1 回答
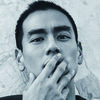
TA贡献1796条经验 获得超7个赞
如果您有圆的中心和半径,您可以通过theta从 中随机选择一个角度[0, 2*pi],通过和计算相应的x和y值,cos(theta)并sin(theta)通过从 中随机选择的一些缩放因子来缩放这些值,从而轻松生成随机坐标[0, radius]。我为你准备了一些代码,见下文。
我省略了你的很多代码(阅读、预处理、保存)以专注于相关部分(参见如何创建一个最小、完整和可验证的示例)。希望您可以自行将我的解决方案的主要思想集成到您的代码中。如果没有,我将提供进一步的解释。
import cv2
import numpy as np
# (Artificial) Background image (instead of reading an actual image...)
bgimg = 128 * np.ones((401, 401, 3), np.uint8)
# Circle parameters (obtained somehow...)
center = (200, 200)
radius = 100
# Draw circle in background image
cv2.circle(bgimg, center, radius, (0, 0, 255), 3)
# Shape of small image (known before-hand...?)
(w, h) = (13, 12)
for k in range(200):
# (Artificial) Small image (instead of reading an actual image...)
smallimg = np.uint8(np.add(128 * np.random.rand(w, h, 3), (127, 127, 127)))
# Select random angle theta from [0, 2*pi]
theta = 2 * np.pi * np.random.rand()
# Select random distance factors from center
factX = (radius - w/2) * np.random.rand()
factY = (radius - h/2) * np.random.rand()
# Calculate random coordinates for small image from angle and distance factors
(x, y) = np.uint16(np.add((np.cos(theta) * factX - w/2, np.sin(theta) * factY - h/2), center))
# Replace (rather than "add") determined area in background image with small image
bgimg[x:x+smallimg.shape[0], y:y+smallimg.shape[1]] = smallimg
cv2.imshow("bgimg", bgimg)
cv2.waitKey(0)
示例输出:
警告:我没有注意,如果小图像可能会违反圆形边界。因此,必须对缩放因子添加一些额外的检查或限制。
编辑:我编辑了上面的代码。考虑到以下评论,我将小图像移动了(width/2, height/2)
,并相应地限制了半径比例因子,以便不违反圆形边界,无论是上/左还是下/右。
之前,有可能在底部/右侧部分 ( n = 200
) 中违反了边界:
编辑后,应防止这种情况 ( n = 20000
):
图像中红线的接触是由于线的粗细。出于“安全原因”,可以再增加 1 个像素的距离。
添加回答
举报