2 回答
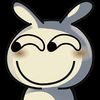
TA贡献1817条经验 获得超14个赞
#ifndef COMPLEX_H
#define COMPLEX_H
#include <iostream>
#include <iomanip>
class Complex
{
public:
Complex(double _real,double _imag = 0.0):real(_real),imag(_imag){} //构造函数,初始化列表和默认参数
Complex(std::istream &is){is >> *this;}; //输入构造函数,调用自身的>>操作符
void SetReal(double _real); //更改实部的值
void SetImag(double _imag); //更改虚部的值
void SetVal(double _real,double _imag); //更改整个复数
inline double GetReal() const; //获取实部,常成员函数
inline double GetImag() const; //获取虚部,常成员函数
Complex& operator+=(const Complex &val); //成员操作符+=
Complex& operator*=(const Complex &val); //成员操作符-=
friend bool operator==(const Complex &lhs,const Complex &rhs); //友元函数,需访问私有数据
friend std::istream& operator>>(std::istream &,Complex &); //友元输入操作符,需私有数据
friend std::ostream& operator<<(std::ostream &,const Complex &); //友元输出操作符,需私有数据
private:
double real;
double imag;
};
Complex operator+(const Complex &lhs, const Complex &rhs); //普通函数,实现两个复数+操作
Complex operator*(const Complex &lhs, const Complex &rhs); //普通函数,实现两个复数*操作
//========================分割线,此线上面为定义代码,此线下面是实现代码===============================
inline bool operator==(const Complex &lhs,const Complex &rhs)
{
return (lhs.real == rhs.real) && (lhs.imag == rhs.imag);
}
inline bool operator!=(const Complex &lhs,const Complex &rhs)
{
return !(lhs==rhs);
}
inline Complex& Complex::operator+=(const Complex &val)
{
real += val.real;
imag += val.imag;
return *this;
}
inline Complex operator+(const Complex &lhs,const Complex &rhs)
{
Complex ret(lhs);
ret += rhs;
return ret;
}
inline Complex& Complex::operator*=(const Complex &val)
{
double tReal = real;
double tImag = imag;
real = tReal*val.real - tImag*val.imag;
imag = tReal*val.imag + tImag*val.real;
return *this;
}
inline Complex operator*(const Complex &lhs,const Complex &rhs)
{
Complex ret(lhs);
ret *= rhs;
return ret;
}
inline std::istream& operator>>(std::istream &is,Complex &com)
{
std::cout << "请输入实数部分:" ;
is >> com.real;
if(is)
{
std::cout << "请输入虚数部分:" ;
is >> com.imag;
if(is)
{
return is;
}
else
{
com = Complex(0.0);
}
}
else
{
com = Complex(0.0);
}
return is;
}
inline std::ostream& operator<<(std::ostream &os, const Complex &com)
{
os << "复数为:" << com.real << std::showpos << com.imag << "i" << std::endl;
return os;
}
inline double Complex::GetReal() const
{
return real;
}
inline double Complex::GetImag() const
{
return imag;
}
void Complex::SetReal(double _real)
{
real = _real;
}
void Complex::SetImag(double _imag)
{
imag = _imag;
}
void Complex::SetVal(double _real,double _imag)
{
real = _real;
imag = _imag;
}
#endif
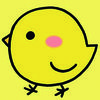
TA贡献1911条经验 获得超7个赞
只实现了要求的基本加减乘除供参考
#include <iostream> using namespace std; class complex { public : complex( double r = 0, double i = 0):real(r), imaginary(i){} complex operator+(complex &a) { complex t(* this ); t.real += a.real; t.imaginary += a.imaginary; return t; } complex operator-(complex &a) { complex t(* this ); t.real -= a.real; t.imaginary -= a.imaginary; return t; } complex operator*(complex &a) { complex t; t.real = a.real*real - a.imaginary*imaginary; t.imaginary = a.real*imaginary + a.imaginary*real; return t; } complex operator/(complex &a) { complex t, t1(a); double div ; t1.imaginary*= -1; div = a.real*a.real - a.imaginary * a.imaginary; t = a * * this ; t.real /= div ; t.imaginary /= div ; return t; } friend ostream &operator << (ostream & out, const complex & a); private : double real, imaginary; }; ostream &operator << (ostream & out, const complex & a) { out << '(' << a.real; if (a.imaginary>0) out<< '+' ; out << a.imaginary << "i)" ; return out; } int main() { complex a(1,2), b(3,4); cout << a << '+' << b << '=' << a+b<< endl; cout << a << '-' << b << '=' << a-b<< endl; cout << a << '*' << b << '=' << a*b<< endl; cout << a << '/' << b << '=' << a/b<< endl; } |
- 2 回答
- 0 关注
- 212 浏览
添加回答
举报