3 回答
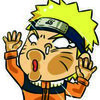
TA贡献1936条经验 获得超6个赞
我尝试使用几种方法来实现您的需求。
这是我的示例代码。
from azure.storage.blob.baseblobservice import BaseBlobService
import numpy as np
account_name = '<your account name>'
account_key = '<your account key>'
container_name = '<your container name>'
blob_name = '<your blob name>'
blob_service = BaseBlobService(
account_name=account_name,
account_key=account_key
)
示例 1. 使用 sas 令牌生成 blob url 以通过以下方式获取内容 requests
from azure.storage.blob import BlobPermissions
from datetime import datetime, timedelta
import requests
sas_token = blob_service.generate_blob_shared_access_signature(container_name, blob_name, permission=BlobPermissions.READ, expiry=datetime.utcnow() + timedelta(hours=1))
print(sas_token)
url_with_sas = blob_service.make_blob_url(container_name, blob_name, sas_token=sas_token)
print(url_with_sas)
r = requests.get(url_with_sas)
dat = np.frombuffer(r.content)
print('from requests', dat)
示例 2. 通过以下方式将 blob 的内容下载到内存中 BytesIO
import io
stream = io.BytesIO()
blob_service.get_blob_to_stream(container_name, blob_name, stream)
dat = np.frombuffer(stream.getbuffer())
print('from BytesIO', dat)
示例 3. 使用numpy.fromfilewithDataSource打开带有 sas 令牌的 blob url,它实际上会将 blob 文件下载到本地文件系统中。
ds = np.DataSource()
# ds = np.DataSource(None) # use with temporary file
# ds = np.DataSource(path) # use with path like `data/`
f = ds.open(url_with_sas)
dat = np.fromfile(f)
print('from DataSource', dat)
我认为示例 1 和 2 更适合您。
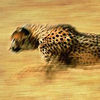
TA贡献1860条经验 获得超9个赞
当涉及到 np.savez 时,上述解决方案通常需要工作。
上传到存储:
import io
import numpy as np
stream = io.BytesIO()
arr1 = np.random.rand(20,4)
arr2 = np.random.rand(20,4)
np.savez(stream, A=arr1, B=arr2)
block_blob_service.create_blob_from_bytes(container,
"my/path.npz",
stream.getvalue())
从存储下载:
from numpy.lib.npyio import NpzFile
stream = io.BytesIO()
block_blob_service.get_blob_to_stream(container, "my/path.npz", stream)
ret = NpzFile(stream, own_fid=True, allow_pickle=True)
print(ret.files)
""" ['A', 'B'] """
print(ret['A'].shape)
""" (20, 4) """
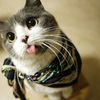
TA贡献1827条经验 获得超7个赞
有点晚了,但如果有人想使用 numpy.load 执行此操作,这里是代码(Azure SDK v12.8.1):
from azure.storage.blob import BlobServiceClient
import io
import numpy as np
# define your connection parameters
connect_str = ''
container_name = ''
blob_name = ''
blob_service_client = BlobServiceClient.from_connection_string(connect_str)
blob_client = blob_service_client.get_blob_client(container=container_name,
blob=blob_name)
# Get StorageStreamDownloader
blob_stream = blob_client.download_blob()
stream = io.BytesIO()
blob_stream.download_to_stream(stream)
stream.seek(0)
# Load form io.BytesIO object
data = np.load(stream, allow_pickle=False)
print(data.shape)
添加回答
举报