2 回答
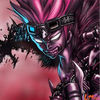
TA贡献1794条经验 获得超8个赞
是的,@Inject可以作为@Autowired......给定一些条件。
Guice 不需要Modules,尽管它们经常使用。因此,如果您愿意,您可以摆脱它们。
如果您的类是具体类,您可以直接使用@Inject它,就像@Autowired,但您可能还必须标记该类,@Singleton因为 Guice 中的默认范围不是单例,而是每个人的新实例,与 Spring 不同。
Guice 不是 Spring,但其中一个最重要的特性存在于另一个中。
在 Tomcat 中使用 Guice
当你想用 Tomcat 使用 Guice 时,需要做的配置很少,但仍然有配置。您将需要一个Module,但只需要servlet。
在您的 中web.xml,添加以下内容:
<listener>
<listener-class>path.to.MyGuiceServletConfig</listener-class>
</listener>
<filter>
<filter-name>guiceFilter</filter-name>
<filter-class>com.google.inject.servlet.GuiceFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>guiceFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
MyGuiceServletConfig.java
public class MyGuiceServletConfig extends GuiceServletContextListener {
@Override protected Injector getInjector() {
return Guice.createInjector(new ServletModule() {
@Override protected void configureServlets() {
serve("/*").with(MyServlet.class); // Nothing else is needed.
}
});
}
}
MyServlet.java
public class MyServlet extends HttpServlet {
@Inject // Similar to @Autowired
private MyService myService;
@Override public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
resp.getWriter().write(myService.hello("Guice"));
}
}
现在你有一个选择MyService:要么用它制作一个接口,你必须定义和绑定一个实现,要么用它制作一个具体的类。
MyService 作为接口
MyService.java
@ImplementedBy(MyServiceImpl.class) // This says "hey Guice, the default implementation is MyServiceImpl."
public interface MyService {
String hello(String name);
}
MyServiceImpl.java
@Singleton // Use the same default scope as Spring
public class MyServiceImpl implements MyService {
// @Inject dependencies as you wish.
public String hello(String name) { return "Hello, " + name + "!"; }
}
如果您想避免@ImplementedBy,您仍然可以使用上面的模块,并添加bind(MyService.class).to(MyServiceImpl.class).in(Scopes.SINGLETON);或编写相同的提供程序方法Module:
@Provides @Singleton MyService provideMyService() {
return new MyServiceImpl();
}
MyService 作为一个具体的类
MyService.java
@Singleton // Use the same default scope as Spring
public class MyService {
// @Inject dependencies as you wish.
public String hello(String name) { return "Hello, " + name + "!"; }
}
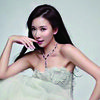
TA贡献1827条经验 获得超4个赞
在 Guice 中,没有@AutowiredSpring 注释的直接等价物。依赖注入的使用在入门页面中有解释。
1)您必须使用注释来注释您的服务的构造函数@Inject:
@Inject
BillingService(CreditCardProcessor processor,
TransactionLog transactionLog) {
this.processor = processor;
this.transactionLog = transactionLog;
}
2)然后在一个模块中定义类型和实现之间的绑定:
public class BillingModule extends AbstractModule {
@Override
protected void configure() {
/*
* This tells Guice that whenever it sees a dependency on a TransactionLog,
* it should satisfy the dependency using a DatabaseTransactionLog.
*/
bind(TransactionLog.class).to(DatabaseTransactionLog.class);
/*
* Similarly, this binding tells Guice that when CreditCardProcessor is used in
* a dependency, that should be satisfied with a PaypalCreditCardProcessor.
*/
bind(CreditCardProcessor.class).to(PaypalCreditCardProcessor.class);
}
}
3)最后构建一个注入器并使用它:
public static void main(String[] args) {
/*
* Guice.createInjector() takes your Modules, and returns a new Injector
* instance. Most applications will call this method exactly once, in their
* main() method.
*/
Injector injector = Guice.createInjector(new BillingModule());
/*
* Now that we've got the injector, we can build objects.
*/
BillingService billingService = injector.getInstance(BillingService.class);
...
}
添加回答
举报