3 回答
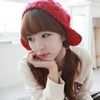
TA贡献1876条经验 获得超7个赞
**您在看这个吗?**请按照@foobar2k19's answer 中的解释进行操作。
function houseParty(input) {
let guestsList = [];
for (let i = 0; i < input.length; i++) {
let nameOfThePerson = input[i].split(" ")[0];
if (input[i].includes('not')) {
if (guestsList.indexOf(nameOfThePerson) > -1) {
guestsList.splice(guestsList.indexOf(nameOfThePerson), 1);
}
} else if(guestsList.includes(nameOfThePerson)) {
guestsList.push(nameOfThePerson + ' is already in the list!');
} else {
guestsList.push(nameOfThePerson);
}
}
return guestsList;
}
const inputArr = ['Tom is going!',
'Annie is going!',
'Tom is going!',
'Garry is going!',
'Jerry is going!'];
console.log(houseParty(inputArr));
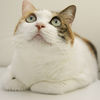
TA贡献1921条经验 获得超9个赞
首先尝试使用 Array#prototype#reduce 构建频率列表,然后将其映射到您想要的响应。
function houseParty(input) {
const res = Object.entries(input.reduce((acc, curr) => {
const name = curr.split(' ')[0];
if (!acc[name]) {
acc[name] = 1;
} else {
acc[name] += 1;
}
return acc;
}, {}))
.map(x => {
if (x[1] > 1) {
return `${x[0]} is already in the list`;
} else {
return x[0];
}
});
return res;
}
const result = houseParty(['Allie is going!',
'George is going!',
'John is not going!',
'George is not going!'
]);
console.log(result);
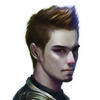
TA贡献1777条经验 获得超3个赞
我会给你更多'容易理解'的方式。
注 1:
最好检查字符串中的“not going”匹配,因为名称可能包含“not”——世界上有很多奇怪的名字(例如 Knott)。
笔记2:
如果他/她的名字在不同状态的输入中重复,您必须从列表中删除他/她。
function houseParty(input) {
let guestList = [];
let notComing = [];
let name, going;
//loop through input
for(let i = 0; i < input.length; i++) {
//get persons name
name = input[i].split(' ')[0];
//set going status
going = !input[i].includes('not going');
if (going) {
//check name in going list
if (guestList.indexOf(name) > -1) {
//this person is already in list
console.log(`${name} is in the list`);
}
else {
//add person in list
guestList.push(name);
}
//check if person was in not going list, remove from it
if (notComing.indexOf(name) > -1) {
//remove from not going list
notComing.splice(notComing.indexOf(name), 1);
}
}
else {
//check if name is in not going list
if (notComing.indexOf(name) > -1) {
console.log(`${name} is in the list`);
}
else {
notComing.push(name);
}
//check if person was in going list before
if (guestList.indexOf(name) > -1) {
guestList.splice(guestList.indexOf(name), 1);
}
}
}
//you have both lists filled now
console.log("Guests: ", guestList);
console.log("Not coming: ", notComing);
}
let input = [
'Allie is going!',
'George is going!',
'John is not going!',
'George is going!',
'George is not going!',
'Knott is going!'
];
//test
houseParty(input);
添加回答
举报