = 0, j = 0; i < a.Length && j < b.Length;)
{
if (a[i] == b[j])
{
yield return a[i];
++i;
++j;
}
else if (a[i] > b[j])
{
++j;
}
else
{
++i;
}
}
}
static void Main(string[] args)
{
var a = GetRandomArray(5, 3, 7);
var b = GetRandomArray(10, 1, 25);
Console.WriteLine("A: " + string.Join(", ", a));
Console.WriteLine("B: " + string.Join(", ", b));
Console.WriteLine("Matches: " + string.Join(", ", GetMatches(a, b)));
Console.ReadKey();
}
结果是这样的:
A: 7, 4, 6, 3, 5
B: 17, 1, 8, 14, 11, 22, 3, 20, 4, 25
Matches: 3, 4
您可以考虑如果其中一个或两个序列包含重复项会发生什么。
- 3 回答
- 0 关注
- 141 浏览
添加回答
举报